jQuery code snippet to apply Drag/Touch Support for the iPad and devices with touch support. Could be particularly useful with jQuery UI draggable and floating objects.
//iPAD Support
$.fn.addTouch = function(){
this.each(function(i,el){
$(el).bind('touchstart touchmove touchend touchcancel',function(){
//we pass the original event object because the jQuery event
//object is normalized to w3c specs and does not provide the TouchList
handleTouch(event);
});
});
var handleTouch = function(event)
{
var touches = event.changedTouches,
first = touches[0],
type = '';
switch(event.type)
{
case 'touchstart':
type = 'mousedown';
break;
case 'touchmove':
type = 'mousemove';
event.preventDefault();
break;
case 'touchend':
type = 'mouseup';
break;
default:
return;
}
var simulatedEvent = document.createEvent('MouseEvent');
simulatedEvent.initMouseEvent(type, true, true, window, 1, first.screenX, first.screenY, first.clientX, first.clientY, false, false, false, false, 0/*left*/, null);
first.target.dispatchEvent(simulatedEvent);
};
};
Frequently Asked Questions (FAQs) about jQuery and iPad Touch Support
How can I add drag/touch support to my iPad using jQuery?
Adding drag/touch support to your iPad using jQuery involves using the jQuery UI Touch Punch. This is a small hack that enables the use of touch events on sites using the jQuery UI user interface library. To use it, you need to include the jQuery UI Touch Punch script after the jQuery UI and before its dependent scripts.
Can I use jQuery to recognize touch events in Safari for iPad?
Yes, you can use jQuery to recognize touch events in Safari for iPad. jQuery supports a set of touch events which you can use to build a touch-enabled web application. These events include touchstart, touchmove, touchend, and touchcancel.
How can I save an HTML table edited with jQuery to local storage?
You can save an HTML table edited with jQuery to local storage by converting the table data into a JSON object and then storing it in the local storage. You can use the localStorage.setItem()
method to store the data and localStorage.getItem()
to retrieve it.
What is the role of jQuery in the Shiny Things technology stack?
In the Shiny Things technology stack, jQuery is used as a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers.
How can I use the jQuery.touch plugin?
The jQuery.touch plugin is a jQuery plugin for touch devices. It adds touch events and CSS3 animation capabilities to jQuery. To use it, you need to include the jQuery.touch.js script in your HTML file after the jQuery script.
How can I troubleshoot issues with jQuery on Ubuntu?
Troubleshooting issues with jQuery on Ubuntu can be done by checking the console for any error messages, ensuring that the jQuery script is properly included in your HTML file, and making sure that your jQuery code is correct and does not contain any syntax errors.
Is it possible to use jQuery to create a touch-enabled slider?
Yes, it is possible to use jQuery to create a touch-enabled slider. You can use the jQuery UI Touch Punch to add touch event support to your slider. This will allow users to navigate through the slider using touch gestures.
How can I optimize my jQuery code for better performance on touch devices?
Optimizing your jQuery code for better performance on touch devices can be done by minimizing the use of heavy animations, reducing the number of DOM manipulations, and using event delegation to handle events efficiently.
Can I use jQuery to detect the type of touch event on an iPad?
Yes, you can use jQuery to detect the type of touch event on an iPad. jQuery supports a set of touch events which you can use to determine the type of touch event that occurred.
How can I use jQuery to handle multi-touch gestures on an iPad?
Handling multi-touch gestures on an iPad using jQuery can be a bit complex as it involves tracking multiple touch points. However, there are plugins available like jQuery MultiSwipe that can simplify this process.
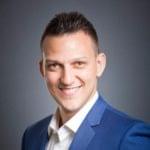
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.