WordPress users who aren’t necessarily developers can’t always customize their website the way they want. They can choose a theme from thousands that are available (both free and paid), but there’s often frustration that they can’t easily change the logo. Some themes provide options allowing their users to change the logo but it’s not always the case.
To allow all users to change the logo of their website easily, the WordPress team decided to add a new feature in WordPress 4.5: Custom Logo. Using this feature, any WordPress user will now be able to change their logo via the Theme Customizer.
If you’re a developer, you’ll want to know how to make use of the Custom Logo feature in your theme. The good news is that it’s not complicated, so you won’t have any excuse to not allow your user to customize their logo! In this article, we’ll look at how a user can change their logo with the Custom Logo feature. Then, we’ll cover how developers can enable the Custom Logo feature using the Custom Logo API.
Customizing a Logo
From a user’s perspective, when the Custom Logo feature is enabled, changing the logo of a website is pretty easy. That was the aim of the feature after all.
The first thing to do is open the Theme Customizer. To do that, you can use the ‘Customize’ entry in the ‘Appearance’ menu in the WordPress dashboard. If you can’t see that entry, that means you don’t have the admin rights to the site to customize the theme (or you are using a very old version of WordPress and you should update it as soon as possible!). You can also access the Customizer thanks to the ‘Customize’ link in the admin top bar on any page of your website.
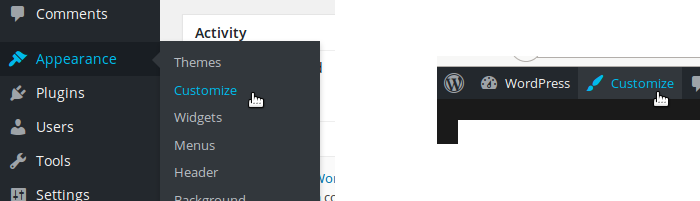
In the Customizer, go to the ‘Site Identity’ panel and you’ll find the ‘Logo’ section. If it’s the first time you’ve changed the logo, you’ll find a ‘Select logo’ button allowing you to select the logo you want to display on your website.
Hitting this button will open a media window. You can choose any existing image or you can upload a new one. Submit your choice with the ‘Choose logo’ button in the bottom right corner.
Once a logo is chosen, the ‘Logo’ section displays it, above two buttons: one to remove the custom logo, and another to change it.
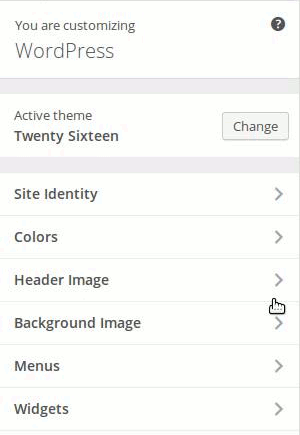
The advantage of using the Theme Customizer is that you directly see the changes. In fact, right after choosing your logo you’ll see it on your website. You’re the only one who can see these changes, until you save them with the ‘Save & Publish’ button at the top of the Customizer.
Using the Custom Logo API
You can test this feature with WordPress’ default themes, because they allow the use of Custom Logo. If you use another theme, you need this theme to explicitly enable the Custom Logo feature. We’ll now highlight how this can be achieved.
Enabling the Custom Logo Feature
To enable the Custom Logo feature, we actually need to declare support for it. There’s a good reason why this feature is not enabled by default. As we’ll see below, we have to use a specific function to display the Custom Logo. If we don’t use this function, the Custom Logo is not displayed, so it’s useless changing it.
To declare support for the Custom Logo feature we use the add_theme_support()
function. The best way to do this is by using a callback function and the after_setup_theme
action. Put the following code in the functions.php
file of your theme to declare the support for the Custom Logo feature.
function mytheme_setup() {
add_theme_support('custom-logo');
}
add_action('after_setup_theme', 'mytheme_setup');
Now your users will be able to select a logo. The problem is that it’s not displayed yet. Before we look at how we display the custom logo, I’ll now walk through another useful parameter.
Choosing the Size of the Logo
A logo is part of the identity of a website. However, that doesn’t mean that as the theme’s developer, you can’t choose its size. I think it can be fairly important to do this, as a logo that is too big can break the entire theme design, which isn’t what we want.
If you want to control the size of the Custom Logo, you first need to declare the size with add_image_size()
which accepts three parameters: the name you want for this size, the width and the height. Then you indicate this size (with the name you chose) as a parameter in the Custom Logo support declaration.
add_image_size('mytheme-logo', 160, 90);
add_theme_support('custom-logo', array(
'size' => 'mytheme-logo'
));
If the user chooses a smaller logo, then the logo won’t be resized. However, if an image’s dimensions are bigger than what is specified, whether that’s in height and/or width, then then it will be resized. In all cases, WordPress will preserve the original ratio when the image is resized.
So, the Custom Logo won’t be taller or wider than the area provided, but it can be smaller.
Displaying the Custom Logo
If you declare support for the Custom Logo feature, you’ll need to display the user’s selection. To do so, you can use the function the_custom_logo()
anywhere you want to see the logo. It will automatically echo the right HTML code to display the logo: an image in the right size, encapsulated into a link redirecting to the homepage.
If, for any reason you want to retrieve this code instead of just echoing it, you can also use get_custom_logo()
. Note that, under the hood, the_custom_logo()
calls get_custom_logo()
to display the user’s logo.
Finally, a third function can help you in your theme: has_custom_logo()
. As its name suggests, this function returns a boolean: true
if a Custom Logo has been set, false
otherwise.
In the following code we display the Custom Logo, if any. We detect the existence of a Custom Logo with has_custom_logo()
and, if there’s no logo to show, we only display the site’s name.
<?php
// Display the Custom Logo
the_custom_logo();
// No Custom Logo, just display the site's name
if (!has_custom_logo()) {
?>
<h1><?php bloginfo('name'); ?></h1>
<?php
}
?>
Note that we don’t use has_custom_logo()
before using the_custom_logo()
. In fact, if there’s no logo to show, then the_custom_logo()
will display an empty string so it’s useless to check the existence of a logo.
Below is another way to do the same thing. We define a function (in the functions.php
file for instance) that displays the Custom Logo or only the site’s name. This time, we use function_exists()
to ensure our theme remains compatible with older versions of WordPress.
function mytheme_custom_logo() {
// Try to retrieve the Custom Logo
$output = '';
if (function_exists('get_custom_logo'))
$output = get_custom_logo();
// Nothing in the output: Custom Logo is not supported, or there is no selected logo
// In both cases we display the site's name
if (empty($output))
$output = '<h1><a href="' . esc_url(home_url('/')) . '">' . get_bloginfo('name') . '</a></h1>';
echo $output;
}
Then we can call this function anywhere we want, for example in the header.
In Summary
As new versions of WordPress are released, the Theme Customizer becomes more and more useful for end users. Users can now personalize their website without needing to change the code of the theme.
Thanks to the new Custom Logo API, and the Site Icon API we covered in a previous article, the end user can now more easily control the identity of their website, without needing custom development.
Frequently Asked Questions about WordPress Custom Logo API
How Can I Change the Size of My Custom Logo in WordPress?
To change the size of your custom logo in WordPress, you need to add a few lines of code to your theme’s functions.php file. This code will define a new image size for your logo. Here’s an example:add_theme_support( 'custom-logo', array(
'height' => 100,
'width' => 400,
'flex-height' => true,
'flex-width' => true,
));
In this example, the height is set to 100px and the width is set to 400px. The ‘flex-height’ and ‘flex-width’ parameters allow the logo to be resized to maintain its aspect ratio.
How Can I Add a Custom Logo to My WordPress Theme?
To add a custom logo to your WordPress theme, you need to add support for it in your theme’s functions.php file. Here’s an example of how to do this:function theme_prefix_setup() {
add_theme_support( 'custom-logo' );
}
add_action( 'after_setup_theme', 'theme_prefix_setup' );
After adding this code, you can go to the Customizer in your WordPress dashboard and you will see an option to add a logo under the ‘Site Identity’ section.
How Can I Display the Custom Logo on My Website?
To display the custom logo on your website, you can use the the_custom_logo()
function in your theme’s template files where you want the logo to appear. Here’s an example:the_custom_logo();
This function will output the HTML necessary to display the logo.
How Can I Retrieve the URL of the Custom Logo?
To retrieve the URL of the custom logo, you can use the get_custom_logo()
function. This function returns the HTML for the custom logo, but you can extract the URL from it using the wp_get_attachment_image_src()
function. Here’s an example:$custom_logo_id = get_theme_mod( 'custom_logo' );
$image = wp_get_attachment_image_src( $custom_logo_id , 'full' );
echo $image[0];
This code will output the URL of the custom logo.
How Can I Change the Link of the Custom Logo?
By default, the custom logo in WordPress links to the home page of the website. If you want to change this, you can use a filter to modify the output of the get_custom_logo()
function. Here’s an example:function change_logo_url( $html ) {
$html = preg_replace( '/(href=["\'])(.*?)(["\'])/', '$1' . 'http://new-url.com' . '$3', $html );
return $html;
}
add_filter( 'get_custom_logo', 'change_logo_url' );
This code will change the link of the custom logo to ‘http://new-url.com‘.
How Can I Add a Custom Class to the Custom Logo?
To add a custom class to the custom logo, you can use a filter to modify the output of the get_custom_logo()
function. Here’s an example:function add_logo_class( $html ) {
$html = str_replace( 'custom-logo-link', 'custom-logo-link your-custom-class', $html );
return $html;
}
add_filter( 'get_custom_logo', 'add_logo_class' );
This code will add the ‘your-custom-class’ class to the custom logo link.
How Can I Remove the Custom Logo from My WordPress Theme?
To remove the custom logo from your WordPress theme, you can simply remove the add_theme_support( 'custom-logo' )
line from your theme’s functions.php file. Alternatively, you can remove the logo from the Customizer in your WordPress dashboard.
How Can I Add Multiple Custom Logos to My WordPress Theme?
WordPress only supports one custom logo by default. However, you can add multiple logos by registering additional image sizes in your theme’s functions.php file and then using the add_image_size()
function to create additional logos. You can then use the wp_get_attachment_image()
function to display these logos in your theme’s template files.
How Can I Add a Fallback Image for the Custom Logo?
To add a fallback image for the custom logo, you can use a conditional statement in your theme’s template files. Here’s an example:if ( has_custom_logo() ) {
the_custom_logo();
} else {
echo '<img src="' . get_template_directory_uri() . '/images/fallback-logo.png" alt="Logo">';
}
This code will display the custom logo if it exists, otherwise it will display a fallback image.
How Can I Add a Custom Logo to the WordPress Login Page?
To add a custom logo to the WordPress login page, you can use the login_enqueue_scripts
action hook to add custom CSS to the login page. Here’s an example:function custom_login_logo() {
echo '<style type="text/css">
#login h1 a, .login h1 a {
background-image: url(' . get_stylesheet_directory_uri() . '/images/site-login-logo.png);
height:65px;
width:320px;
background-size: 320px 65px;
background-repeat: no-repeat;
padding-bottom: 30px;
}
</style>';
}
add_action( 'login_enqueue_scripts', 'custom_login_logo' );
This code will replace the default WordPress logo on the login page with your custom logo.
Currently a math student, Jérémy is a passionate guy who is interested in many fields, particularly in the high tech world for which he covers the news everyday on some blogs, and web development which takes much of his free time. He loves learning new things and sharing his knowledge with others.