Following on from 5 NEW JQUERY.AJAX() EXAMPLES JQUERY 1.9+, I wanted to document the changes (some could be classed as advantages) of using the promise interface for jQuery .Ajax() 1.9+.
- Naming – Obviously the names have changed from success -> done, error -> fail, complete -> always.
- Deferred – The deferred promises can be bound to anywhere in your application allowing flexibility and reusability.
- Order of callbacks – They are called in this order: 1 fail, 2 done, 3 always. Standard.
- Mutiples – You can specify any number of callbacks of the same type. ie .always(), .always(), .always() which will all get fired once the ajax request returns completes.
- Arguments
- Chaining – Since jQuery 1.8 you can chain ajax invocations using the .then() function. See below for example.
- Combining – You can combine .done() and .error() into .then(). See below for example.
//old complete function
complete Function( jqXHR jqXHR, String textStatus )
//new done function
jqXHR.done(function(data, textStatus, jqXHR) {});
Source: jQuery.Ajax API
Feel free to leave comments if you find any more I’ve missed.
Combine .done() and .fail() into .then()
You can combine the done() and fail() functions in one then() function. The code above can be rewritten as:var promise = $.ajax({
url: "/myServerScript"});
promise.then(mySuccessFunction, myErrorFunction);
Source: Deferred and promise in jQuery
Chaining Ajax Requests
Since jQuery 1.8, you can chain the then() function sequentially. In the code below, first promise1 is run and when resolved successfully, getStuff is run, returning a promise and when this is resolved successfully, the anonymous function is executed.var promise1 = $.ajax("/myServerScript1");
function getStuff() {
return $.ajax("/myServerScript2");}
promise1.then(getStuff).then(function(myServerScript2Data){
// Both promises are resolved});
Every callback function receives the result of the previous asynchronous function, in the case of Ajax, that would be the returned data.
Using .when() as promise
You can use .when() to allocate a promise callback function namely .done().var container = $("#mycontainer");
$.when(
function () {
return $.Deferred(function (dfd) {
container.fadeOut('slow', dfd.resolve);
}).promise();
}(),
$.ajax({
url: 'Path/To/My/Url/1',
type: 'POST',
dataType: 'json'
}),
$.ajax({
url: 'Path/To/My/Url/2',
type: 'POST',
dataType: 'json'
})
).done(function (x, data) {
container.html('Your request has been processed!');
container.fadeIn('slow');
});
As you can see we pass three promises to $.when, one for the fade out animation and two for the ajax operations.
* The first parameter is a self executing anonymous function that creates the deferred handler and returns the promise. In $.Deferred’s callback the dfd.resolve function is passed in to fadeOut()’s callback parameter, which means that once the animation completes the deferred will be resolved.
* With regards to the other two parameters we pass to $.when, since the result of $.ajax is a jqXHR object that implements Promise we just pass the value that’s returned as is.
Source: Always keep your (jQuery) Promises
Frequently Asked Questions about jQuery 1.9 AJAX Promise Interface Advantages
What is the main advantage of using jQuery 1.9 AJAX Promise Interface?
The primary advantage of using jQuery 1.9 AJAX Promise Interface is its ability to handle multiple AJAX requests simultaneously. This feature is particularly useful when you need to execute multiple AJAX requests and want to perform some action only when all the requests are completed. The Promise interface provides a way to aggregate the results of multiple AJAX requests and execute a callback function once all the requests are done. This makes the code more readable and manageable.
How does jQuery 1.9 AJAX Promise Interface improve error handling?
jQuery 1.9 AJAX Promise Interface improves error handling by providing a unified way to handle errors. Instead of having to write separate error handling code for each AJAX request, you can use the Promise interface to handle all errors in one place. This not only simplifies the code but also makes it easier to maintain and debug.
Can I use jQuery 1.9 AJAX Promise Interface with other versions of jQuery?
Yes, you can use jQuery 1.9 AJAX Promise Interface with other versions of jQuery. However, it’s important to note that the Promise interface was introduced in jQuery 1.5, so it won’t work with earlier versions. Also, some features of the Promise interface may not be available in later versions of jQuery, so it’s always a good idea to check the jQuery documentation for compatibility issues.
How does jQuery 1.9 AJAX Promise Interface compare to other methods of handling AJAX requests?
Compared to other methods of handling AJAX requests, jQuery 1.9 AJAX Promise Interface offers several advantages. It provides a more structured and organized way to handle multiple AJAX requests, improves error handling, and makes the code more readable and maintainable. However, it may be a bit more complex to use than other methods, especially for beginners.
What is the difference between jQuery’s Promise and Deferred objects?
Both Promise and Deferred objects in jQuery are used for managing asynchronous operations, but they serve different purposes. A Deferred object represents a unit of work that is not yet completed, while a Promise object represents the eventual result of that work. In other words, a Deferred object can be resolved or rejected, while a Promise object can only be fulfilled or rejected.
How can I convert a traditional AJAX call into a Promise-based AJAX call?
Converting a traditional AJAX call into a Promise-based AJAX call involves wrapping the AJAX call in a function that returns a Promise object. The Promise object is then resolved or rejected based on the success or failure of the AJAX call.
Can I use jQuery 1.9 AJAX Promise Interface with other JavaScript libraries?
Yes, you can use jQuery 1.9 AJAX Promise Interface with other JavaScript libraries. However, you need to be aware of potential compatibility issues, especially if the other libraries also use Promises or similar constructs.
How can I handle multiple AJAX requests with jQuery 1.9 AJAX Promise Interface?
You can handle multiple AJAX requests with jQuery 1.9 AJAX Promise Interface by using the $.when() function. This function takes multiple Promise objects as arguments and returns a new Promise object that is resolved when all the input Promise objects are resolved.
What happens if an AJAX request fails when using jQuery 1.9 AJAX Promise Interface?
If an AJAX request fails when using jQuery 1.9 AJAX Promise Interface, the Promise object associated with the request is rejected. You can handle this situation by attaching a .fail() handler to the Promise object, which will be called if the Promise is rejected.
Can I use jQuery 1.9 AJAX Promise Interface for non-AJAX asynchronous operations?
Yes, you can use jQuery 1.9 AJAX Promise Interface for non-AJAX asynchronous operations. The Promise interface is a general-purpose construct for managing asynchronous operations, so it can be used with any operation that may not complete immediately, such as reading a file or querying a database.
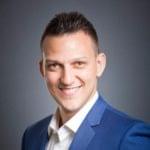
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.