Offline browsing is becoming increasingly important to web developers and designers. Giving the user the ability to use your website offline has always been a goal, but one that was pretty difficult to reach, to say the least. As we move into the age of HTML5, however, this is changing and you can now take advantage of the ApplicationCache interface. Using the application cache, you can specify which files the browser can cache and use when the user is offline. Your website will work as if the user is online, and best of all, they won’t notice any difference! So, how do you specify which files the browser should cache? Well, this is defined in the cache manifest file.
The Cache Manifest
The cache manifest file lives inside your website, and it defines which files can be cached by the browser. The cache manifest has anappcache
extension, and to use it, you need to reference it in the html tag of your webpage.
<!DOCTYPE HTML> <html manifest="offline.appcache">The catch is this must be included on every page. If it’s not there, the browser won’t cache the page. And what does that mean? Well, even if you don’t include the current HTML page in the manifest file, it will be explicitly cached by the browser too.
Cache Manifest Gotcha
Here’s something else to watch out for. For the web server to serve the cache manifest properly a new mime type of text/cache-manifest must be added, otherwise things won’t work as you may expect. To do this in IIS 7, select your website and click MIME Types.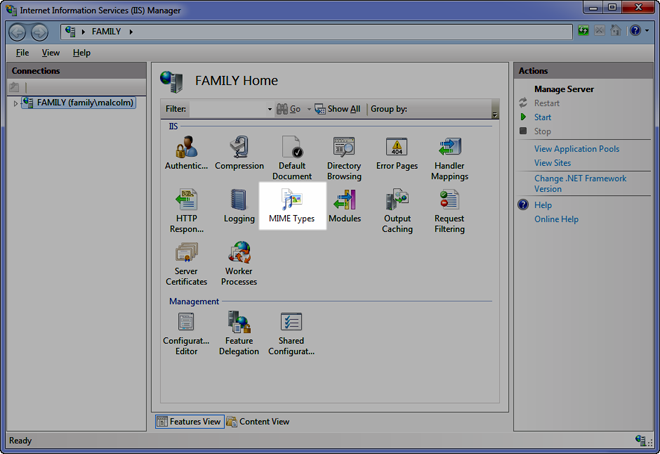
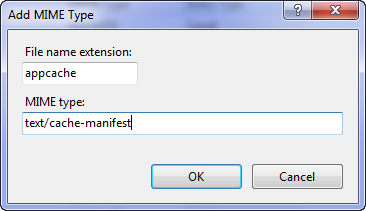
The Cache Manifest Structure
The cache manifest is broken up into three sections:- cache – defines which resources the browser can cache
- network – defines which resources requires the user to be online
- fallback – defines a fallback for resources that cannot be cached
CACHE MANIFEST # Created on 8 October 2011 CACHE: site.css site.js NETWORK: login.aspx currency.aspx # offline.jpg will replace all images in the images folder # offline.html will replace all html pages if they cannot be found FALLBACK: site/images images/offline.jpg *.html offline.htmlThe manifest file is not hard to understand. Lines beginning with # are comments and will be ignored by the browser. Each section tells the browser what can be cached, what can’t be cached and what to do when a resource can’t be found. These sections can be listed in any order. Before moving on, there’s one piece of information to remember at this point – and it’s very important. If one resource fails to download, the entire cache process fails. It’s all or nothing. If this happens, the browser will fall back to the old cached files. Bear that in mind.
Update the Application Cache
Caching resources improves performance, but it can also mean the resources are not current. This can happen if, for example, a resource is updated on a website, but the application cache remains cached until one of the following occurs:- the cache manifest file has changed
- the user clears their temporary internet files
- the application cache is programmatically updated
Application Cache and JavaScript
The application cache has many events that fire during the caching process. I really can’t think of too many occasions when you’d want to hook into these events, apart from manually forcing the cache to refresh, or writing a demo for a presentation. Nevertheless, here they are:onchecking
– the user agent is checking for updates, or attempting to download the manifest for the first time.onnoupdate
– no update to the manifest file.ondownloading
– the user agent has found an update, or attempting to download the manifest for the first time.onprogress
– the user agent is download the resources listed in the manifest.oncached
– the download is complete and the resources are cached.onupdateready
– the resources have been downloaded and they can be refreshed by calling swapCacheonobsolete
– the manifest is either a 404 or 410 page, so the application cache gets deleted.onerror
– caused by a number of items. The manifest is a 404 or 410 page. The manifest could have changed while an update was being run.
var appCache = window.applicationCache; function logEvent(e) { console.log(e); } function logError(e) { console.log("error " + e); }; appCache.addEventListener('cached', logEvent, false); appCache.addEventListener('checking', logEvent, false); appCache.addEventListener('downloading', logEvent, false); appCache.addEventListener('error', logError, false); appCache.addEventListener('noupdate', logEvent, false); appCache.addEventListener('obsolete', logEvent, false); appCache.addEventListener('progress', logEvent, false); appCache.addEventListener('updateready', logEvent, false);If you want to refresh the page for the user when the cache is cleared, you can add some extra code to the updateready event to handle that.
appCache.addEventListener('updateready', function (e) { appCache.swapCache(); window.location.reload(); }, false);As always you can check out the complete API reference here. This is one area of HTML5 that is a game changer for me. I recommend you come to know it, love it and use it.
Frequently Asked Questions (FAQs) about Offline Browsing in HTML5 with ApplicationCache
What is the ApplicationCache in HTML5 and how does it enable offline browsing?
The ApplicationCache, also known as AppCache, is a feature in HTML5 that allows users to store web data locally. This means that even when you’re offline, you can still access certain web pages or applications that you’ve previously visited. The AppCache stores a version of the web files in your local system, which can be accessed without an internet connection. This is particularly useful for mobile applications that need to provide an offline mode.
How do I implement ApplicationCache in my web application?
Implementing ApplicationCache involves creating a cache manifest file, which is a simple text file that lists the resources the browser should cache for offline access. This file should be referenced in the HTML file using the manifest attribute in the html tag. Once the manifest file is linked, the browser will automatically cache the resources listed in the file.
Can I control what gets stored in the ApplicationCache?
Yes, you have full control over what gets stored in the ApplicationCache. In the cache manifest file, you can specify which files should be cached, which files require a network connection, and which files should be used as fallbacks if the original file is not available.
How do I update the files stored in the ApplicationCache?
To update the files stored in the ApplicationCache, you need to make a change to the cache manifest file. This could be as simple as adding a comment. When the browser detects a change in the manifest file, it will re-download all the files listed in it.
What happens if a file listed in the manifest file is not available?
If a file listed in the manifest file is not available, the browser will use the fallback file specified in the manifest file. If no fallback file is specified, the browser will display an error.
Can all browsers use ApplicationCache?
Most modern browsers support ApplicationCache, including Chrome, Firefox, Safari, and Internet Explorer 10 and above. However, it’s always a good idea to check the browser compatibility before implementing this feature.
Is there a limit to how much data can be stored in the ApplicationCache?
Yes, browsers typically limit the amount of data that can be stored in the ApplicationCache. The exact limit varies by browser, but it’s usually around 5MB.
Can I use ApplicationCache for dynamic content?
Yes, you can use ApplicationCache for dynamic content. However, keep in mind that the content will only update when the cache manifest file changes. So, if your content updates frequently, you’ll need to update the manifest file regularly as well.
What are the benefits of using ApplicationCache?
Using ApplicationCache can improve the performance of your web application by reducing server load and saving bandwidth. It also allows users to access your application even when they’re offline, which can improve user experience.
Are there any downsides to using ApplicationCache?
One downside to using ApplicationCache is that it can be complex to set up and manage, especially for large applications. Also, because the content is stored locally, there may be security implications to consider.
Malcolm Sheridan is a Microsoft awarded MVP in ASP.NET, ASPInsider, Telerik Insider and a regular presenter at conferences and user groups throughout Australia and New Zealand. Follow him on twitter @malcolmsheridan.