The WordPress Dashboard Widgets API lets themes and plugins add, remove or re-position WordPress dashboard widgets. The WordPress Dashboard is the first thing we see when we log in to the WordPress administration screen. The WordPress Dashboard Widget API has been available from WordPress 2.7 onwards. In this tutorial we’ll look at the different actions and functions that are provided by the API. And, how to add, remove and position dashboard widgets using this API.
Behind the Scene of WordPress Dashboard Widgets
Internally, WordPress dashboard widgets are WordPress meta boxes. The Dashboard Widget API uses the WordPress meta box API to add, remove and position dashboard widgets.Adding a Dashboard Widget
We can add a widget to the WordPress dashboard using thewp_add_dashboard_widget
function. We need to wrap this function inside the wp_dashboard_setup
hook.
Here’s some example code on how to use wp_add_dashboard_widget
:
function add_dashboard_widget()
{
wp_add_dashboard_widget("sitepoint", "SitePoint Videos", "display_sitepoint_dashboard_widget");
}
function display_sitepoint_dashboard_widget()
{
echo "Watch Video Courses at <a href='http://learnable.com'>Learnable</a>";
}
add_action("wp_dashboard_setup", "add_dashboard_widget");
Here, we are calling wp_add_dashboard_widget
inside add_dashboard_widget
, which is triggered by the wp_dashboard_setup
hook.
wp_add_dashboard_widget
takes three arguments. Here are the arguments:
- $id: Every dashboard widget must have a unique id. Make sure you prefix it with your plugin or theme name to avoid overriding.
- $title: Title of the widget to be displayed.
- $callback: A callback which displays the content of the widget.
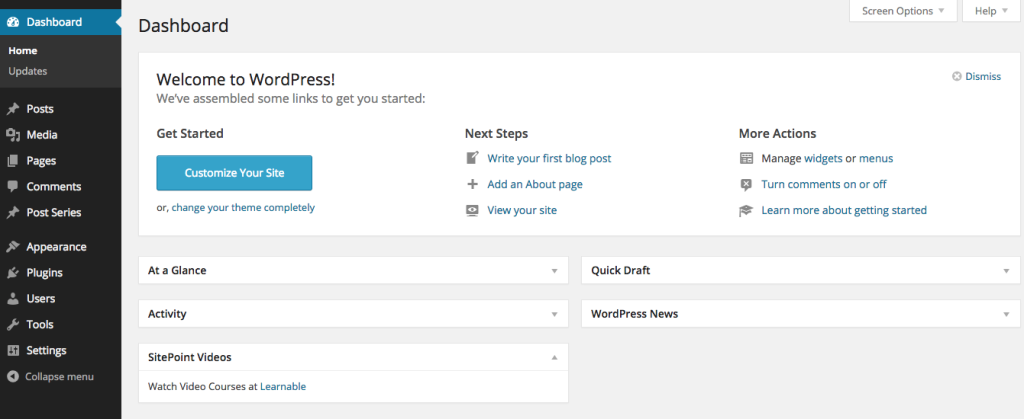
Removing a Dashboard Widget
We can remove a default or custom dashboard widget using theremove_meta_box
function. We need wrap this function in the wp_dashboard_setup
function.
Here’s how we’d use remove_meta_box
:
function remove_dashboard_widget()
{
remove_meta_box("sitepoint", "dashboard", "normal");
}
add_action("wp_dashboard_setup", "remove_dashboard_widget");
Here, we’re calling the remove_meta_box
function inside the remove_dashboard_widget
function, which is indeed triggered by the wp_dashboard_setup
action.
remove_meta_box
takes three arguments, they are:
- $id: The unique id of the widget. Here’s the list of the id’s of the default widgets: dashboard_activity, dashboard_right_now, dashboard_recent_comments, dashboard_recent_drafts, dashboard_primary, dashboard_quick_press, dashboard_secondary, dashboard_primary and dashboard_incoming_links.
- $location: This will always have the value “dashboard” while removing a dashboard.
- $position: If you’re removing a widget which was created by WordPress core by default, or created using
wp_add_dashboard_widget
then its value is “normal”.
Positioning Widgets in the Dashboard
By default, the new widgets are added to bottom left of the dashboard page. The WordPress Dashboard Widgets API doesn’t provide a way to change the position of the widgets. However, we can hack the WordPress core$wp_meta_box
variable as a workaround.
Here is an example on how we can move our widget to the top:
function add_dashboard_widget()
{
wp_add_dashboard_widget("sitepoint", "SitePoint Videos", "display_sitepoint_dashboard_widget");
global $wp_meta_boxes;
// Get reference to the list of dashboard widgets
$dashboard_widgets = $wp_meta_boxes["dashboard"]["normal"]["core"];
//Create a backup of the dashboard widgets list
$dashboard_widgets_backup = array("sitepoint" => $dashboard_widgets["example_dashboard_widget"] );
//remove our widget from the end
unset($dashboard_widgets["example_dashboard_widget"]);
//Merge the backedup and original arrays
$sorted_dashboard = array_merge($dashboard_widgets_backup, $dashboard_widgets);
//save the new sorted widgets array
$wp_meta_boxes["dashboard"]["normal"]["core"] = $sorted_dashboard;
}
function display_sitepoint_dashboard_widget()
{
echo "Watch Video Courses at <a href='http://learnable.com'>Learnable</a>";
}
add_action("wp_dashboard_setup", "add_dashboard_widget");
You can alter this array and change the position of the widgets as needed.
Displaying an RSS Feed in a Dashboard Widget
Let’s create a dashboard widget which retrieves SitePoint’s RSS feed, then displays it. We’ll use WordPress’ API to fetch and parse the RSS feed. Here’s the code for our widget:function add_dashboard_widget()
{
wp_add_dashboard_widget("rss-feed", "RSS", "display_rss_dashboard_widget");
}
function display_rss_dashboard_widget()
{
include_once( ABSPATH . WPINC . "/feed.php");
$rss = fetch_feed("https://www.sitepoint.com/feed/");
$maxitems = $rss->get_item_quantity(10);
$rss_items = $rss->get_items(0, $maxitems);
?>
<ul>
<?php
if($maxitems == 0)
{
echo "<li>No items</li>";
}
else
{
foreach($rss_items as $item)
{
?>
<li>
<a href="<?php echo esc_url($item->get_permalink()); ?>">
<?php echo esc_html($item->get_title()); ?>
</a>
</li>
<?php
}
}
?>
</ul>
<?php
}
add_action("wp_dashboard_setup", "add_dashboard_widget");
Here, we are including the file “feed.php”, which exposes all of the APIs needed to work with RSS in WordPress. Then, we use fetch_feed
to download and parse the RSS feed, finally we display 10 RSS items.
Here is what our RSS dashboard widget should look like:
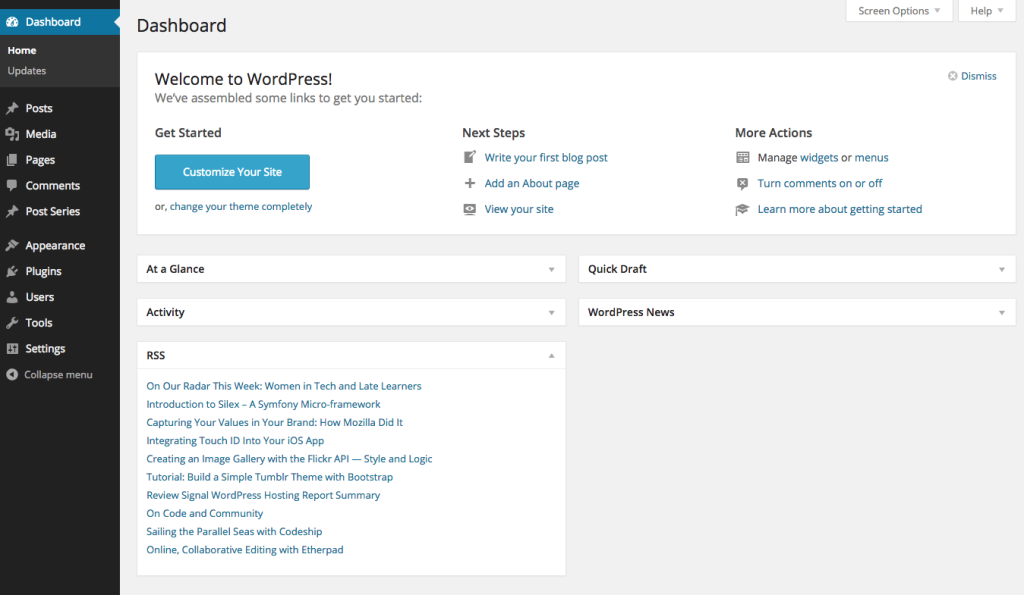
Conclusion
Many WordPress plugins such as analytics, WooCommerce addons and various monitoring extensions use the Dashboard Widgets API. The WordPress Dashboard Widgets API is one of the most important APIs for WordPress developers, in this example we’ve walked you through some basic examples. Please feel free to comment on your experiences with the API below.Frequently Asked Questions about WordPress Dashboard Widgets API
How can I add a new widget to my WordPress dashboard?
Adding a new widget to your WordPress dashboard is a straightforward process. First, you need to create a function that outputs the contents of your widget. Then, you need to register this function with WordPress using the wp_add_dashboard_widget
function. This function takes three parameters: the widget’s ID, its name, and the function that outputs its contents. Once you’ve done this, your widget will appear on your dashboard.
Can I remove existing widgets from my WordPress dashboard?
Yes, you can remove existing widgets from your WordPress dashboard. This can be done using the remove_meta_box
function. This function takes three parameters: the ID of the widget you want to remove, the screen on which the widget is displayed, and the context in which the widget is displayed.
How can I customize the appearance of my dashboard widgets?
The appearance of your dashboard widgets can be customized using CSS. You can add custom CSS to your theme’s style.css file or use a custom CSS plugin. You can target specific widgets by using their ID as the CSS selector.
Can I control the position of my dashboard widgets?
Yes, you can control the position of your dashboard widgets. This can be done using the wp_dashboard_setup
action hook. This hook allows you to specify the position of your widgets on the dashboard.
How can I add custom data to my dashboard widgets?
You can add custom data to your dashboard widgets by creating a function that outputs the data and registering this function with WordPress using the wp_add_dashboard_widget
function. The data can be anything you want, such as statistics, graphs, or even custom forms.
Can I use AJAX in my dashboard widgets?
Yes, you can use AJAX in your dashboard widgets. This can be done by enqueuing the necessary JavaScript files and using the wp_ajax_
action hook to handle the AJAX request on the server side.
How can I make my dashboard widgets interactive?
You can make your dashboard widgets interactive by using JavaScript. You can enqueue your JavaScript files using the wp_enqueue_script
function and then use JavaScript to add interactivity to your widgets.
Can I add multiple instances of the same widget to my dashboard?
No, you cannot add multiple instances of the same widget to your dashboard. Each widget must have a unique ID, so if you want to add multiple instances of the same widget, you will need to register each instance as a separate widget with a unique ID.
Can I add dashboard widgets to other screens in WordPress?
Yes, you can add dashboard widgets to other screens in WordPress. This can be done using the add_meta_box
function. This function allows you to add widgets to any screen in WordPress, not just the dashboard.
How can I make my dashboard widgets responsive?
You can make your dashboard widgets responsive by using CSS media queries. You can add these media queries to your theme’s style.css file or use a custom CSS plugin. You can target specific widgets by using their ID as the CSS selector.

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.