What is Require.js?
RequireJS is a JavaScript file and module loader. It is optimized for in-browser use, but it can be used in other JavaScript environments, like Rhino and Node. Using a modular script loader like RequireJS will improve the speed and quality of your code.- Speed – Asynchronous JavaScript Loading.
- Manage JavaScript dependencies such as jQuery plugins.
- File Structure your web app files.
- When you get good you can code modules which do specific web app stuff.
- Removes the need for including 100 script tags in your HTML.
- Can be easily integrate with build scripts.
Does it work?
Yes. Screenshot below was taken from my dev with chrome dev tools open (disabling cache) so is naturally fast but amazingly even here you can see a performance increase.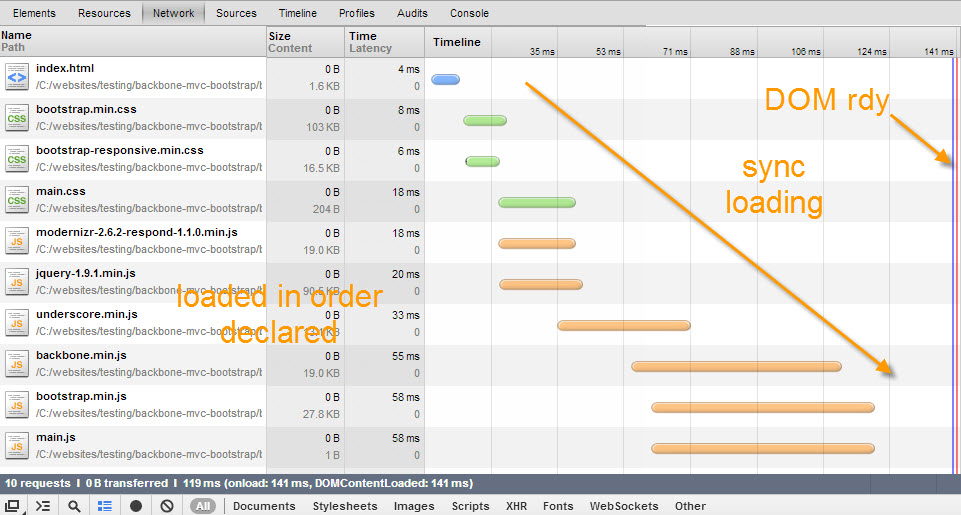
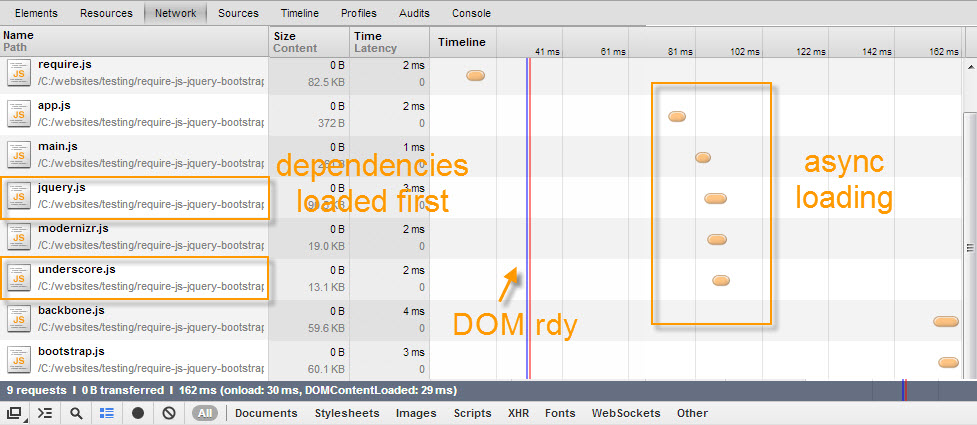
Web App Scructure
This is a very basic structure you can use for a web app.- root/
- index.html
- js
- vendor
- [External JavaScript Files & jQuery Plugins]
- app
- main.js
- [your modules and web app JavaScript files]
- app.js
- vendor
- css
- img
HTML Before:
The normal way to load scripts… modernizr goes in the head, the rest in the body.< !DOCTYPE html>
<head>
<title>My Web App</title>
<link rel="stylesheet" href="app/css/main.css"/>
<script src="app/js/vendor/modernizr-2.6.2-respond-1.1.0.min.js"></script>
</head>
<body>
<div id="main" class="container"></div>
<script src="app/js/vendor/jquery-1.9.1.min.js"></script>
<script src="app/js/vendor/underscore.min.js"></script>
<script src="app/js/vendor/backbone.min.js"></script>
<script src="app/js/vendor/bootstrap.min.js"></script>
<script src="app/js/main.js"></script>
</body>
HTML After:
Require.js goes in the head. Nice and neat.< !DOCTYPE html>
<head>
<title>My Web App</title>
<link rel="stylesheet" href="app/css/main.css"/>
<script data-main="js/app" src="js/vendor/require.js"></script>
</head>
<body>
<div id="main" class="container"></div>
</body>
app.js
This file contains the config for require.js. If you change the directory structure this needs to match. I’m showing you the shim version, you can also load jQuery from a CDN.// Place third party dependencies in the lib folder
//
// Configure loading modules from the lib directory,
requirejs.config({
"baseUrl": "js/vendor",
"paths": {
"app": "../app"
},
"shim": {
"backbone": ["jquery", "underscore"],
"bootstrap": ["jquery"]
}
});
// Load the main app module to start the app
requirejs(["app/main"]);
main.js
This file contains the web app dependencies and once loaded you can start your app using whatever framework you like such as Backbone or Angular.//Load Web App JavaScript Dependencies/Plugins
define([
"jquery",
"modernizr",
"underscore",
"backbone",
"bootstrap"
], function($)
{
$(function()
{
//do stuff
console.log('required plugins loaded...');
});
});
Still can’t get it working?
Download CodeFrequently Asked Questions (FAQs) about RequireJS Setup
What is the main purpose of RequireJS in JavaScript development?
RequireJS is a JavaScript file and module loader. It is optimized for in-browser use but can be used in other JavaScript environments as well. The main purpose of RequireJS is to encourage the use of modular JavaScript development by providing a clear structure for adding dependencies. This can significantly improve the speed and quality of your code, especially in large projects. It also helps in managing and loading JavaScript files in an efficient manner, which can be a significant advantage when working with complex projects that have a large number of scripts.
How does RequireJS handle dependencies?
RequireJS uses the Asynchronous Module Definition (AMD) API for JavaScript modules. These modules can be loaded asynchronously, which means that they can be loaded in parallel, but executed in the exact order you specify. This is particularly useful for handling dependencies in large-scale projects. You can define dependencies and then RequireJS ensures that they are loaded and available before executing the dependent code.
How can I define a module using RequireJS?
To define a module in RequireJS, you use the define() function. This function takes two arguments: an array of dependencies and a factory function. The dependencies are scripts that must be loaded before the module can be executed, and the factory function is the code that runs to create your module. Here’s an example:define(['dependency1', 'dependency2'], function (dep1, dep2) {
// Module code here
});
How can I load a module using RequireJS?
To load a module in RequireJS, you use the require() function. This function takes two arguments: an array of dependencies and a callback function. The dependencies are scripts that must be loaded before the callback can be executed, and the callback function is the code that runs after your dependencies have been loaded. Here’s an example:require(['module1', 'module2'], function (mod1, mod2) {
// Callback code here
});
Can I use RequireJS with other JavaScript libraries like jQuery?
Yes, RequireJS is compatible with other JavaScript libraries such as jQuery. You can include jQuery as a dependency in your modules or load it using the require() function. This allows you to take advantage of the modular structure and dependency management features of RequireJS while still using the features and functionality of jQuery.
How can I handle errors in RequireJS?
RequireJS provides an onError callback for handling errors. This callback is called whenever an error occurs while loading a module or its dependencies. You can use this callback to log errors or handle them in a way that is appropriate for your application.
Can I use RequireJS in Node.js?
Yes, RequireJS can be used in Node.js. However, Node.js has its own module system (CommonJS), so you may not need to use RequireJS. If you do choose to use RequireJS in Node.js, you can take advantage of its asynchronous loading and dependency management features.
How can I optimize my code with RequireJS?
RequireJS includes an optimization tool called r.js. This tool can concatenate and minify your scripts, as well as inline any text-based dependencies. This can significantly reduce the number of HTTP requests your application makes and improve its load time.
Can I use RequireJS with TypeScript?
Yes, RequireJS can be used with TypeScript. TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. You can use RequireJS to manage and load your TypeScript modules just like you would with JavaScript modules.
How can I configure RequireJS?
You can configure RequireJS using the require.config() function. This function allows you to set various configuration options for RequireJS, such as the base URL for scripts, paths to libraries, shim configurations for non-AMD scripts, and more. Here’s an example:require.config({
baseUrl: 'scripts',
paths: {
jquery: 'lib/jquery'
}
});
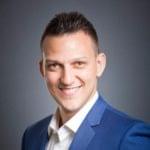
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.