I decided to test the performance of DOM caching in a basic JavaScript namespacing pattern which I use quite a lot when coding on a day to day basis. Update 10/04/2013: I’ve added some basic logic into the loops. Updated jsfiddle, Updated jsperf.
You could get a 76% increase in speed performance (based on operations per second) when you use DOM caching.Here is the full results of a jsperf performance test – grouped in pairs. So compare 1 with 2, compare 3 with 4 and so on…
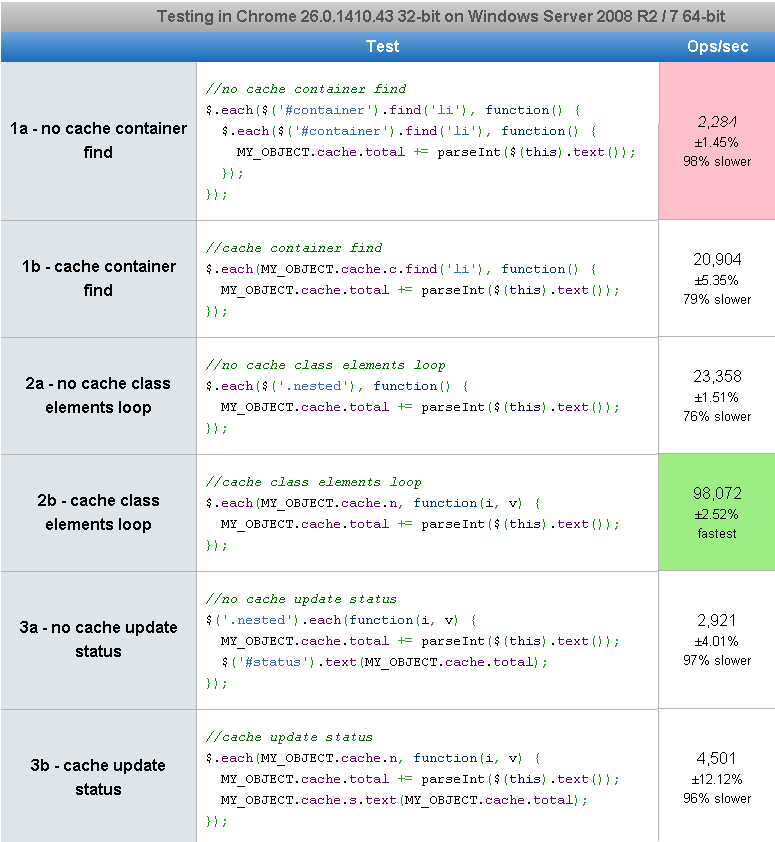
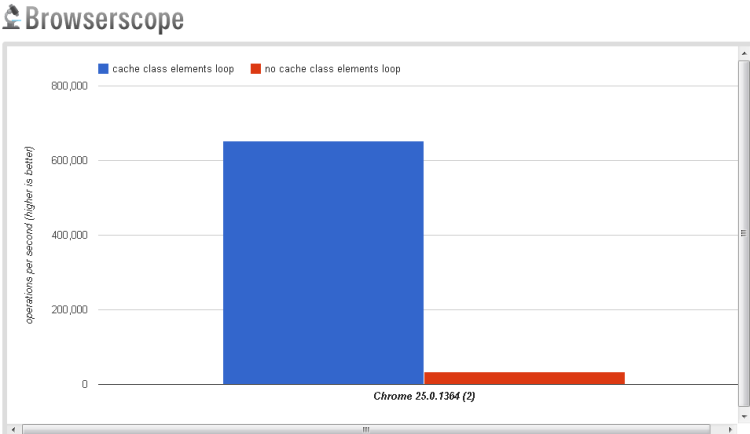
list
nested 1
nested 2
nested 3
list
list
list
A basic js object with some caching.
MY_OBJECT =
{
cache: {},
init: function()
{
this.cache.c = $('#container');
this.cache.n = this.cache.c.find('.nested');
this.cache.s = this.cache.c.find('#status');
}
}
MY_OBJECT.init();
Some standard tests.
//no cache container find
$.each($('#container').find('li'), function(i,v)
{
//...
});
//cache container find
$.each(MY_OBJECT.cache.c.find('li'), function(i,v)
{
//...
});
//no cache class elements loop
$.each($('.nested'), function(i,v)
{
//...
});
//cache class elements loop
$.each(MY_OBJECT.cache.n, function(i,v)
{
//...
});
//no cache update status
$('.nested').each(function(i,v)
{
$('#status').text($(this).text());
});
//cache update status
$.each(MY_OBJECT.cache.n, function(i,v)
{
MY_OBJECT.cache.s.text($(this).text());
});
Frequently Asked Questions about jQuery Performance and DOM Caching
What is DOM Caching and why is it important in jQuery?
DOM Caching, or Document Object Model Caching, is a technique used in jQuery to improve the performance of web applications. It involves storing the results of expensive or frequently used operations for later use, reducing the need for repeated operations and thus improving the speed and efficiency of the application. This is particularly important in jQuery, a JavaScript library, where performance can be significantly impacted by repeated DOM manipulations. By caching the results of these operations, we can reduce the load on the browser and improve the user experience.
How can I implement DOM Caching in my jQuery code?
Implementing DOM Caching in jQuery is relatively straightforward. The most common method is to store the results of a jQuery selector in a variable, which can then be reused later in the code. For example, instead of repeatedly calling $('#myElement')
to select an element with the ID of ‘myElement’, you could store this in a variable like so: var myElement = $('#myElement');
. This variable can then be used in place of the selector, reducing the need for repeated DOM lookups.
What are the potential pitfalls of DOM Caching?
While DOM Caching can greatly improve the performance of your jQuery code, it’s important to be aware of potential pitfalls. One of the main issues is stale cache, where the cached data becomes out of sync with the actual DOM. This can occur if the DOM is modified after the cache is created, leading to inconsistencies between the cached data and the actual state of the DOM. To avoid this, it’s important to update the cache whenever the DOM is modified.
How does DOM Caching compare to other performance optimization techniques in jQuery?
DOM Caching is one of many performance optimization techniques available in jQuery. Other techniques include minimizing DOM manipulations, using efficient selectors, and reducing the use of expensive operations like .html()
. While each of these techniques can improve performance, DOM Caching is particularly effective as it reduces the need for repeated operations, which can be a major source of performance issues in jQuery.
Can I use DOM Caching with other JavaScript libraries or frameworks?
Yes, DOM Caching is not exclusive to jQuery and can be used with any JavaScript library or framework that interacts with the DOM. The principle is the same: store the results of expensive or frequently used operations for later use. However, the implementation may vary depending on the specific library or framework.
How can I ensure that my cache is always up-to-date?
Ensuring that your cache is always up-to-date requires careful management of your code. Whenever the DOM is modified, the cache should be updated to reflect these changes. This can be done manually by updating the cache whenever the DOM is modified, or automatically using event listeners to detect changes to the DOM and update the cache accordingly.
What is the impact of DOM Caching on memory usage?
While DOM Caching can improve performance by reducing the need for repeated operations, it can also increase memory usage as the cached data needs to be stored somewhere. However, this increase in memory usage is typically small compared to the performance benefits, and is usually not a concern unless you’re dealing with very large amounts of data.
Can I use DOM Caching in conjunction with server-side caching?
Yes, DOM Caching can be used in conjunction with server-side caching to further improve performance. Server-side caching involves storing the results of expensive operations on the server, reducing the need for these operations to be performed on each request. By combining this with DOM Caching, you can reduce both the server load and the client-side processing, leading to a faster and more responsive application.
How can I measure the performance impact of DOM Caching?
There are several tools available to measure the performance impact of DOM Caching. One of the most common is the browser’s built-in developer tools, which can provide detailed information about the time taken for each operation. By comparing the performance with and without DOM Caching, you can get a clear idea of the impact on performance.
Are there any alternatives to DOM Caching for improving jQuery performance?
Yes, there are several alternatives to DOM Caching for improving jQuery performance. These include minimizing DOM manipulations, using efficient selectors, reducing the use of expensive operations, and using server-side caching. Each of these techniques can improve performance, and often the best results are achieved by combining several techniques.
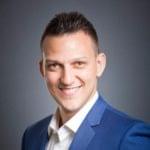
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.