Snippets are regularly-used chunks of code you can quickly insert into program files. They’re useful and a core feature of the Atom text editor. That said, you can use the editor for many months without realising snippets are available or appreciating their power!
Atom packages and language grammars are often supplied with pre-defined snippets. For example, start or open a new HTML file then type img
and hit the Tab key. The following code appears:
<img src="" alt="" />
and the cursor will be between the src
attribute quotes. Hit Tab again and the cursor will move to the alt
attribute. Hit Tab a final time to move to the end of the tag.
Snippet trigger text is indicated with a green arrow as you start typing. You can view all defined snippets for your current file’s language type by placing the cursor anywhere and hitting Alt-Shift-S. Scroll or search through the list to locate and use a particular snippet.
Alternatively, open the Packages list in Settings and enter ‘language’ to view a list of all grammar types. Choose one and scroll to the Snippets section to view pre-defined triggers and code.
How to Create Your Own Snippets
You will have your own regularly-used code blocks which can be defined as snippets. A useful command I use when debugging Node.js applications is to log objects to the console as JSON strings:console.log('%j', Object);
Atom already has a pre-defined log
trigger for console.log();
but you can improve that with a custom snippet.
Custom snippets are defined in the snippets.cson
file located in your ~/.atom
folder. Select Open Your Snippets from the File menu to edit it. Snippets require the following information:
- the language identifier, or scope string
- a name which concisely describes the code
- the trigger text which fires the snippet once Tab is hit, and
- the snippet body code with optional tab stops.
snippets.cson
and type snip
followed by Tab — yes, there’s even a snippet to help you define snippets!…
'.source.js':
'Snippet Name':
'prefix': 'Snippet Trigger'
'body': 'Hello World!'
Note that CSON is CoffeeScript Object Notation. It’s a terse syntax which maps directly to JSON; in essence, indentation is used rather than {}
brackets.
First, you require the scope string which identifies the language where your snippet can be applied. The easiest way to determine the scope is to open the Packages list in Settings and enter ‘language’. Open the grammar package you require and look for the Scope definition near the top.
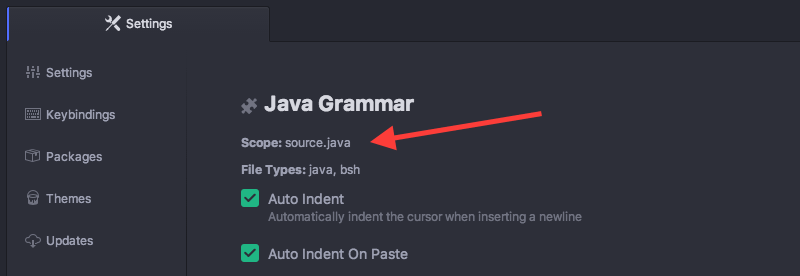
snippets.cson
must also have a period added to the start of that string. Popular web-language scopes include:
- HTML:
.text.html.basic
- CSS:
.source.css
- SASS:
.source.sass
- JavaScript:
.source.js
- JSON:
.source.json
- PHP:
.text.html.php
- Java:
.source.java
- Ruby:
.text.html.erb
- Python:
.source.python
- plain text (including markdown):
.text.plain
'.source.js':
'log JSON':
'prefix': 'lj'
'body': 'console.log(\'%j\', ${1:Object});$2'
The snippet becomes active as soon as your snippets.cson
file is saved.
In this example:
- the scope is set to
.source.js
for JavaScript - the snippet is named “
log JSON
“ - the Tab trigger (prefix) is set to
lj
- the snippet body is set to
console.log('%j', Object);
(however, we’ve added some additional control codes, as shown next).
\'
).
Tab stops are defined using a dollar followed by a number, i.e. $1, $2, $3 etc. $1 is the first tab stop location where the cursor appears. When Tab is hit, the cursor moves to $2 and so on.
Tab stop $1 above has been defined with default text to remind or prompt the user: ${1:Object}
. When the snippet is used, Object is selected within console.log('%j', Object);
so it can be changed to an appropriate object name.
Additional snippets can be added to the bottom of the snippets.cson
file. If you require two or more snippets for the same language, add them to the appropriate scope section. For example, you can create another JavaScript snippet in the '.source.js'
scope to log the length of any array:
'.source.js':
'log JSON':
'prefix': 'lj'
'body': 'console.log(\'%j\', ${1:Object});$2'
'log array length':
'prefix': 'llen'
'body': 'console.log(\'${1:array} length\', ${1:array}.length);$2'
Notice this has two ${1:array}
tab stops. When console.log('array length', array.length);
appears, you’ll see two cursors and both instances of array are highlighted — you need only type the array name once and both change!
Multi-line Snippets
If you’re feeling more adventurous, longer multi-line snippets can be defined using three double-quotes"""
at the start and end of the body code. This snippet generates a 2×2 table with a single header row:
'.text.html.basic':
'2x2 table':
'prefix': 'table2x2'
'body': """
<table summary="$1">
<thead>
<tr>
<th>$2</th>
<th>$3</th>
</tr>
</thead>
<tbody>
<tr>
<td>$4</td>
<td>$5</td>
</tr>
<tr>
<td>$6</td>
<td>$7</td>
</tr>
</tbody>
</table>
$8
"""
The code indentation within the snippet body doesn’t have any impact on the CSON definition, but I suggest you keep it indented beyond the body
definition to aid readability.
Happy snippetting!
If you’re new to Atom, you should also refer to 10 Essential Atom Add-ons for recommended packages.
Frequently Asked Questions (FAQs) about Using Code Snippets in Atom
How can I create a new snippet in Atom?
Creating a new snippet in Atom is a straightforward process. First, you need to open the snippets file by going to the ‘File’ menu, then ‘Snippets’. This will open a .cson file where you can define your snippets. Each snippet starts with a ‘.source’ line that specifies the language it applies to, followed by the name of the snippet in quotes. Then, you define the prefix that will trigger the snippet and the body of the snippet itself. The body can be multiple lines and uses the ‘${1:default_text}’ syntax to specify tab stops.
How can I use a snippet in Atom?
To use a snippet in Atom, you simply need to type the prefix you defined for the snippet and then press the ‘Tab’ key. This will insert the body of the snippet at your cursor’s location. If your snippet has tab stops, you can use the ‘Tab’ key to move between them and enter the desired text.
Can I use snippets for any programming language in Atom?
Yes, you can use snippets for any programming language that Atom supports. You just need to specify the correct scope for the language when defining your snippet. For example, for JavaScript, you would use ‘.source.js’, and for Python, you would use ‘.source.python’.
How can I share my snippets with others?
If you want to share your snippets with others, you can simply share your snippets.cson file. This file contains all your snippet definitions and can be found in your Atom configuration directory. Alternatively, you can create a package that includes your snippets and publish it to the Atom package repository.
Can I use snippets to insert commonly used code blocks?
Absolutely! Snippets are a great way to insert commonly used code blocks. You can define a snippet for any piece of code you find yourself typing frequently, and then insert it with just a few keystrokes. This can save you a lot of time and help ensure consistency in your code.
How can I edit an existing snippet in Atom?
To edit an existing snippet in Atom, you need to open the snippets.cson file and find the snippet you want to edit. You can then modify the prefix, body, or scope as needed. Remember to save the file when you’re done to apply your changes.
Can I use variables in my snippets?
Yes, you can use variables in your snippets. Variables are represented by ‘${1:default_text}’, where ‘1’ is the tab stop and ‘default_text’ is the default text that will be inserted. You can use variables to create placeholders in your snippets that you can quickly fill in when you insert the snippet.
How can I delete a snippet in Atom?
To delete a snippet in Atom, you need to open the snippets.cson file and find the snippet you want to delete. Then, simply delete the lines of code that define the snippet and save the file. The snippet will be removed immediately.
Can I import snippets from other editors into Atom?
While Atom doesn’t have a built-in feature for importing snippets from other editors, you can manually copy the snippet definitions from your other editor and paste them into your snippets.cson file in Atom. You may need to adjust the syntax slightly to match Atom’s snippet syntax.
Can I use snippets in Atom’s find and replace functionality?
Yes, you can use snippets in Atom’s find and replace functionality. When you open the find and replace panel, you can enter a snippet in the ‘replace’ field. When you perform the replace operation, the snippet will be inserted in place of the found text.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.