jQuery function to get coordinates of element.
The jQuery methods
.position() method allows us to retrieve the current position of an element relative to the offset parentvar pos = $('#wrapper').position();
console.dir(pos);
//output: left: 0, top: 20
.offset(), which retrieves the current position relative to the document.
var offset = $('#wrapper').offset();
console.dir(offset);
//output: left: 70, top: 40
Into coordinates:
var elem = $("#wrapper");
var x = $("#wrapper").offset().left;
var y = $("#wrapper").offset().top;
console.log('x: ' + x + ' y: ' + y);
//output: x: 70 y: 40
jQuery getCoord() function
jQuery.fn.getCoord = function()
{
var elem = $(this);
var x = elem.offset().left;
var y = elem.offset().top;
console.log('x: ' + x + ' y: ' + y);
//output: x: 70 y: 40
return {
"x" : x,
"y" : y
};
//note that it is not efficient as it breaks the jQuery chain
//return elem;
};
$('#wrapper').getCoord();
//output: Object { x=70, y=40 }
Frequently Asked Questions (FAQs) about jQuery Coordinates and Elements
How can I get the position of an element relative to the document using jQuery?
To get the position of an element relative to the document, you can use the jQuery .offset()
method. This method returns an object containing the properties top
and left
, which represent the element’s position in pixels relative to the document. Here’s an example:var position = $("#element").offset();
console.log("The element is located at " + position.left + ", " + position.top);
In this code, #element
is the ID of the element you want to find the position of. The console.log
statement will print the element’s position to the console.
What is the difference between .position()
and .offset()
in jQuery?
The .position()
and .offset()
methods in jQuery both return the position of an element, but they calculate this position differently. The .position()
method returns the position of an element relative to its offset parent. An offset parent is the closest ancestor (parent, grandparent, etc.) that has a position other than static
.
On the other hand, the .offset()
method returns the position of an element relative to the document. This means it calculates the position based on the entire page, not just the element’s immediate parent or ancestor.
How can I set the position of an element using jQuery?
To set the position of an element using jQuery, you can use the .offset()
method in combination with an object that specifies the top
and left
properties. Here’s an example:$("#element").offset({ top: 100, left: 200 });
In this code, #element
is the ID of the element you want to move, and 100
and 200
are the new top and left positions, respectively, in pixels.
How can I find the absolute position of an element using jQuery?
The absolute position of an element can be found using the .offset()
method in jQuery. This method returns an object containing the properties top
and left
, which represent the element’s position in pixels relative to the document. Here’s an example:var position = $("#element").offset();
console.log("The element is located at " + position.left + ", " + position.top);
In this code, #element
is the ID of the element you want to find the position of. The console.log
statement will print the element’s position to the console.
How can I get the position of an element relative to its parent using jQuery?
To get the position of an element relative to its parent, you can use the jQuery .position()
method. This method returns an object containing the properties top
and left
, which represent the element’s position in pixels relative to its offset parent. Here’s an example:var position = $("#element").position();
console.log("The element is located at " + position.left + ", " + position.top);
In this code, #element
is the ID of the element you want to find the position of. The console.log
statement will print the element’s position to the console.
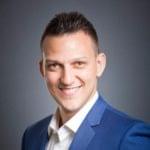
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.