Many WordPress plugins retrieve posts from the database by customizing the sorting order, retrieving posts based on a specific meta key or taxonomy. Have you ever wondered how these plugins retrieve customized lists of posts without writing any SQL queries? In this tutorial we’ll learn how to do exactly that.
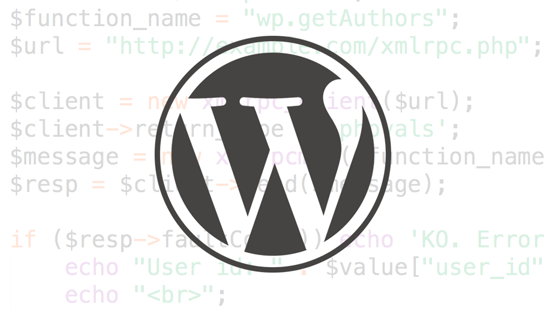
In this article we’ll explore the get_posts()
function with some examples of how to use it in your own projects. We’ll also cover some typical use cases for this function and how it’s different from the WP_Query
object and get_pages
function.
What is the get_posts()
Function?
The get_posts
function has been available in WordPress core since WordPress 1.2.0. This function is basically used to retrieve posts from the database by applying custom filters and sorting the final result based on a set of parameters.
The get_posts()
function returns an array of WP_Post
objects. Each WP_Post
object represents an individual post.
Internally get_posts
uses the WP_Query
object to construct and execute the SQL queries based on the passed set of parameters.
Note: Posts means post, page and custom post type.
Why Not Use the WP_Query
Object Directly?
Plugins use get_posts
function instead of WP_Query
object because using the WP_Query
object directly alters the main loop (i.e., the global $wp_query
variable) which would cause site issues.
What Is the Difference Between get_posts()
and get_pages()
Functions?
Both of them are used to retrieve posts from the WordPress database, however, here are some of the differences between them:
- Several of the parameter names and values differ between them. Although they behave the same way regardless of the names of the parameters.
- The
get_pages()
function currently does not retrieve posts based onmeta_key
andmeta_value
parameters. - The
get_pages()
function doesn’t use theWP_Query
object. Instead, it constructs and executes SQL queries directly.
get_posts()
Function Parameters
The get_posts
function takes only one argument as an array. The array contains the different parameters to apply custom filters and sort the result.
Here’s a code example which shows how to call this function and the various parameters available:
<?php
$args = array(
"posts_per_page" => 5,
"paged" => 1
"tax_query" => array(
array(
"taxonomy" => "category",
"field" => "slug",
"terms" => "videos,movies",
)
),
"orderby" => "post_date",
"order" => "DESC",
"exclude" => "1123, 4456",
"meta_key" => "",
"meta_value" => "",
"post_type" => "post",
"post_status" => "publish"
);
$posts_array = get_posts($args);
?>
There are more parameters available, but these are the most commonly used ones. Let’s look at each of these parameters:
- posts_per_page: This parameter defines the number of posts to return. Use -1 if you want all the posts.
- paged: Allows us to navigate between a set of posts while using the
posts_per_page
parameter. It is used for pagination. For example: supposeposts_per_page
is 10 and there are 20 posts in the result, then if you assignpaged
to 2 then last 10 posts are returned. - tax_query: Display posts of a particular taxonomy slug i.e., filter out posts of the other taxonomy slug.
terms
can take a comma separated string representing multiple taxonomy slugs. - orderby: It’s used to sort the retrieved posts. Some possible values are: “none”, “date”, “rand”, “comment_count”, “meta_value”, “meta_value_num” etc. While sorting using “meta_value” and “meta_value_num” you need to provide the
meta_key
parameter. - order: Designates the ascending or descending order of the
orderby
parameter. Possible values are “DESC” or “ASC”. - exclude: It takes a comma separated list of post IDs which will be excluded from a database search.
- meta_key and meta_value: If you provide only
meta_key
, then posts which have the key will be returned. If you also providemeta_value
then posts matching themeta_value
for themeta_key
is returned. - post_type: Retrieves content based on post, page or custom post type. Remember that the default
post_type
is only set to display posts but not pages. - post_status: Retrieves posts by status of the post. Possible values are: “publish”, “pending”, “draft”, “future”, “any” or “trash”.
The WP_Post
Object
The get_posts
function returns an array that contains WP_Post
objects. Here are the important properties of the WP_Post
object:
- ID: ID of the post
- post_author: Author name of the post
- post_type: Type of the post
- post_title: Title of the post
- post_date: Date on which post was published. Format: 0000-00-00 00:00:00
- post_content: Content of the post.
- post_status: Status of the post
- comment_count: Number of comments for the post
Examples of get_posts
Let’s check out some examples using the get_posts
function.
Most Popular Posts
If you want to display the top n number of the most discussed posts on your site, then you can use get_posts
to retrieve them. Here’s an example:
<?php
$args = array("posts_per_page" => 10, "orderby" => "comment_count");
$posts_array = get_posts($args);
foreach($posts_array as $post)
{
echo "<h1>" . $post->post_title . "</h1><br>";
echo "<p>" . $post->post_content . "</p><br>";
}
?>
Here, we are using the orderby
parameter to sort the posts based on the number of comments, retrieving the top 10 posts.
Random Posts
You can also easily retrieve random posts. This is helpful to recommend users another article on your site once they’ve finished reading the current one. Here’s the code for this:
<?php
$args = array("posts_per_page" => 1, "orderby" => "rand");
$posts_array = get_posts($args);
foreach($posts_array as $post)
{
echo "<h1>" . $post->post_title . "</h1><br>";
echo "<p>" . $post->post_content . "</p><br>";
}
?>
In the above example, we passed the value rand
to the order_by
parameter.
Posts with Matching Meta Key and Value
We might want to retrieve all posts which have a particular meta key and value assigned. For example: some blogs have a reviewer for every article. We might want to retrieve articles reviewed by a particular reviewer.
Here is the code to do just that:
<?php
$args = array("posts_per_page" => -1, "meta_key" => "reviewer", "meta_value" = "narayanprusty");
$posts_array = get_posts($args);
foreach($posts_array as $post)
{
echo "<h1>" . $post->post_title . "</h1><br>";
echo "<p>" . $post->post_content . "</p><br>";
}
?>
Here, we’re retrieving all the posts reviewed by “narayanprusty”. We’re assuming the reviewer name is stored via the meta key “reviewer” for every post.
Custom Post Type with Custom Taxonomy
We may want to retrieve posts of a custom post type with a custom taxonomy name. Consider this code example:
<?php
$args = array("posts_per_page" => -1, "post_type" => "coupons", "tax_query" => array(
array(
"taxonomy" => "coupon_category",
"field" => "slug",
"terms" => "plugins,themes",
)
));
$posts_array = get_posts($args);
foreach($posts_array as $post)
{
echo "<h1>" . $post->post_title . "</h1><br>";
echo "<p>" . $post->post_content . "</p><br>";
}
?>
In this example, we’re retrieving the posts of a custom post type named “coupons” which belong to the “plugins” and “themes” custom taxonomies.
Conclusion
In this article we saw how the get_posts
function works, the various parameters it supports, looping through the returned result and some sample use cases. The get_posts
function is one of the most used WordPress functions, I hope you can now start using it your own projects.
Frequently Asked Questions (FAQs) about WordPress get_posts Function
What is the difference between get_posts and WP_Query in WordPress?
Both get_posts and WP_Query are used to retrieve posts from your WordPress database. However, they differ in their usage and flexibility. get_posts is a simpler function, ideal for beginners and for situations where you only need to retrieve a specific set of posts. On the other hand, WP_Query is more powerful and flexible. It allows for more complex queries and gives you more control over the WordPress loop. While get_posts is easier to use, WP_Query provides more advanced features for customizing your queries.
How can I modify the number of posts returned by get_posts?
You can control the number of posts returned by get_posts by using the ‘numberposts’ parameter. By default, it is set to 5. If you want to retrieve all posts, you can set ‘numberposts’ to -1. For example, to get 10 posts, you would use: get_posts(array(‘numberposts’ => 10));
Can I use get_posts to retrieve custom post types?
Yes, you can use get_posts to retrieve custom post types. You just need to specify the ‘post_type’ parameter in your query. For example, if you have a custom post type called ‘products’, you can retrieve these posts with: get_posts(array(‘post_type’ => ‘products’));
How can I sort the posts returned by get_posts?
You can sort the posts returned by get_posts by using the ‘orderby’ and ‘order’ parameters. ‘orderby’ determines the field to sort by, and ‘order’ determines the sorting order (ASC for ascending and DESC for descending). For example, to sort posts by title in ascending order, you would use: get_posts(array(‘orderby’ => ‘title’, ‘order’ => ‘ASC’));
Can I use get_posts to retrieve posts from a specific category?
Yes, you can use get_posts to retrieve posts from a specific category. You just need to specify the ‘category’ parameter in your query. For example, to get posts from the category with the ID 3, you would use: get_posts(array(‘category’ => 3));
How can I retrieve posts from multiple categories using get_posts?
To retrieve posts from multiple categories, you can pass an array of category IDs to the ‘category’ parameter. For example, to get posts from the categories with the IDs 3 and 4, you would use: get_posts(array(‘category’ => array(3, 4)));
Can I use get_posts to retrieve posts by a specific author?
Yes, you can use get_posts to retrieve posts by a specific author. You just need to specify the ‘author’ parameter in your query. For example, to get posts by the author with the ID 1, you would use: get_posts(array(‘author’ => 1));
How can I retrieve posts that contain a specific meta value using get_posts?
To retrieve posts that contain a specific meta value, you can use the ‘meta_key’ and ‘meta_value’ parameters. For example, to get posts that have a meta key of ‘color’ and a meta value of ‘blue’, you would use: get_posts(array(‘meta_key’ => ‘color’, ‘meta_value’ => ‘blue’));
Can I use get_posts to retrieve posts that have a specific tag?
Yes, you can use get_posts to retrieve posts that have a specific tag. You just need to specify the ‘tag’ parameter in your query. For example, to get posts that have the tag ‘WordPress’, you would use: get_posts(array(‘tag’ => ‘WordPress’));
How can I retrieve posts from a specific date range using get_posts?
To retrieve posts from a specific date range, you can use the ‘date_query’ parameter. This parameter accepts an array of arrays, with each inner array defining a date query clause. For example, to get posts from January 2020, you would use: get_posts(array(‘date_query’ => array(array(‘year’ => 2020, ‘month’ => 1))));

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.