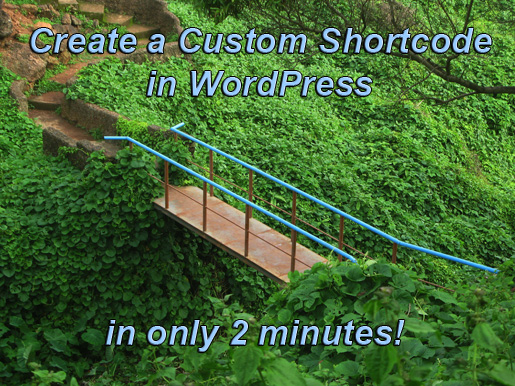
Summary for Future Reference
Because I hope you’ll bookmark this page and reference later, here’s a summary of what it takes to get a shortcode functioning:- Create the code you want
- Save the code (usually in some place like the wp-content folder)
- Include the new file you saved within the functions.php file
- Reference your shortcode using add_shortcode(‘shortcode’, ‘function’)
- Place your shortcode wherever you want it
Why All the Shortcode Love, Exactly?
If you’ve ever had to do any kind of WordPress development, you’ve run into the situation where you need some custom functionality or a specialized design element. For security reasons, WordPress strips a lot of HTML tags and all PHP code out of posts and pages. This is usually for our own good, but it makes for a nightmare when you just want a simple design modification. Shortcodes are the stop-gap. They allow you to inject any PHP or other code right where you want it. Once you’re comfortable creating the shortcode markup, you’ll never go back. So, let’s jump into creating a custom shortcode and build out a process that you can reference in the future when you’re ready to make your own.Step 1: Create the Code
This is where you need to create your functionality. I don’t know what you want to accomplish, but here’s a very simple example of a shortcode that allows me to inject a custom message into a post. This is just for demonstration purpose, but it should get your imagination fired up: [sourcecode language=”php”] <?php function some_random_code(){ echo ‘Thanks for reading my posts!’; }// End some_random_code() ?> [/sourcecode] So we have a very simple little PHP function. You could literally put anything in this function that you want to execute on your site — a custom form, special HTML markup, or calls to an external database.Step 2: Save the Code (But NOT in the Functions.php File)
While you can store the above code within the functions.php file, I would be setting you up for failure long-term if I actually advised it. I strongly recommend that you create a separate file for your own custom shortcodes. Call it whatever you like, and store it in the wp-content folder, or create a plugin (see my article on creating WordPress plugins). For this example, create a file named “my_custom_shortcodes.php” and save it within the wp-content folder. This folder is generally safe from WordPress upgrades and core updates that can overwrite your existing functions.php file. You can lose a lot of work fast if a client decides to update to the latest theme update or install a new theme without telling you. It’s a lot easier to just go in and recreate the next few steps.Step 3: Include Your Custom PHP File
Now we need to tell WordPress where to find your custom shortcode file. Assuming you saved it in your wp-content folder, here’s what you need to add to your functions.php file: [sourcecode language=”php”] include(WP_CONTENT_DIR . ‘/my_custom_shortcodes.php’); [/sourcecode] Now, WordPress is aware of your content and will integrate it into its functionality. We’re 90% done at this point!Step 4: Define Your Shortcode
Before you can actually start using the shortcode throughout your site, you need to tell WordPress that it is, in fact, a real shortcode. Right below your code in Step 3 within your functions.php file, add the following: [sourcecode language=”php”] add_shortcode( ‘some_random_code_sc’, ‘some_random_code’ ); [/sourcecode] Here’s what’s happening — the first part of the add_shortcode call is the name of the shortcode you want to use. This is what will be put in your posts and pages. The next argument is the name of the function. So here’s an example in more plain English: [sourcecode language=”php”] add_shortcode( ‘shortcode_name’, ‘function_name’ ); [/sourcecode] Quick note: I like to use the name of the function for my shortcode, but I add the “_sc” to the shortcode (for ShortCode) to help me track down my code if I want to edit it later. Here’s what my functions.php file looks like after I’ve added my two lines of code: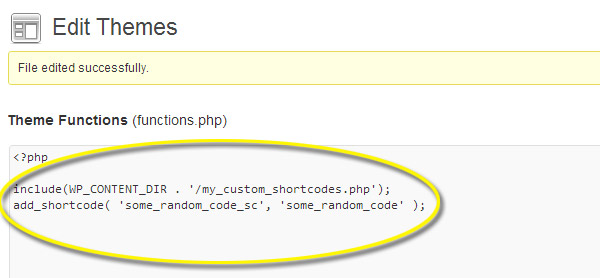
Step 5: Add Your Shortcode
Now, all you need to do is add your shortcode to any post or page! Create a new post/page and add the shortcode to it, publish, and view the page.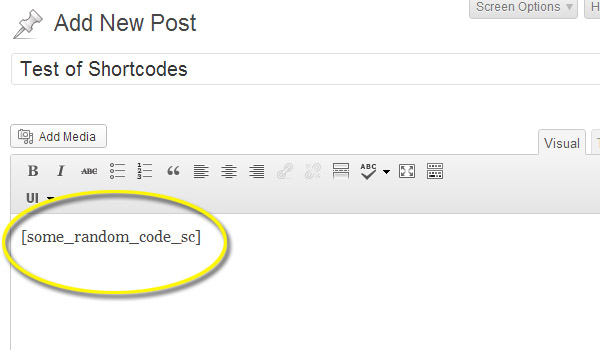
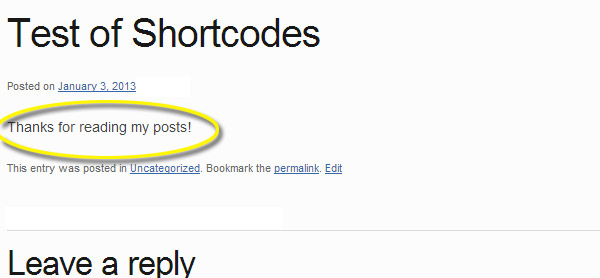
Frequently Asked Questions about Creating Custom Shortcodes for WordPress
What are the benefits of creating custom shortcodes in WordPress?
Custom shortcodes in WordPress offer a range of benefits. They allow you to add functionality to your site without having to write complex code each time. You can reuse the same shortcode across multiple posts or pages, saving you time and effort. They also help in keeping your content clean and organized, as you can replace long pieces of code with a simple shortcode.
Can I use custom shortcodes in widgets?
Yes, you can use custom shortcodes in widgets. By default, WordPress text widgets don’t process shortcodes. However, you can add a simple line of code to your theme’s functions.php file to enable this feature. Just add add_filter(‘widget_text’, ‘do_shortcode’); to your functions.php file.
How can I create a shortcode that accepts parameters?
To create a shortcode that accepts parameters, you need to modify the function you’ve created for your shortcode. The function should accept an array of attributes as its argument. You can then use these attributes within your function to customize the output. For example, if you’re creating a shortcode to display a button, you could accept parameters for the button’s color, size, and text.
Can I nest shortcodes within each other?
Yes, you can nest shortcodes within each other. However, the order in which you place them matters. The inner shortcode must be closed before the outer one. For example, [outer_shortcode][inner_shortcode][/inner_shortcode][/outer_shortcode] is the correct way to nest shortcodes.
How can I debug my custom shortcode if it’s not working?
If your custom shortcode isn’t working, there are a few steps you can take to debug it. First, check your syntax to make sure there are no errors. Second, ensure that you’ve added your shortcode function to your theme’s functions.php file. Finally, use the var_dump() function to output the value of variables and see where things might be going wrong.
Can I use HTML within my shortcode?
Yes, you can use HTML within your shortcode. This allows you to create more complex output with your shortcode. However, remember to return the output of your shortcode function, rather than echoing it. Echoing the output can cause issues with the order in which content is displayed on your page.
How can I create a shortcode that displays dynamic content?
To create a shortcode that displays dynamic content, you need to include PHP code within your shortcode function that retrieves or generates the content you want to display. This could be anything from a recent posts list to a random quote generator.
Can I use shortcodes in my theme files?
Yes, you can use shortcodes in your theme files. To do this, you need to use the do_shortcode() function provided by WordPress. For example, echo do_shortcode(“[your_shortcode]”); would output the result of your shortcode where you place this code in your theme file.
How can I create a shortcode that outputs a form?
To create a shortcode that outputs a form, you need to include HTML for the form within your shortcode function. You can then use PHP to process the form data when it’s submitted. Remember to properly sanitize and validate any data you receive from the user to ensure security.
Can I deactivate or delete a shortcode?
Yes, you can deactivate or delete a shortcode. To deactivate a shortcode, simply comment out the add_shortcode line in your functions.php file. To delete a shortcode, remove both the function for the shortcode and the add_shortcode line from your functions.php file. Remember to check your site for instances of the shortcode and remove them, as they will display as plain text if the shortcode is deactivated or deleted.

Tara Hornor has a degree in English and has found her niche writing about marketing, advertising, branding, graphic design, and desktop publishing. She is a Senior Editor for Creative Content Experts, a company that specializes in guest blogging and building backlinks. In addition to her writing career, Tara also enjoys spending time with her husband and two children.