This code uses HTML5 Datalist tag to setup autocomplete options for a text field. It grabs the data from a JSON file with an AJAC request (data which can be stored in the browser persistently if need be or locally on a js object). It then uses the selection of a suburb to autopopulate other fields postcode and state when the suburb changes. Works like a treat in pretty much all browser except Safari.
Notes: HTML Datalist still not compatiable with all browsers. See compatibility. For backfill plugin use this one: jQuery.relevantdropdowns.js – It inserts a UL tag with LI for options in replace of the datalist options.
HTML5 Datalist Tag
Full jQuery
This code populates the datalist via JSON and auto fills other fields based on the field’s auto complete selection by the user.window.DATALIST = {
cache: {},
init: function()
{
var _this = this,
this.cache.$form = $('formid');
this.cache.$suburbs = this.cache.$form.find('datalist#suburbs');
this.cache.$suburbInput = this.cache.$form.find('input[name="suburb"]');
this.cache.$postcodeInput = this.cache.$form.find('input[name="postcode"]');
this.cache.$stateInput = this.cache.$form.find('input[name="state"]');
//grab the datalist options from JSON data
var checkMembershipRequest = $.ajax({
type: "GET",
dataType: "JSON",
url: "/php/suburbs.php"
});
checkMembershipRequest.done(function(data)
{
console.log(data);
//data could be cached in the browser if required for speed.
// localStorage.postcodeData = JSON.stringify(data);
//add options to datalist
$.each(data.suburbs, function(i,v)
{
_this.cache.$suburbs.append(''+i+'');
});
//hook up data handler when suburb is changed to autocomplete postcode and state
_this.cache.$suburbInput.on('change', function()
{
// console.log('suburb changed');
var val = $(this).val(),
selected = _this.cache.$suburbs.find('option[data-value="'+val+'"]'),
postcode = selected.data('postcode'),
state = selected.data('state');
_this.cache.$postcodeInput.val(postcode);
_this.cache.$stateInput.val(state);
});
});
checkMembershipRequest.fail(function(jqXHR, textStatus)
{
console.log( "postcode request fail - an error occurred: (" + textStatus + ")." );
//try again...
});
}
}
Full HTML
This is what your HTML might look like:
*Suburb:
*Postcode:
State:
Full JSON
PHP file returns JSON – could be .json or .php and grab data from a database if required.{
"suburbs": {
"suburb1": {
"postcode": "2016",
"state": "NSW"
},
"suburb2": {
"postcode": "4016",
"state": "QLD"
},
"suburb3": {
"postcode": "3016",
"state": "CA"
},
"suburb4": {
"postcode": "8016",
"state": "WA"
},
"suburb5": {
"postcode": "6016",
"state": "SA"
}
}
}
html5 trigger datalist
Use ALT+down arrow to simulate user action. You’ll need to use jQuery to simulate a multiple trigger keypress. keycode ALT = 18 (also modifier key called altKey) keycode Down Arrow = 40var e = jQuery.Event("keydown");
e.which = 40;
e.altKey = true;
$("input").trigger(e);
Frequently Asked Questions (FAQs) about jQuery AJAX and HTML5 Datalist Autocomplete
How can I dynamically load datalist options with AJAX in Firefox?
To dynamically load datalist options with AJAX in Firefox, you need to use the jQuery AJAX method. First, you need to create an AJAX request to your server-side script. This script should return the data you want to populate in the datalist. Once the AJAX request is successful, you can use the response data to populate the datalist. Here’s a simple example:$.ajax({
url: 'your-server-side-script',
success: function(data) {
var dataList = $('#your-datalist-id');
dataList.empty();
$.each(data, function(index, value) {
dataList.append('<option value="'+value+'">');
});
}
});
In this code, replace ‘your-server-side-script’ with the URL of your server-side script and ‘your-datalist-id’ with the ID of your datalist.
How can I use jQuery autocomplete with callback AJAX JSON?
jQuery UI provides an autocomplete widget that you can use with AJAX and JSON. You need to initialize the autocomplete widget on your input field and provide a source option. The source option should be a function that makes an AJAX request and uses the response data to populate the autocomplete suggestions. Here’s an example:$('#your-input-id').autocomplete({
source: function(request, response) {
$.ajax({
url: 'your-server-side-script',
dataType: 'json',
data: {
term: request.term
},
success: function(data) {
response(data);
}
});
}
});
In this code, replace ‘your-input-id’ with the ID of your input field and ‘your-server-side-script’ with the URL of your server-side script.
How can I use jQuery AJAX and HTML5 datalist for autocomplete functionality?
You can use jQuery AJAX and HTML5 datalist to create an autocomplete functionality. First, you need to create an input field and a datalist in your HTML. Then, you need to use jQuery AJAX to fetch the data you want to use for the autocomplete suggestions. Once the AJAX request is successful, you can use the response data to populate the datalist. Here’s an example:<input list="your-datalist-id">
<datalist id="your-datalist-id"></datalist>
$.ajax({
url: 'your-server-side-script',
success: function(data) {
var dataList = $('#your-datalist-id');
dataList.empty();
$.each(data, function(index, value) {
dataList.append('<option value="'+value+'">');
});
}
});
In this code, replace ‘your-datalist-id’ with the ID of your datalist and ‘your-server-side-script’ with the URL of your server-side script.
How can I handle errors in jQuery AJAX requests?
jQuery AJAX provides several methods to handle errors. The most common method is the ‘error’ callback option. This option is a function that is called when the AJAX request fails. The function receives three arguments: the jqXHR object, a string describing the type of error, and an optional exception object if one occurred. Here’s an example:$.ajax({
url: 'your-server-side-script',
success: function(data) {
// handle successful response
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('AJAX request failed: ' + textStatus);
}
});
In this code, replace ‘your-server-side-script’ with the URL of your server-side script.
How can I use jQuery AJAX to send data to the server?
You can use the ‘data’ option in the jQuery AJAX method to send data to the server. The ‘data’ option should be an object where the property names are the data keys and the property values are the data values. The data is sent to the server in the body of the HTTP request. Here’s an example:$.ajax({
url: 'your-server-side-script',
method: 'POST',
data: {
key1: 'value1',
key2: 'value2'
},
success: function(response) {
// handle successful response
}
});
In this code, replace ‘your-server-side-script’ with the URL of your server-side script, ‘key1’ and ‘key2’ with your data keys, and ‘value1’ and ‘value2’ with your data values.
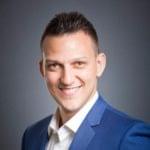
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.