I just quickly code the initial version of a jQuery plugin to dynamically create a Paypal Buy It Now Button. This is only v1.0 just to get a quick win, I am adding more features soon. There is a demo and I’ve committed the project to GitHub. Enhancements welcomed. I’ve used the basic jQuery namespace pattern for the plugin, I might upgrade to something slightly heavier for the next version. Stay tuned.
Usage
It’s pretty easy to use, you just specify your paypal email address, the item name and the options you want in the drop down box.
;(function($)
{
$(document).ready(function()
{
var options =
{
formId: 'myPaypalButton',
itemName: 'Buy a T-Shirt',
email: 'paypalemailadress@gmail.com',
basePrice: 59.00,
options:
{
sizes:
{
name: 'Please select your size:',
type: 'select',
values:
{
'Size - Small' : 60.00,
'Size - Medium' : 69.00,
'Size - Large' : 79.99,
'Size - Extra Large' : 89.00,
'Size - Too Big' : 199.95
}
}
},
countryCode: 'au', //au, uk
currencySymbol: '$',
currencyCode: 'AUD'
};
$('#demo1').paypalBuyButton(options);
});
})(jQuery);
The Plugin Code
Here is the development version of the plugin, the minified version is only 2kb!
/*
jQuery Paypal Buy It Now Plugin v1.0
Copyright (c) 2012 Sam Deering, jquery4u.com
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
"Software"), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
DEMO: http://samdeering.com/jquerypaypalbuyitnow/
DOWNLOAD: https://github.com/sdeering/jquerypaypalbuyitnow
ARTICLE: http://jquery4u.com/plugins/jquerypaypalbuyitnow/
*/
;(function($){
$.fn.extend({
paypalBuyButton: function(options)
{
this.defaultOptions = {};
var settings = $.extend({}, this.defaultOptions, options);
//construct form
var formHTML = '
';
//add options
$.each(settings.options, function(i, option)
{
switch(option.type)
{
case "select":
formHTML += '';
$.each(option.values, function(key, val)
{
formHTML += ''+key+' ($'+val+')';
});
formHTML += '';
//select box change handler
$('select[name="select-'+i+'"]').live('change', function(e)
{
console.log($(this).val());
$('#'+settings.formId+' input[name="amount"]').val($(this).val());
});
break;
default:
}
});
formHTML += '';
formHTML += '';
//add form to container
$(this).html(formHTML);
return this.each(function()
{
var $this = $(this);
});
}
});
})(jQuery);
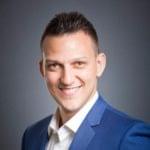
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.