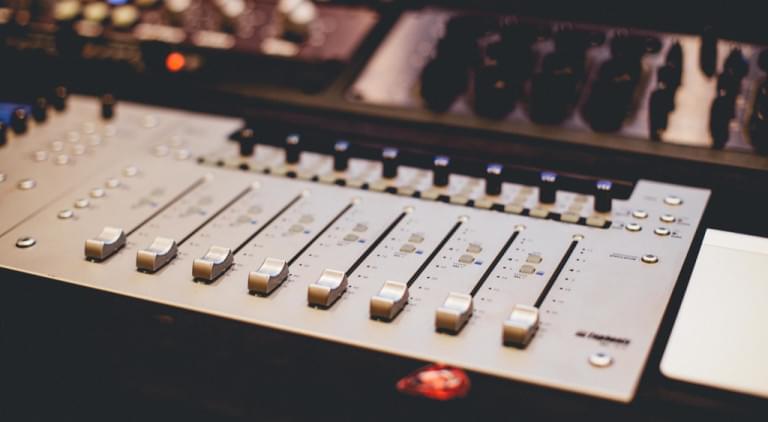
Key Takeaways
- Java’s switch statement allows selection of a particular execution path based on a variable’s value. It can be used with variables of type int, byte, short, char, String, or an enum. The statement compares the variable with a list of case labels and executes the corresponding block once a match is found.
- It’s important to note that without a break keyword, the switch statement will continue to execute the next branch, even after a match is found. This is called fall-through and can be used intentionally. However, many developers avoid this by placing switches into methods and returning a value, eliminating the need for a break.
- The switch statement cannot be used with boolean expressions or directly with arrays or ArrayLists. It only works with byte, short, char, int, enumerated types, String objects, and certain wrapper types like Byte, Short, Char, and Integer. If no case matches the switch expression and there is no default case, none of the statements in the switch block will be executed.
Table of Contents
Java’s switch statement allows the comfortable selection of one of many execution paths based on a variable’s value. The variable must either be an enum, a String
, or an integral type like int
. Given to switch
, it is compared with a list of case
labels that each specify a value – as soon as the first one matches, the corresponding statement block is executed. The switch statement works much like a long if
–else
–if
chain and can often be used to replace it.
This article only requires working knowledge of integers and strings but the more you know about if
and particularly if
–else
–if
the more you will get out of it. Experience with other numeric types, enums and methods are a bonus.
Using switch
Let’s jump right in and start with an example! The following switch statement writes a textual representation of the first three natural numbers to the console:
// any number is fine
int number = 2;
switch (number) {
case 0:
System.out.println("zero");
break;
case 1:
System.out.println("one");
break;
case 2:
System.out.println("two");
break;
default:
System.out.println("many");
break;
}
Just from looking at it, what do you think will happen?
The switch will look at number
, which is currently 2
, and compare it with each of the values behind the case
keywords. It’s not 0
, it’s not 1
, it’s 2
. Bingo! So off it goes calling System.out.println("two")
.
Syntax of switch
You can use the switch
statement with variables of type int
, byte
, short
, char
(note that long
does not work), String
, or an enum (or enumeration type as they are formally called). Here’s how it works:
switch (<variable>) {
case <value>:
// statements
break;
case <other-value>:
// statements
break;
case <more-values>:
// statements
break;
default:
// statements
break;
}
You use the keyword switch
followed by the variable you want to switch over (as it is commonly phrased) and a pair of curly braces. Inside those curly braces, you list as many branches as you like.
Each regular branch consists of the keyword case
, a value that matches the variable’s type (meaning it could be assigned to it), and a colon. Together, these three things are called a switch label. It is followed by the statements you want to execute if the variable has that particular value. Unless you have a very good reason, every switch-branch should end in a break
. (I’ll explain in a minute, why.)
If you need a branch that is executed if none of the labels matched, you can create one with default
. It works much like a regular branch but takes no value.
Fall-Through
It is important to note that the switch statement does not only execute the matching branch. Instead it starts executing code at the matching label. If it does not hit a break
it will go right on into the next branch. This is called fall through and can be used intentionally.
The following statement starts counting at the given number:
// any number is fine
int number = 1;
switch (number) {
case 0:
System.out.println("zero");
// fall-through intended
case 1:
System.out.println("one");
// fall-through intended
case 2:
System.out.println("two");
// fall-through intended
default:
System.out.println("many");
}
In this case, the output is one two many
because number
matches 1
and switch executes all blocks because there are no breaks. The comment “fall-through intended” tells your fellow programmers that you did this on purpose and not just forgot to add the break
.
Return Inside switch
Many developers consider repeating break
ugly and try to push switches into methods, so that they can return a value. Because a return
ends execution of that method, there is no risk to fall through and hence no need for break
.
For the first example, the code might look as follows:
public String toEnglish(int number) {
switch (number) {
case 0: return "zero";
case 1: return "one";
case 2: return "two";
default: return "many";
}
}
Much shorter, isn’t it? Executing System.out.println(toEnglish(2))
would then print two
.
Other Types
I already mentioned that you can switch over more than just int
s. Other primitives that allow that are byte
, short
, and char
. You can also switch over they wrapper types Integer
, Byte
, Short
, and Character
but note that you will get a NullPointerException
if the variable is null
.
You can also switch over String
…
public int toNumber(String number) {
switch (number) {
case "zero": return 0;
case "one": return 1;
case "two": return 2;
default: throw new IllegalArgumentException();
}
}
… and enums.
public enum LittleNumber { ZERO, ONE, TWO }
public int toNumber(LittleNumber number) {
switch (variable) {
case ZERO: return "zero (0)";
case ONE: return "one (1)";
case TWO: return "two (2)";
default: return "unknown";
}
}
Summary
You learned how the switch statement is put together with the switch
keyword, labels (case
, a value, and a colon or default
and a colon) and code blocks. On the first match, the code is executed until it hits a break
, which might mean that it falls through into following branches. To keep switches short, it is common to put them into methods and return the results, thus avoiding breaks.
Armed with this knowledge, you can dive deeper. If you wonder why you can’t use long
, give this StackOverflow question a go. Also, you might soon wish there were ways to give the case
branches a little more expressiveness, for example by checking whether a numerical value is smaller than another. Then you’re thinking towards pattern matching, a feature Java does not yet support directly but may soon. Until then, library implementations can be a replacement and Javaslang has a good one.
Frequently Asked Questions (FAQs) about Java’s Switch Statement
What is the default case in a Java switch statement?
The default case in a Java switch statement is a special case that is executed when none of the other cases match the switch expression. It’s similar to the “else” clause in an if-else statement. The default case is optional, but it’s good practice to include it as a catch-all for unexpected values. It’s typically placed at the end of the switch statement, but it can technically be placed anywhere within the switch block.
Can I use a string in a switch statement in Java?
Yes, starting from Java SE 7, you can use a String object in the expression of a switch statement. The switch statement compares the String object in its expression with the expressions associated with each case label as if it were using the String.equals method. Therefore, the comparison of String objects in switch statements is case sensitive.
How does the break keyword work in a switch statement?
The break keyword is used to exit the switch statement after a case has been matched and its statements executed. If a break statement is not included in a case, the program will continue to execute the next case’s statements, regardless of whether the case matches the switch expression. This is known as “falling through” cases.
Can I use switch statements with boolean expressions?
No, switch statements in Java cannot be used with boolean expressions. They only work with byte, short, char, int, enumerated types, String objects, and certain wrapper types like Byte, Short, Char, and Integer.
What happens if no case matches in a switch statement?
If no case matches the switch expression and there is no default case, then none of the statements in the switch block will be executed. The program will simply continue with the code following the switch statement.
Can I use non-constant expressions for case labels in a switch statement?
No, the expressions associated with case labels in a switch statement must be constant expressions. They can’t be variables or expressions that compute values at runtime.
Can I have multiple cases execute the same code in a switch statement?
Yes, you can have multiple cases execute the same code by stacking the cases on top of each other without break statements in between. This is known as “case fall-through”.
Can I use a switch statement inside another switch statement?
Yes, you can nest switch statements within other switch statements. This is known as a “nested switch statement”. However, nested switch statements can be difficult to read and understand, so they should be used sparingly.
Can I use a switch statement with arrays or ArrayLists?
No, switch statements in Java cannot be used directly with arrays or ArrayLists. They only work with the data types mentioned in Question 4.
How does the switch statement compare to if-else statements in terms of performance?
In general, the performance difference between switch statements and if-else statements is negligible and should not be a deciding factor in choosing one over the other. However, in certain situations where there are many cases, a switch statement may be slightly faster because it uses a jump table or hash list, while an if-else statement has to evaluate each condition sequentially.
Nicolai is a thirty year old boy, as the narrator would put it, who has found his passion in software development. He constantly reads, thinks, and writes about it, and codes for a living as well as for fun. Nicolai is the former editor of SitePoint's Java channel, writes The Java 9 Module System with Manning, blogs about software development on codefx.org, and is a long-tail contributor to several open source projects. You can hire him for all kinds of things.
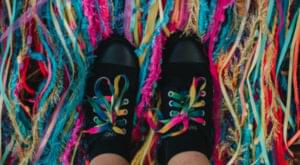
Published in
·Accessibility·Bootstrap·Design·Design & UX·HTML & CSS·Patterns & Practices·UX·February 12, 2018