10 jQuery Plugins to help with Responsive Layouts
Responsive layout in web design is an important topic of discussion at the moment. Basically a responsive layout allows you to offer a specific and optimized screen size based on whatever device (mobile, tablet…) the visitor uses. Typically, you would use Media Queries to resize the overall layout, but navigation, forms, images, sliders, carousels… they all need to be optimized as well. This is why we are giving you our collection of 10 jQuery plugins that will help you optimize and resize those trickier web elements. Have fun!
Related Posts:
- 10 jQuery Layout Tutorials
- 10 jQuery Plugins for your Webpage Customization
- 8 Stunning jQuery Page Layout Plugins
1. The Heads-Up Grid
Built with HTML, CSS & JavaScript. The Heads-Up Grid is a responsive overlay grid for in-browser website development. It has been created to make it relatively easy to adapt to the needs of responsive web design.
2. FitVids.JS
Is a lightweight, easy-to-use jQuery plugin for responsive width video embeds. It automates the Intrinsic Ratio Method by Thierry Koblentz to achieve fluid width videos in your responsive web design.
3. AdaptiveMedia
With AdaptiveMedia, you need only one CSS file for any window sizes, no need LESS or same solutions, no need CSS3 Media Queries. Less than 3KB, HTML5 and IE6 compatible. User callback: execute user function before completed all job.
4. rlightbox
Is a jQuery UI mediabox that can display many types of content such as images, YouTube and Vimeo videos. It has many unique features like Panorama and Live Re-size and like other jQuery UI widgets, it is ThemeRoller ready.
5. Elastislide
Is a responsive jQuery carousel that will adapt its size and its behavior in order to work on any screen size.
6. Responsive Image Gallery with Thumbnail Carousel
Using the Elastislide plugin (above), this technique will show you how to implement a responsive gallery that adapts to the view-port width. The gallery will has a view switch that allows you to view it with the thumbnail carousel or without. It also supports keyboard navigation.
7. Seamless Responsive Photo Grid
Maintains the aspect ratio of your images.
8. Responsive jQuery Slideshow
This example makes it possible to have fluid jQuery slideshow which scales responsively. This is the same method which is used on the new kiskolabs.com.
9. wmuSlider
Please keep in mind that it’s still very raw (really) and under the MIT LICENSE, which basically means: break it, steal it but THE STUFF IS PROVIDED “AS IS”!
10. Doubletake
Intended to be a proof of concept, Doubletake is a plugin that dynamically updates the src of your images based on the browser width. You start with a small, mobile-friendly image in your HTML. Doubletake will then use a defined set of breakpoints to update image SRCs when necessary.
Frequently Asked Questions about jQuery Plugins for Responsive Layouts
What are jQuery plugins for responsive layouts?
jQuery plugins for responsive layouts are tools that help web developers create websites that can adapt to different screen sizes. These plugins are built using jQuery, a popular JavaScript library, and they provide a variety of features that make it easier to design responsive websites. For example, they can automatically resize images, adjust the layout of a page, or change the navigation menu depending on the size of the user’s screen.
How do I use a jQuery plugin for responsive layouts?
To use a jQuery plugin for responsive layouts, you first need to include the jQuery library and the plugin’s JavaScript file in your HTML document. Then, you can use the plugin’s functions in your JavaScript code to control the layout of your website. Each plugin has its own set of functions and options, so you should refer to the plugin’s documentation for specific instructions.
What are some popular jQuery plugins for responsive layouts?
There are many jQuery plugins for responsive layouts available, each with its own set of features. Some popular ones include Responsive Grid System, FlexSlider, and FitText. Responsive Grid System is a plugin that helps you create a flexible grid layout for your website. FlexSlider is a plugin that provides a responsive and customizable slider. FitText is a plugin that automatically adjusts the font size of your text to fit the width of the parent element.
Can I use jQuery plugins for responsive layouts with other JavaScript libraries?
Yes, you can use jQuery plugins for responsive layouts with other JavaScript libraries. However, you should be aware that some libraries may conflict with jQuery, so you may need to use jQuery’s noConflict method to avoid issues. Also, keep in mind that each library you add to your website increases its load time, so you should only use the libraries you really need.
Do I need to know JavaScript to use jQuery plugins for responsive layouts?
While you can use jQuery plugins for responsive layouts without knowing JavaScript, having a basic understanding of JavaScript will make it much easier to use these plugins effectively. jQuery is a JavaScript library, so its syntax and concepts are based on JavaScript. If you’re new to JavaScript, there are many online resources available that can help you learn the basics.
Are jQuery plugins for responsive layouts compatible with all browsers?
Most jQuery plugins for responsive layouts are designed to be compatible with all modern browsers. However, some older browsers may not support certain features of these plugins. You should always test your website in multiple browsers to ensure that it works correctly.
How can I customize a jQuery plugin for responsive layouts?
Most jQuery plugins for responsive layouts provide options that you can use to customize their behavior. These options are usually set when you initialize the plugin in your JavaScript code. Refer to the plugin’s documentation for a list of available options and instructions on how to use them.
Can I use multiple jQuery plugins for responsive layouts on the same page?
Yes, you can use multiple jQuery plugins for responsive layouts on the same page. However, you should be careful to avoid conflicts between plugins. If you’re using multiple plugins, it’s a good idea to initialize them in a specific order and to test your website thoroughly to ensure that everything works correctly.
Do jQuery plugins for responsive layouts affect the performance of my website?
jQuery plugins for responsive layouts can affect the performance of your website, especially if you’re using multiple plugins or if your plugins are not optimized. However, most plugins are designed to be lightweight and efficient, so the impact on performance is usually minimal.
Where can I find more jQuery plugins for responsive layouts?
There are many websites where you can find jQuery plugins for responsive layouts. Some popular ones include the jQuery Plugin Registry, GitHub, and various web development blogs and forums. Always make sure to download plugins from reputable sources to avoid security risks.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
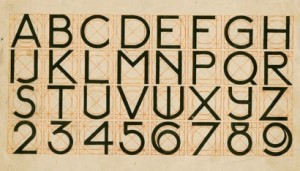
Published in
·Design·Design & UX·Illustration·Photography & Imagery·Resources·Typography·June 16, 2016