Nothing special just some JavaScript code snippet to open a popup window.
W = window,
D = document;
//set the window to widgets dimensions
var winH = 400, //allow for window padding and header
winW = 400,
winT = 400,
winL = 400;
//set the content
var windowName = 'Widget', // should not include space for IE
path = W.location.path,
windowOptions = 'location=0,status=0,toolbar=0,dependent=1,resizable=1,menubar=0,screenX=' + winL + ',screenY=' + winT + ',width=' + winW + ',height=' + winH,
that = this;
//note path needs to be in the same domain...
//open the window
that._Window = window.open(path, windowName, windowOptions);
that._Interval = window.setInterval(function () {
if (that._Window.closed) {
window.clearInterval(that._Interval);
//code to run when window is closed
}
}, 1000);
Frequently Asked Questions about JavaScript Popup Windows
How can I create a popup window using JavaScript?
Creating a popup window in JavaScript is quite simple. You can use the window.open()
method. This method creates a new window and returns a reference to it. Here is a basic example:var myWindow = window.open("", "myWindow", "width=200,height=100");
myWindow.document.write("<p>This is 'myWindow'</p>");
In this example, the window.open()
method takes three parameters: the URL of the page to open (left blank here to create an empty window), the name of the window, and a string indicating the window’s features (like its width and height).
How can I control the size and position of the popup window?
You can control the size and position of the popup window by specifying them in the third parameter of the window.open()
method. For example, to create a window that is 500 pixels wide and 600 pixels tall, and positioned 10 pixels from the left and top of the screen, you would use:var myWindow = window.open("", "myWindow", "width=500,height=600,left=10,top=10");
Can I load a specific URL in the popup window?
Yes, you can load a specific URL in the popup window by specifying it as the first parameter in the window.open()
method. For example:var myWindow = window.open("https://www.example.com", "myWindow", "width=500,height=600");
How can I close a popup window using JavaScript?
You can close a popup window using the window.close()
method. This method closes the current window, or the window on which it was called. Here’s an example:myWindow.close();
Can I prevent the popup window from being resized?
Yes, you can prevent the popup window from being resized by adding the resizable=no
feature in the window.open()
method. For example:var myWindow = window.open("", "myWindow", "width=500,height=600,resizable=no");
How can I check if a popup window is still open?
You can check if a popup window is still open by using the window.closed
property. This property returns a boolean value indicating whether the window is closed (true) or not (false). Here’s an example:if(!myWindow.closed) {
// The window is still open
}
Can I interact with the contents of the popup window?
Yes, you can interact with the contents of the popup window using the document
property of the window object. For example, to write some text in the popup window, you can use:myWindow.document.write("<p>Hello, World!</p>");
Can I open a popup window without the browser’s toolbar?
Yes, you can open a popup window without the browser’s toolbar by adding the toolbar=no
feature in the window.open()
method. For example:var myWindow = window.open("", "myWindow", "width=500,height=600,toolbar=no");
Can I force the popup window to always be on top?
No, JavaScript does not provide a built-in way to force a popup window to always be on top. This is controlled by the user’s operating system and browser settings.
Can I detect when the popup window is closed?
Yes, you can detect when the popup window is closed by using the window.onunload
event. This event is fired when the window is about to unload its resources. Here’s an example:myWindow.onunload = function() {
// The window is about to be closed
};
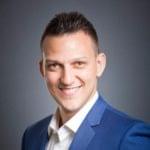
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.