Winston is a popular logging library for Node.js, designed to simplify the process of creating, formatting, and transporting log messages. It provides a flexible and modular logging system with various transports and formats, enabling developers to tailor logging to their specific requirements.
In this article, you will learn about fundamental practices and principles of logging and understand why logging is essential in application development. Additionally you will set up Winston in a Node.js project on Vultr Compute server and configure different logging levels.
This is a sponsored article by Vultr. Vultr is the worlds largest privately-held cloud computing platform. A favorite with developers, Vultr has served over 1.5 million customers across 185 countries with flexible, scalable, global Cloud Compute, Cloud GPU, Bare Metal, and Cloud Storage solutions. Learn more about Vultr.
Why Logging is Important
Logging plays a crucial role in application development, providing several benefits:
- Debugging: Logs help developers identify and resolve issues by pinpointing the root cause of errors and exceptions.
- Monitoring: Logs offer valuable insights into application performance, resource usage, and user behavior, enabling proactive problem-solving and system optimization.
- Auditing: Logs serve as a historical record of system events, making it easier to track changes, detect security breaches, and comply with regulatory requirements.
Fundamental Practices and Principles of Logging
Logging should be approached with care and consideration. Here are some best practices and principles to keep in mind:
- Log at the appropriate level: Use different log levels (e.g., error, warn, info, debug) to categorize messages based on their severity and importance.
- Be consistent: Standardize log message formats and include relevant context, such as timestamps, log levels, and error messages.
- Log structured data: Use structured log formats, like JSON, to facilitate parsing, analysis, and integration with monitoring tools.
- Minimize logging overhead: Avoid excessive logging, as it can negatively impact performance and generate noise, making it harder to identify critical issues.
- Secure sensitive data: Be cautious when logging sensitive information, such as passwords, tokens, or personal data, to prevent unauthorized access and protect user privacy.
Winston Basics and Logging Levels
Winston supports multiple logging levels to help you categorize log messages based on their severity:
error
: Critical errors that cause application failure or severe degradation.warn
: Non-critical warnings that indicate potential issues or unexpected behavior.info
: Informational messages that provide context or describe the application’s normal operation.debug
: Detailed debugging information that helps developers understand the internal workings of the application.verbose
: Extremely detailed information, typically used for advanced troubleshooting or performance analysis.silly
: The least severe logging level, often used for logging trivial or insignificant events.
Setting Up Winston in Your Node.js Project
To get started with Winston, follow these steps:
- Deploy a Vultr Compute instance using the Vultr Customer Portal with Node.js marketplace application.
- Securely access the server using SSH as a non-root sudo user.
- Update the server.
- Create a new Node.js project and initialize a
package.json
file:$ mkdir my-winston-project $ cd my-winston-project $ npm init -y
- Install Winston and Express.
$ npm install winston express
- Create a new file named
app.js
.$ nano app.js
- Add the following code.
const express = require("express"); const app = express(); app.get("/", (req, res, next) => { console.log("debug", "Hello, world"); console.log("This is the home route."); res.status(200).send("Logging Hello World.."); }); app.get("/event", (req, res, next) => { try { throw new Error("Not User!"); } catch (error) { console.error("Events Error: Unauthenticated"); res.status(500).send("Error!"); } }); app.listen(3000, () => { console.log("Server Listening On Port 3000"); });
Save and exit the file.
- Allow incoming incoming connections to port
3000
.$ sudo ufw allow 3000
- Run the application.
$ node app.js
Now upon accessing the routes, you will receive the logs in the following format.
debug Hello, world This is the home route.
- Create a new file named
logger.js
.nano logger.js
- Add the following code.
const winston = require("winston"); const logger = winston.createLogger({ level: "debug", format: winston.format.json(), transports: [new winston.transports.Console()], }); module.exports = logger;
Save and exit the file.
- Open the
app.js
file to configure the Winston logger.$ nano app.js
- Edit the existing configuration.
const express = require("express"); const logger = require("./logger"); const app = express(); app.get("/", (req, res, next) => { logger.log("debug", "Hello, world"); logger.debug("This is the home route."); res.status(200).send("Logging Hello World.."); }); app.get("/event", (req, res, next) => { try { throw new Error("Not User!"); } catch (error) { logger.error("Events Error: Unauthenticated"); res.status(500).send("Error!"); } }); app.listen(3000, () => { logger.info("Server Listening On Port 3000"); });
- Run the application.
$ node app.js
Now upon accessing the routes, you will receive the logs in the following JSON format.
{"level":"info","message":"Server Listening On Port 3000"} {"level":"debug","message":"Hello, world"} {"level":"debug","message":"This is the home route."} {"level":"error","message":"unauthenticated user"}
Do More With Vultr
- Containerize Node.js Web Applications
- Implement a CI/CD Pipeline with Jenkins and Vultr Kubernetes Engine
- Run Multiple Node.JS Applications on Ubuntu with Nginx
- Deploy a MERN Application on Ubuntu
Conclusion
Logging is an essential aspect of application development, providing valuable insights into system performance, user behavior, and error resolution. By using Winston, a powerful and flexible logging library for Node.js, you can easily create, format, and transport log messages tailored to your specific needs. With the knowledge gained from this article, you are now equipped to incorporate effective logging practices into your Node.js projects.
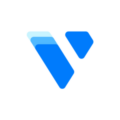
Vultr is the world’s largest privately-held cloud computing platform. A favorite with developers, Vultr has served over 1.5 million customers across 185 countries with flexible, scalable, global Cloud Compute, Cloud GPU, Bare Metal, and Cloud Storage solutions.