Is it possible to declare arrays in JavaScript object literal notation?
Example 1 – this works with arrays
Declaration:NAMESPACE = {
data:
{
items: Array() //array
}
}
Storage of data:
NAMESPACE.data.items.push(data[0]);
Example 2 – this works with objects
Declaration:NAMESPACE = {
data:
{
items: {} //object
}
}
Storage of data:
NAMESPACE.data.items[data['key']] = data;
Please share your thoughts on this in comments.
Frequently Asked Questions (FAQs) about JavaScript Object Literal Notation and Arrays
What is the difference between object literal notation and array literal notation in JavaScript?
In JavaScript, both object literal notation and array literal notation are ways to create objects and arrays respectively. Object literal notation uses curly braces {} and can include properties and methods. For example, var person = {firstName:”John”, lastName:”Doe”}; creates an object with two properties. On the other hand, array literal notation uses square brackets [] and can include any number of elements. For example, var fruits = [“apple”, “banana”, “cherry”]; creates an array with three elements.
When should I use object literal notation and when should I use array literal notation?
The choice between object literal notation and array literal notation depends on the data you are working with. If you have a list of items where order matters, use an array. If you have a set of properties that belong to an object, use an object literal. For example, if you are storing a list of student names, an array would be appropriate. But if you are storing information about a single student, like their name, age, and grade, an object would be more suitable.
Can I use array methods on objects created with object literal notation?
No, array methods are not available for objects created with object literal notation. Array methods like push(), pop(), shift(), unshift(), etc., are specifically designed to work with arrays. If you try to use these methods on an object, JavaScript will throw an error.
Can I include functions in an object created with object literal notation?
Yes, you can include functions in an object created with object literal notation. These functions are called methods. For example, var person = {firstName:”John”, lastName:”Doe”, fullName: function() {return this.firstName + ” ” + this.lastName;}}; Here, fullName is a method that returns the full name of the person.
How can I access elements in an array created with array literal notation?
You can access elements in an array created with array literal notation using their index. The index of an array starts from 0. For example, if you have an array var fruits = [“apple”, “banana”, “cherry”];, you can access the first element with fruits[0], the second element with fruits[1], and so on.
Can I mix data types in an array created with array literal notation?
Yes, you can mix data types in an array created with array literal notation. An array in JavaScript can hold any type of data, including numbers, strings, objects, and even other arrays. For example, var mixedArray = [1, “two”, {name: “three”}, [4, 5, 6]]; is a valid array in JavaScript.
Can I add properties to an object after it’s been created with object literal notation?
Yes, you can add properties to an object after it’s been created with object literal notation. You can do this by using the dot notation or the bracket notation. For example, if you have an object var person = {firstName:”John”, lastName:”Doe”};, you can add a new property age with person.age = 25; or person[“age”] = 25;.
Can I change the value of an element in an array after it’s been created with array literal notation?
Yes, you can change the value of an element in an array after it’s been created with array literal notation. You can do this by using the index of the element. For example, if you have an array var fruits = [“apple”, “banana”, “cherry”];, you can change the first element with fruits[0] = “pear”;.
Can I nest objects and arrays created with object and array literal notation?
Yes, you can nest objects and arrays created with object and array literal notation. This is often done when you want to represent complex data structures. For example, you can have an object that contains an array of objects, each representing a person with their own set of properties.
How can I iterate over elements in an array created with array literal notation?
You can iterate over elements in an array created with array literal notation using a for loop or the forEach() method. For example, if you have an array var fruits = [“apple”, “banana”, “cherry”];, you can print each element with for (var i = 0; i < fruits.length; i++) {console.log(fruits[i]);} or fruits.forEach(function(fruit) {console.log(fruit);});.
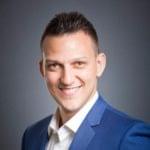
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.