Just building up a collection of input selection code snippets. The latest versions of Chrome and Firefox use the .setSelectionRange() function. Don’t forget Firefox needs focus first on an element before you can set the range. See Input.setSelectionRange. Related post: HTML5 Input Autofocus
Get Cursor Position
// GET CURSOR POSITION
jQuery.fn.getCursorPosition = function(){
if(this.lengh == 0) return -1;
return $(this).getSelectionStart();
}
Set Text Selection
jQuery.fn.getSelectionStart = function(){
if(this.lengh == 0) return -1;
input = this[0];
var pos = input.value.length;
if (input.createTextRange) {
var r = document.selection.createRange().duplicate();
r.moveEnd('character', input.value.length);
if (r.text == '')
pos = input.value.length;
pos = input.value.lastIndexOf(r.text);
} else if(typeof(input.selectionStart)!="undefined")
pos = input.selectionStart;
return pos;
}
Set Cursor Position
//SET CURSOR POSITION
jQuery.fn.setCursorPosition = function(pos) {
this.each(function(index, elem) {
if (elem.setSelectionRange) {
elem.setSelectionRange(pos, pos);
} else if (elem.createTextRange) {
var range = elem.createTextRange();
range.collapse(true);
range.moveEnd('character', pos);
range.moveStart('character', pos);
range.select();
}
});
return this;
};
//SET CURSOR POSITION
jQuery.fn.setCursorPosition = function(pos) {
this.each(function(index, elem) {
if (elem.setSelectionRange) {
elem.setSelectionRange(pos, pos);
} else if (elem.createTextRange) {
var range = elem.createTextRange();
range.collapse(true);
range.moveEnd('character', pos);
range.moveStart('character', pos);
range.select();
}
});
return this;
};
Set Cursor Position (v2)
//different version of SET CURSOR POSITION function above
function setCursorPos(node,pos){
var node = (typeof node == "string" || node instanceof String) ? document.getElementById(node) : node;
node.focus(); //crucial for firefox
if(!node){
return false;
}else if(node.createTextRange){
var textRange = node.createTextRange();
textRange.collapse(true);
// textRange.moveEnd(pos); //see api textRange requires 2 params
// textRange.moveStart(pos);
textRange.moveStart('character', pos);
textRange.moveEnd('character', 0);
// console.log('textRange...');
textRange.select();
return true;
}else if(node.setSelectionRange){
node.setSelectionRange(pos,pos);
// console.log('setSelectionRange...');
return true;
}
return false;
}
//different version of SET CURSOR POSITION function above
function setCursorPos(node,pos){
var node = (typeof node == "string" || node instanceof String) ? document.getElementById(node) : node;
node.focus(); //crucial for firefox
if(!node){
return false;
}else if(node.createTextRange){
var textRange = node.createTextRange();
textRange.collapse(true);
// textRange.moveEnd(pos); //see api textRange requires 2 params
// textRange.moveStart(pos);
textRange.moveStart('character', pos);
textRange.moveEnd('character', 0);
// console.log('textRange...');
textRange.select();
return true;
}else if(node.setSelectionRange){
node.setSelectionRange(pos,pos);
// console.log('setSelectionRange...');
return true;
}
return false;
}
Frequently Asked Questions about Input Text Selection Code Snippets
How can I use the setSelectionRange method in JavaScript?
The setSelectionRange method in JavaScript is used to select a portion of the text of a text field or a textarea. It takes two parameters: the start and end positions of the selection. Here’s a simple example:var input = document.getElementById('myInput');
input.setSelectionRange(0, 5);
In this example, the text from the 0th to the 5th character in the input field with the id ‘myInput’ will be selected.
What is the difference between selectionStart and selectionEnd properties?
The selectionStart and selectionEnd properties in JavaScript return the start and end positions of the selected text in a text field or a textarea. If no text is selected, both properties return the position of the caret. These properties are read/write, meaning you can also use them to set the selection.
How can I use the setSelectionRange method with jQuery?
To use the setSelectionRange method with jQuery, you first need to get the DOM element from the jQuery object using the get method or array notation. Here’s an example:var input = $('#myInput').get(0);
input.setSelectionRange(0, 5);
In this example, the text from the 0th to the 5th character in the input field with the id ‘myInput’ will be selected.
What is the purpose of the inputElement in the setSelectionRange method?
The inputElement in the setSelectionRange method refers to the text field or textarea where you want to set the selection. It’s the element on which you call the setSelectionRange method.
Can I use the setSelectionRange method on elements other than text fields and textareas?
No, the setSelectionRange method can only be used on text fields and textareas. If you try to use it on other elements, you’ll get a TypeError.
How can I check if a text field or textarea supports the setSelectionRange method?
You can check if a text field or textarea supports the setSelectionRange method by using the ‘in’ operator in JavaScript. Here’s an example:if ('setSelectionRange' in document.getElementById('myInput')) {
// The setSelectionRange method is supported
}
In this example, if the setSelectionRange method is supported by the input field with the id ‘myInput’, the code inside the if statement will be executed.
What happens if the start and end parameters of the setSelectionRange method are the same?
If the start and end parameters of the setSelectionRange method are the same, no text will be selected. Instead, the caret will be placed at the specified position.
Can I use negative values for the start and end parameters of the setSelectionRange method?
Yes, you can use negative values for the start and end parameters of the setSelectionRange method. A negative start parameter counts from the end of the input’s value. A negative end parameter will cause the end of the selection to be that many characters from the end of the input’s value.
What is the return value of the setSelectionRange method?
The setSelectionRange method does not return any value. Its sole purpose is to set the selection in a text field or textarea.
Can I use the setSelectionRange method in all browsers?
The setSelectionRange method is supported in all modern browsers, including Chrome, Firefox, Safari, and Edge. However, it’s not supported in Internet Explorer.
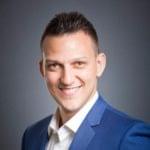
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.