It using a calculation of % interest which using logarithms to calculate the principle payment/total interest and how long it will take to pay off the loan given a borrow amount and a monthly payment amount
//FORMULA FOR CALUCLATING INTEREST RATE
//p = x*(1 - (1+r)^-n)/r
var interest = 15, rate = interest/100, principal = 1000, payment = 100, noofpay = 12;
var nper1 = Math.log((1-((principal/payment) * (rate/noofpay))));
var nper2 = Math.log((1+(rate/noofpay)));
nper = -(nper1/nper2);
interestpaid=payment*nper-principal;
nper = -Math.round((nper1/nper2));
nyear=Math.floor(nper/12);
nmonth=nper%12;
if (nper>0)
{
if (nmonth==0)
{
period=nyear+" Year(s)";
}
else
{
period=nyear+" Year(s) and "+nmonth+" Month(s)";
}
}
else
{
period="Invalid Values";
interestpaid=0;
}
console.log("Montly Payments: " + period + ", Total Interest Paid: " + interestpaid.toFixed(2));
Into a function with parameters passed in:
//the price calculation formula
//@return the price and length of time
function calculate(interest, principal, payment)
{
//get data
var calcElem = $('#calc');
//FORMULA FOR CALUCLATING INTEREST RATE
//p = x*(1 - (1+r)^-n)/r
var rate = interest/100, noofpay = 12;
var nper1 = Math.log((1-((principal/payment) * (rate/noofpay))));
var nper2 = Math.log((1+(rate/noofpay)));
nper = -(nper1/nper2);
interestpaid=payment*nper-principal;
nper = -Math.round((nper1/nper2));
nyear=Math.floor(nper/12);
nmonth=nper%12;
if (nper>0)
{
if (nmonth==0)
{
period=nyear+" Year(s)";
}
else
{
period=nyear+" Year(s) and "+nmonth+" Month(s)";
}
}
else
{
period="Invalid Values";
interestpaid=0;
}
//console.log("Montly Payments: " + period + ", Total Interest Paid: " + interestpaid.toFixed(2));
var priceData = {
price : '$'+this.formatCurrency(interestpaid+owe),
interest : '$'+this.formatCurrency(interestpaid),
time : period
}
return priceData;
}
Frequently Asked Questions (FAQs) about JavaScript Interest Loan Calculator Algorithm
How can I implement a loan calculator using JavaScript?
Implementing a loan calculator using JavaScript involves creating a form for user input, writing a function to calculate the loan, and displaying the results. The function should take into account the loan amount, interest rate, and loan term. It should use the formula for calculating loan payments, which is P = r(PV) / 1 – (1 + r)^-n, where P is the monthly payment, r is the monthly interest rate, PV is the present value or loan amount, and n is the number of payments.
How can I add a compound interest feature to my loan calculator?
To add a compound interest feature to your loan calculator, you need to modify the calculation function. Compound interest is calculated using the formula A = P (1 + r/n)^(nt), where A is the amount of money accumulated after n years, including interest, P is the principal amount, r is the annual interest rate, n is the number of times that interest is compounded per year, and t is the time the money is invested for in years.
How can I design the user interface for my loan calculator?
Designing the user interface for your loan calculator involves creating a form for user input and a space to display the results. You can use HTML and CSS for this. The form should include fields for the loan amount, interest rate, and loan term. The results should be displayed in a clear and easy-to-understand format.
How can I validate user input in my loan calculator?
You can validate user input in your loan calculator using JavaScript. You should check that the loan amount, interest rate, and loan term are all positive numbers. If any of these values are not valid, you should display an error message to the user.
How can I handle errors in my loan calculator?
You can handle errors in your loan calculator by using try-catch blocks in your JavaScript code. If an error occurs during the calculation, you can catch it and display an appropriate error message to the user.
How can I add a feature to calculate the total interest paid over the loan term?
To add a feature to calculate the total interest paid over the loan term, you need to modify the calculation function. The total interest paid is the total payments made minus the loan amount. You can calculate the total payments by multiplying the monthly payment by the number of payments.
How can I add a feature to calculate the total payments made over the loan term?
To add a feature to calculate the total payments made over the loan term, you need to modify the calculation function. The total payments made is the monthly payment multiplied by the number of payments.
How can I add a feature to calculate the monthly payment?
To add a feature to calculate the monthly payment, you need to modify the calculation function. The monthly payment is calculated using the formula P = r(PV) / 1 – (1 + r)^-n.
How can I add a feature to calculate the loan term?
To add a feature to calculate the loan term, you need to modify the calculation function. The loan term is the number of payments divided by the number of payments per year.
How can I add a feature to calculate the interest rate?
To add a feature to calculate the interest rate, you need to modify the calculation function. The interest rate is the annual interest rate divided by the number of payments per year.
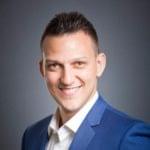
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.