Use JavaScript to replace all characters with asterisks.
//iVal is your string of characters (with asterisks).
iVal = iVal.substr(i).replace(/[S]/g, "*");
Frequently Asked Questions (FAQs) about jQuery Replacing Characters with Asterisks
How can I replace all characters in a string with asterisks using jQuery?
To replace all characters in a string with asterisks using jQuery, you can use the JavaScript replace() method in combination with a regular expression. Here’s an example:var str = "Hello World";
var newStr = str.replace(/./g, '*');
console.log(newStr); // **********
In this code, the regular expression /./g matches any character (except newline), and the ‘g’ flag ensures that all occurrences are replaced.
Can I replace only specific characters with asterisks?
Yes, you can replace only specific characters with asterisks. For instance, if you want to replace all ‘a’ characters in a string, you can modify the regular expression like this:var str = "Hello World";
var newStr = str.replace(/a/g, '*');
console.log(newStr); // Hello World (no change because 'a' is not present)
How can I replace all letters in a word with asterisks, but leave numbers and special characters?
To replace all letters in a string but leave numbers and special characters, you can use the regular expression /[a-zA-Z]/g. This matches any uppercase or lowercase letter. Here’s an example:var str = "Hello123!";
var newStr = str.replace(/[a-zA-Z]/g, '*');
console.log(newStr); // *****123!
Can I replace characters in a string without using regular expressions?
Yes, you can replace characters in a string without using regular expressions by using a loop to iterate over each character in the string. However, this method is less efficient and more complex than using regular expressions.
How can I replace characters in a string with asterisks in a case-insensitive manner?
To replace characters in a string with asterisks in a case-insensitive manner, you can use the ‘i’ flag with your regular expression. For example, to replace all ‘a’ characters regardless of case, you can do:var str = "Hello World";
var newStr = str.replace(/a/gi, '*');
console.log(newStr); // Hello World (no change because 'a' is not present)
Can I replace multiple different characters with asterisks at once?
Yes, you can replace multiple different characters with asterisks at once by including all the characters you want to replace inside square brackets in your regular expression. For example, to replace all ‘a’, ‘b’, and ‘c’ characters, you can do:var str = "abc123";
var newStr = str.replace(/[abc]/g, '*');
console.log(newStr); // ***123
How can I replace all non-alphanumeric characters with asterisks?
To replace all non-alphanumeric characters with asterisks, you can use the regular expression /\W/g. This matches any character that is not a letter or a number. Here’s an example:var str = "Hello, World!";
var newStr = str.replace(/\W/g, '*');
console.log(newStr); // Hello**World*
Can I replace characters in a string with asterisks using jQuery’s .text() or .html() methods?
No, jQuery’s .text() and .html() methods cannot be used to replace characters in a string with asterisks. These methods are used to get or set the text or HTML content of selected elements, not to manipulate strings.
How can I replace characters in a string with asterisks in a case-sensitive manner?
To replace characters in a string with asterisks in a case-sensitive manner, simply do not use the ‘i’ flag with your regular expression. For example, to replace all ‘a’ characters in a case-sensitive manner, you can do:var str = "Hello World";
var newStr = str.replace(/a/g, '*');
console.log(newStr); // Hello World (no change because 'a' is not present)
Can I replace characters in a string with asterisks using jQuery’s .replaceWith() method?
No, jQuery’s .replaceWith() method cannot be used to replace characters in a string with asterisks. This method is used to replace selected elements with new content, not to manipulate strings.
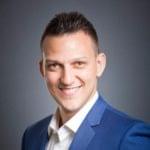
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.