$( document ).ajaxError(function( event, request, settings ) {
$( "#msg" ).append( "Error requesting page " + settings.url + "" );
});
Offical .ajaxError() API
Note: If $.ajax() or $.ajaxSetup() is called with the global option set to false, the .ajaxError() method will not fire.
Below is an older function which you can get the status from the jqXHR object.
$(function() {
$.ajaxSetup({
error: function(jqXHR, exception) {
if (jqXHR.status === 0) {
alert('Not connect.n Verify Network.');
} else if (jqXHR.status == 404) {
alert('Requested page not found. [404]');
} else if (jqXHR.status == 500) {
alert('Internal Server Error [500].');
} else if (exception === 'parsererror') {
alert('Requested JSON parse failed.');
} else if (exception === 'timeout') {
alert('Time out error.');
} else if (exception === 'abort') {
alert('Ajax request aborted.');
} else {
alert('Uncaught Error.n' + jqXHR.responseText);
}
}
});
});
Frequently Asked Questions (FAQs) on jQuery AJAX Error Handling Function
How can I handle multiple AJAX errors in jQuery?
Handling multiple AJAX errors in jQuery can be achieved by using the .ajaxError() method. This method sets a function to be called when AJAX requests complete with an error. It is a global event handler, and hence it will be triggered for every AJAX error, unless a request explicitly suppresses them. You can use this method to create a general error handler for all AJAX errors.
What is the difference between .ajaxError() and .ajaxComplete() in jQuery?
The .ajaxError() and .ajaxComplete() methods in jQuery are both global event handlers for AJAX requests, but they serve different purposes. The .ajaxError() method is triggered when an AJAX request completes with an error. On the other hand, .ajaxComplete() is triggered when an AJAX request completes, regardless of whether it was successful or not.
How can I display a custom error message using jQuery AJAX error handling?
You can display a custom error message using jQuery AJAX error handling by using the .ajaxError() method. Inside the function, you can specify the custom error message you want to display. Here’s an example:$(document).ajaxError(function(event, jqxhr, settings, thrownError) {
alert('Custom error: ' + thrownError);
});
How can I handle specific HTTP error codes using jQuery AJAX error handling?
You can handle specific HTTP error codes using jQuery AJAX error handling by using the .statusCode() method. This method allows you to specify a function to be executed when specific HTTP status codes are returned.
Can I use jQuery AJAX error handling with JSONP?
Yes, you can use jQuery AJAX error handling with JSONP. However, due to the nature of JSONP, error handling is somewhat limited. You can’t catch the errors using .ajaxError() or .error() methods because JSONP requests are not fired using XMLHTTPRequest.
How can I suppress global AJAX error handling for specific requests?
You can suppress global AJAX error handling for specific requests by setting the global option to false in the .ajax() method. This will prevent .ajaxError() and other global handlers from being triggered by these requests.
How can I handle timeout errors in jQuery AJAX?
You can handle timeout errors in jQuery AJAX by setting the timeout option in the .ajax() method and using the .ajaxError() method to handle the error.
How can I debug AJAX errors in jQuery?
You can debug AJAX errors in jQuery by using the .ajaxError() method. This method provides you with the event object, the jqXHR object, AJAX settings used in the request, and the error thrown. These can be used to identify and debug the error.
Can I use jQuery AJAX error handling with other jQuery AJAX methods like .load(), .get(), .post()?
Yes, you can use jQuery AJAX error handling with other jQuery AJAX methods like .load(), .get(), .post(). These methods internally use .ajax() method, and hence the global AJAX error handlers will be triggered when these methods complete with an error.
How can I handle parser errors in jQuery AJAX?
You can handle parser errors in jQuery AJAX by using the .ajaxError() method. A parser error is thrown when the server returns data in a format that can’t be parsed by jQuery. You can identify this error by checking the ‘thrownError’ parameter in the .ajaxError() method.
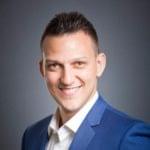
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.