Into 2013… And the way we use the jQuery.Ajax() function has changed over recent versions. With this in mind, and older examples being slightly outdated I have coded up 5 New jQuery.ajax() Examples jQuery 1.9+ to show how you might use Ajax with newer versions of jQuery 1.9.x and 2.0.
The new way has some advantages over the old way.
I’ll try keep this post updated with skeleton Ajax snippets for reference. As always comments welcome.
- The .done() method replaces the deprecated jqXHR.success() method.
- The .fail() method replaces the deprecated .error() method.
- The .always() method replaces the deprecated .complete() method.
jQuery 1.9+ Ajax Example 1 – Subscribe to newsletter
This exampe shows a basic Ajax request for subscribing to a newsletter to send name and email to a backend script.var subscribeRequest = $.ajax({
type: "POST",
url: "subscribe.php",
data: { subscriberName: $('#name').val(), emailAddress: $('#email').val() }
});
subscribeRequest.done(function(msg)
{
alert( "You have successfully subscribed to our mailing list." );
});
subscribeRequest.fail(function(jqXHR, textStatus)
{
alert( "We could not subscribe you please try again or contact us if the problem persists (" + textStatus + ")." );
});
jQuery 1.9+ Ajax Example 2 – Request Timeout
This exampe shows how you might catch errors and fails such as timeouts for an ajax request.Set a timeout (in milliseconds) for the request. The timeout period starts at the point the $.ajax call is made; if several other requests are in progress and the browser has no connections available, it is possible for a request to time out before it can be sent. In Firefox 3.0+ only, script and JSONP requests cannot be cancelled by a timeout; the script will run even if it arrives after the timeout period.
var newDataRequest = $.ajax({
url: "getNewData.php",
timeout: 30000, // timeout after 30 seconds
data: { timestamp: new Date().getTime() }
});
newDataRequest.done(function(data)
{
console.log(data);
});
newDataRequest.fail(function(jqXHR, textStatus)
{
if (jqXHR.status === 0)
{
alert('Not connect.n Verify Network.');
}
else if (jqXHR.status == 404)
{
alert('Requested page not found. [404]');
}
else if (jqXHR.status == 500)
{
alert('Internal Server Error [500].');
}
else if (exception === 'parsererror')
{
alert('Requested JSON parse failed.');
}
else if (exception === 'timeout')
{
alert('Time out error.');
}
else if (exception === 'abort')
{
alert('Ajax request aborted.');
}
else
{
alert('Uncaught Error.n' + jqXHR.responseText);
}
});
jQuery 1.9+ Ajax Example 3 – Datafilter
This exampe shows how you can use the dataFilter function to process the raw data which is returned by your Ajax request.var filterDataRequest = $.ajax({
url: "getData.php",
dataFilter: function (data, type)
{
//include any conditions to filter data here...
//some examples below...
//eg1 - remove all commas from returned data
return data.replace(",", "");
//eg2 - if data is json process it in some way
if (type === 'json')
{
var parsed_data = JSON.parse(data);
$.each(parsed_data, function(i, item)
{
//process the json data
});
return JSON.stringify(parsed_data);
}
}
});
filterDataRequest.done(function(data)
{
console.log(data);
});
filterDataRequest.fail(function(jqXHR, textStatus)
{
console.log( "Ajax request failed... (" + textStatus + ' - ' + jqXHR.responseText ")." );
});
jQuery 1.9+ Ajax Example 4 – MIME Type
This example shows how to specify the content response header types available on a XMLHttpRequest.var contentTypeRequest = $.ajax({
url: "getData.php",
contentType: 'application/x-www-form-urlencoded; charset=UTF-8' //default
//or choose from one below
contentType: 'application/atom+xml' //Atom
contentType: 'text/css' //CSS
contentType: 'text/javascript' //JavaScript
contentType: 'image/jpeg' //JPEG Image
contentType: 'application/json' //JSON
contentType: 'application/pdf' //PDF
contentType: 'application/rss+xml; charset=ISO-8859-1' //RSS
contentType: 'text/plain' //Text (Plain)
contentType: 'text/xml' //XML
});
contentTypeRequest.done(function(data)
{
console.log(data);
});
contentTypeRequest.fail(function(jqXHR, textStatus)
{
console.log( "Ajax request failed... (" + textStatus + ' - ' + jqXHR.responseText ")." );
});
//set specific accept headers (should work cross browser)
$.ajax({
headers: {
Accept : "text/plain; charset=utf-8",
"Content-Type": "text/plain; charset=utf-8"
}
})
//an alternative to the above
$.ajax({
beforeSend: function(xhrObj)
{
xhrObj.setRequestHeader("Content-Type","application/json");
xhrObj.setRequestHeader("Accept","application/json");
}
});
jQuery 1.9+ Ajax Example 5 – Parsing XML
This example i grabbed from the jQuery docs Specifying the data type for ajax requests. It shows loading XML returned from a script and parsing the data as XML (if received by Internet Explorer as plain-text, not text/xml).var getXMLRequest = $.ajax({
url: "data.xml.php",
contentType: "text/xml"
});
getXMLRequest.done(function(data)
{
console.log(data);
var xml;
if (typeof data == "string")
{
xml = new ActiveXObject("Microsoft.XMLDOM");
xml.async = false;
xml.loadXML(data);
}
else
{
xml = data;
}
// Returned data available in object "xml"
});
getXMLRequest.fail(function(jqXHR, textStatus)
{
console.log( "Ajax request failed... (" + textStatus + ' - ' + jqXHR.responseText ")." );
});
Frequently Asked Questions (FAQs) about jQuery AJAX
What is the difference between $.ajax() and $.get() in jQuery?
Both $.ajax() and $.get() are methods used in jQuery for making AJAX requests. However, there are some key differences between the two. The $.ajax() method is a powerful and flexible method that allows you to make any kind of HTTP request. It provides a wide range of options to customize the request, such as specifying the type of data expected from the server, setting timeout limits, and handling errors.
On the other hand, $.get() is a shorthand method for making GET requests. It is less flexible than $.ajax() but is simpler to use. It is ideal for simple, straightforward GET requests where you don’t need to customize the request or handle errors in a specific way.
How can I handle errors in jQuery AJAX?
Error handling in jQuery AJAX can be done using the .fail() method. This method is called when the request fails. It takes a function as an argument, which will be executed when the request fails. The function can take three parameters: jqXHR (a superset of the native XMLHttpRequest object), textStatus, and errorThrown. Here’s an example:$.ajax({
url: "test.html"
}).done(function() {
alert("success");
}).fail(function(jqXHR, textStatus, errorThrown) {
alert("error");
});
In this example, if the request to “test.html” fails, an alert box with the message “error” will be displayed.
How can I send data to the server using jQuery AJAX?
You can send data to the server using the ‘data’ option in the $.ajax() method. The ‘data’ option allows you to specify the data you want to send to the server. This data should be in the form of an object, where each property is a key/value pair representing a data item. Here’s an example:$.ajax({
url: "test.php",
type: "POST",
data: {
name: "John",
location: "Boston"
}
});
In this example, the data { name: “John”, location: “Boston” } is sent to the server.
How can I make a synchronous AJAX request in jQuery?
By default, all AJAX requests in jQuery are asynchronous, which means they don’t block the execution of the rest of the code. However, you can make a synchronous request by setting the ‘async’ option to false in the $.ajax() method. Here’s an example:$.ajax({
url: "test.html",
async: false
});
Please note that synchronous requests can lock the browser, making it unresponsive until the request is complete. Therefore, they are generally not recommended.
How can I use the .done() and .fail() methods in jQuery AJAX?
The .done() and .fail() methods in jQuery AJAX are used to handle the success and failure of the AJAX request, respectively. The .done() method is called when the AJAX request is successful, and the .fail() method is called when the request fails. Both methods take a function as an argument, which will be executed when the request is successful or fails. Here’s an example:$.ajax({
url: "test.html"
}).done(function() {
alert("success");
}).fail(function() {
alert("error");
});
In this example, if the request to “test.html” is successful, an alert box with the message “success” will be displayed. If the request fails, an alert box with the message “error” will be displayed.
How can I specify the type of data expected from the server in jQuery AJAX?
You can specify the type of data expected from the server using the ‘dataType’ option in the $.ajax() method. The ‘dataType’ option allows you to specify the type of data that you’re expecting from the server, such as “json”, “xml”, “html”, or “text”. Here’s an example:$.ajax({
url: "test.php",
dataType: "json"
});
In this example, the AJAX request expects a JSON response from the server.
How can I set a timeout for a jQuery AJAX request?
You can set a timeout for a jQuery AJAX request using the ‘timeout’ option in the $.ajax() method. The ‘timeout’ option allows you to specify a timeout (in milliseconds) for the request. If the request is not completed within the specified time, it will be aborted and the .fail() method will be called. Here’s an example:$.ajax({
url: "test.html",
timeout: 5000
});
In this example, if the request to “test.html” is not completed within 5 seconds (5000 milliseconds), it will be aborted.
How can I make a POST request using jQuery AJAX?
You can make a POST request using the ‘type’ option in the $.ajax() method. The ‘type’ option allows you to specify the type of request to make (“POST”, “GET”, etc.). Here’s an example:$.ajax({
url: "test.php",
type: "POST",
data: {
name: "John",
location: "Boston"
}
});
In this example, a POST request is made to “test.php” with the data { name: “John”, location: “Boston” }.
How can I use the .always() method in jQuery AJAX?
The .always() method in jQuery AJAX is used to specify a function that will be executed no matter whether the request is successful or fails. This function is useful for performing cleanup tasks, such as hiding a loading spinner. Here’s an example:$.ajax({
url: "test.html"
}).done(function() {
alert("success");
}).fail(function() {
alert("error");
}).always(function() {
alert("complete");
});
In this example, no matter whether the request to “test.html” is successful or fails, an alert box with the message “complete” will be displayed.
How can I abort a jQuery AJAX request?
You can abort a jQuery AJAX request using the .abort() method. The .abort() method allows you to terminate the request before it has completed. Here’s an example:var request = $.ajax({
url: "test.html"
});
request.abort();
In this example, the request to “test.html” is immediately aborted.
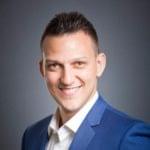
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.