This is a quick step by step to setup a scroll to top for your website. For a demo, just scroll down on this site. 1. Download scrollTo plugin, include it. 2. Get an image (arrow or such). 3. Include some HTML below. 4. Include some jQuery/JavaScript below to catch window scroll and handle display of image. 5. Thats it!
HTML
jQuery
Just some a little jQuery to show the image when the user scrolls down and hide when they scroll up, and handle the click event.$(document).ready(function()
{
var $scrollTop = $('#scroll-to-top');
$(window).scroll(function()
{
//scroll top top
if ($(this).scrollTop() > 100)
{
$scrollTop.fadeIn(1000);
}
else
{
$scrollTop.hide();
}
});
$scrollTop.bind('click', function(e)
{
e.preventDefault();
$.scrollTo(0, 1000);
});
});
Note: If you want a cross browser solution just use this:
window.scrollTo(0,0); //chrome scroll to top bugfix
CSS
Just some simple CSS to handle showing of image and cross browser opacity.#scroll-to-top {
position: fixed;
bottom: 10px;
right: 10px;
display: none;
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=50)";
filter: alpha(opacity=50);
-moz-opacity: 0.5;
-khtml-opacity: 0.5;
opacity: 0.5;
}
#scroll-to-top:hover {
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=100)";
filter: alpha(opacity=100);
-moz-opacity: 1.0;
-khtml-opacity: 1.0;
opacity: 1.0;
}
Frequently Asked Questions (FAQs) about jQuery ScrollTop
How can I use jQuery ScrollTop to scroll to a specific element on the page?
To scroll to a specific element on the page using jQuery ScrollTop, you need to first select the element using jQuery selectors and then use the scrollTop method. Here’s an example:$('html, body').animate({
scrollTop: $("#myElement").offset().top
}, 2000);
In this code, “#myElement” is the ID of the element you want to scroll to, and 2000 is the duration of the scroll animation in milliseconds. This will smoothly scroll the page to the specified element over 2 seconds.
Can I use jQuery ScrollTop without animation?
Yes, you can use jQuery ScrollTop without animation. The animate method in jQuery is not mandatory for scrollTop. Here’s how you can do it:$('html, body').scrollTop($("#myElement").offset().top);
This will instantly scroll the page to the specified element.
How can I determine the current scroll position with jQuery ScrollTop?
You can determine the current scroll position using the scrollTop method without any arguments. Here’s how:var scrollPosition = $(window).scrollTop();
This will return the current vertical position of the scroll bar.
Can I use jQuery ScrollTop for horizontal scrolling?
No, jQuery ScrollTop is only for vertical scrolling. If you want to scroll horizontally, you can use the scrollLeft method.
How can I use jQuery ScrollTop in a scroll event?
You can use jQuery ScrollTop in a scroll event to perform actions based on the scroll position. Here’s an example:$(window).scroll(function() {
if ($(this).scrollTop() > 100) {
// Perform action when scroll position is greater than 100
}
});
In this code, the action will be performed when the scroll position is greater than 100 pixels.
Is jQuery ScrollTop compatible with all browsers?
jQuery ScrollTop is compatible with all modern browsers including Chrome, Firefox, Safari, Edge, and Internet Explorer 9+.
Can I use jQuery ScrollTop on elements other than window or document?
Yes, you can use jQuery ScrollTop on any scrollable element. You just need to select the element using jQuery selectors.
How can I set the scroll position to the bottom of the page with jQuery ScrollTop?
To set the scroll position to the bottom of the page, you can use the height method to get the total height of the document and then use scrollTop. Here’s how:$('html, body').scrollTop($(document).height());
This will instantly scroll to the bottom of the page.
Can I use jQuery ScrollTop to scroll to the top of an iframe?
Yes, you can use jQuery ScrollTop to scroll to the top of an iframe. You just need to select the iframe content document and then use scrollTop.
How can I stop the scrolling animation with jQuery ScrollTop?
You can stop the scrolling animation using the stop method in jQuery. Here’s how:$('html, body').stop();
This will immediately stop the current animation.
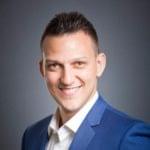
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.