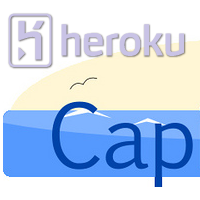
Deploying to the Cloud
We’ll choose Heroku as our cloud option, since it is very popular for Rack and Rails app deployments. You deploy to Heroku by pushing your changes to the Git remote that Heroku provide for you when you first create the app on their service. It should feel familiar for PHP coders transferring to ruby, especially if you have used a cloud platform like phpfog for example. There are several steps involved in getting your Rails app up and running on Heroku:- Develop your app (doh!)
- Sign up for Heroku if you haven’t already
- Initialise Git for your project
- Create a Heroku app
- Add the Git remote for Heroku (you might already have a Github remote repo for example)
- Push your app to Heroku
- Migrate the database (if you are using one)
Choosing a Database
Heroku doesn’t support Sqlite, so if your app uses it, Heroku will complain. Heroku uses the excellent Postgresql. During the initial deployment of your app, you can run your migrations to populate your Heroku Postgresql database. If you have moved to developing with Rails from PHP, you’ll be used to MySQL. In which case, you have nothing to fear from Postgresql. It has gained in popularity with Rails developers recently, so it is well worth getting used to. If you are still considering using Sqlite, this article on the Heroku dev center should put things into perspective for you.Making a Start…
So, your app is at a point where it can be pushed to Heroku. Turning it into a Heroku app is just a case of getting on the command line/terminal. Change directory to your project, and make sure you have committed all your latest changes. Then simply do:heroku create
The next thing that will happen is Heroku will respond by telling you your app has been created. It is highly likely that it will have an odd name (infinite-escarpment-4759 for example), but we can change that shortly. You will also be told what your Git remote url is for Heroku. The nice thing about that is, you can push your changes to Github for example, and then, when you are ready to deploy, you can push to your Heroku branch.
Changing the App Name
Assuming you want this app to have a real purpose rather than just for testing, you’ll want to use a sensible name for it. Back on the command line do:heroku apps:rename new_name_here
The nice thing about this is that your Git remote will automatically be updated. If you use the Heroku web site to rename your app, you’ll need to remove the old Heroku remote and add the new one:
git remote rm heroku git remote add heroku git@heroku.com:newname.git
If you have a domain name that you want to use for your app, return to the command line and do:
heroku domains:add www.mydomain.com
Next, we’ll deploy.
Deploying the App
All you need to do is: git push heroku master
You’ll see Heroku run through a whole bunch of processes, including rake assets:precompile
which is really nice. When it finishes, you should see something like: http://yourapp.herokuapp.com deployed to Heroku
. Great! Oh, wait a minute though…
The Database
You can also deploy your migrations. Back on the command line do:heroku run rake db:migrate
you might see a deprecation warning about the plugins in vendor/plugins. You can safely ignore the warning if you know you haven’t done anything with plugins.
That’s All Folks…
Really. That’s it, your app should now be up and running. The only problem with Heroku is: the free version is a great service. However, if you need to add more resources, it can get quite expensive. Don’t say I didn’t warn you…Deploying to a Virtual Private Server (VPS)
The other option is to buy yourself a VPS, and deploy your app to that. The one I’m using here is called Linode. The first thing I should point out is that, if setting up servers doesn’t appeal to you, then choose Heroku. When you sign up with Linode, you can choose the (Linux) server operating system. They have Ubuntu, Fedora, OpenSUSE etc. so your favourite will probably be supported. It is also possible to create and tear down if you go wrong. In fact, it’s encouraged on Linode. So if you are a Linux n00b, there is plenty of help and support. When your server is set up it is a raw, basic server installation. That means, to get a Rails app up and running you will need to do some installing first. Oh, and in case you were wondering, no, there isn’t a GUI. It’s command line all the way.The Production Stack
You have options here. I ended up going with Nginx for a web server, PostgreSQL for database stuff, and rbenv to manage Ruby versions. You could just as easily choose Apache, Phusion Passenger, and MySQL. I made these choices because I had already tinkered with Phusion Passenger and RVM, and wanted to try the other options. Once you have your Linode set up, login in via SSH. Your Linode dashboard has all the information you need to do that:ssh root@176.xxx.xxx.xxx
You should run a distro update and install any that are required first. Then install the production stack components. If you are using ubuntu install the software properties option to make it easy to add new repositories to apt:
apt-get -y install curl git-core python-software-properties
Then you can add the stable branch for Nginx:
add-apt-repository ppa:nginx/stable
Regardless of your distro you need to install:
- Nginx or Apache
- MySQL or PostgreSQL
- Also install node.js while you are at it, so that you have a way to execute Javascript.
- You will need to install Git and Curl too.
curl -L https://raw.github.com/fesplugas/rbenv-installer/master/bin/rbenv-installer | bash
You’ll be told to copy some lines into your .bashrc file, so make sure that you do. Where you copy them is a bit of a gotcha too. The lines need to go above this line in your .bashrc file:
# If not running interactively, don't do anything [ -z "$PS1" ] && return
This is so that rbenv will work with Capistrano deployments, which we’ll get to shortly. You’ll need to reload your .bashrc file now: ~/.bashrc
. At this point the rbenv command will be available on the command line, and can be used to install some important dependencies for Ruby. For example, for Ubuntu 12.04 you can do:
rbenv bootstrap-ubuntu-12-04
Then wait for a while as the dependancies are installed. Once that is done, you can install Ruby:
rbenv install 1.9.3-p125
And then set it as the default Ruby:
rbenv global 1.9.3-p125
Finally, install the bundler gem so that we have the required access to the bundle executable:
gem install bundler --no-ri --no-rdoc
Use the rbenv rehash command to pick up the changes:
rbenv rehash
Getting the Application Ready
Assuming that you will manage your source code through Github, it’s a good idea to add an ssh connection to it from our server. While still logged in, do:ssh git@github.com
. This will ensure that when we deploy via Capistrano, we shouldn’t get any “unknown” errors.
The idea is, that before we deploy via Capistrano we can check that everything is committed to Github first.Now you can develop and push to github as normal; your Linode server is nearly ready to recieve the deployed app. First though, we need to set up Unicorn and Capistrano.
Unicorn and Capistrano
Unicorn is a HTTP server that works nicely with Nginx for running Rack applications. Capistrano is one of the many ways to deploy a Rails app in a semi-automated way. We will need to add both of them to our gemfile:gem 'unicorn' gem 'capistrano'
Back on the command line, in your application directory run the capify
command. That will create a Capfile and a config/deploy.rb file, both of which, will need to be edited.
Starting with the capfile, we are using the asset pipeline so we’ll uncomment accordingly:
load 'deploy' # Uncomment if you are using Rails' asset pipeline load 'deploy/assets' Dir['vendor/gems/*/recipes/*.rb','vendor/plugins/*/recipes/*.rb'].each { |plugin| load(plugin) } load 'config/deploy' # remove this line to skip loading any of the default tasks
Then, we can paste in our Capistrano recipe:
[gist id=”3627252″]
It’s beyond the scope of this article to go through the file in detail. However, you can see that there is a section for setting up roles for the web app and the database, and also, the directory that the app will be deployed to.
Our Github credentials are included, and there is a section for making sure all local changes have been committed before the deployment runs.
We also need to add a config file for Nginx in: config/nginx.conf
:
[gist id=”3627362″]
This is a standard config file for Nginx that maps to the location of your app. You can find sample nginx config files here.
You will also need to add a config file for configuring Unicorn:
[gist id=”3627458″]
You can see a sample file here, but the file basically sets paths and the number of worker processes. This file also goes in your app’s config directory.
The final config file we need to add to our application is a shell script to start Unicorn:
[gist id=”3627518″]
Again this goes in your app’s config directory. It is a fairly generic script with variables that will need to be adjusted at the top of the file. This script will also need to be marked as executable.
It is a good idea to set up another user on your VPS, so that user’s credentials are used rather than root, for the user that appears in the various config files.You should commit the config files to your Git repository too.
Running the Deployment
Now you can run:cap deploy:setup
to begin deploying the application. the command will log into the server using the user account you set up. It will also create the various directories specified in your config files.
You should now be able to run: cap deploy:cold
. The ‘cold’ option means the database migrations will also be run. You may see errors at this point, but the output will give you an indication as to where the problem is so it can be fixed.
Fixing Nginx Default Site
When you visit your server’s IP address, you’ll still see the default Nginx page. Log in to your server via SSH, and remove the symbolic link:sudo rm /etc/nginx/sites-enabled/default
Then restart Nginx (this is for Ubuntu, your distro’s documentation will help you with this):
sudo service nginx restart
One More Thing…
Run the following command so that Unicorn starts properly when the server is restarted:sudo update-rc.d unicorn_appname defaults
You should now be able to browse to your site and see your app running.
Finally…
The fastest and most pain free way to deploy a Rails app is via Heroku, there is no doubt about that. However, it can get expensive, and you don’t have finite control over your application. For as little as $20 per month, you can have a full featured, virtual private server to configure your own way and with maximum control over the apps you deploy. It is a much more involved process though.Frequently Asked Questions about Deploying a Rails Application
What are the prerequisites for deploying a Rails application?
Before deploying a Rails application, you need to have a basic understanding of Ruby on Rails and its MVC architecture. You should also be familiar with the command line, Git, and GitHub. It’s also important to have a Rails application ready for deployment. If you’re deploying to a specific platform, you may need to meet additional requirements. For example, if you’re deploying to Heroku, you’ll need a Heroku account and the Heroku CLI installed on your machine.
How do I choose the right server for my Rails application?
Choosing the right server for your Rails application depends on several factors including your application’s requirements, your budget, and your technical expertise. Some popular options include Heroku, AWS, and DigitalOcean. Heroku is user-friendly and great for beginners, but it can be expensive for larger applications. AWS and DigitalOcean offer more control and scalability, but they require more setup and maintenance.
How do I prepare my Rails application for deployment?
Preparing your Rails application for deployment involves several steps. First, you need to ensure that your application is in a production-ready state. This means fixing any bugs, optimizing your code, and testing your application thoroughly. Next, you need to configure your application for the production environment. This may involve setting environment variables, configuring your database, and precompiling your assets. Finally, you need to version control your application using Git and push your code to a repository on GitHub or another platform.
How do I deploy my Rails application to Heroku?
Deploying your Rails application to Heroku involves several steps. First, you need to create a new Heroku application using the Heroku CLI. Next, you need to push your code to Heroku using Git. Then, you need to migrate your database using the heroku run rake db:migrate
command. Finally, you need to restart your Heroku application and open it in your web browser.
How do I troubleshoot common deployment issues?
Troubleshooting deployment issues can be challenging, but there are several strategies you can use. First, check the error messages in your server logs. These can often provide clues about what’s going wrong. If you’re using Heroku, you can view your logs using the heroku logs
command. If you’re having database issues, make sure your database is properly configured for the production environment. If you’re having issues with assets, make sure your assets are being precompiled correctly.
How do I update my deployed Rails application?
Updating your deployed Rails application involves pushing your updated code to your server and restarting your application. If you’ve made changes to your database schema, you’ll also need to migrate your database. If you’re using Heroku, you can do this using the heroku run rake db:migrate
command.
How do I secure my Rails application for deployment?
Securing your Rails application for deployment involves several steps. First, you should ensure that your application is running the latest version of Rails and all other dependencies to benefit from the latest security patches. You should also configure your application to use HTTPS, which encrypts data in transit between your application and its users. Additionally, you should use secure passwords and API keys, and store them securely using environment variables or a secure key management service.
How do I scale my Rails application after deployment?
Scaling your Rails application after deployment can involve several strategies, depending on your application’s needs and your server’s capabilities. You might need to add more dynos or workers if your application is receiving more traffic than it can handle. You might also need to upgrade your database or use a content delivery network (CDN) to serve your assets more quickly. Additionally, you might need to optimize your code or use caching to improve your application’s performance.
How do I monitor my deployed Rails application?
Monitoring your deployed Rails application is crucial for maintaining its performance and availability. You can use tools like New Relic or Datadog to monitor your application’s performance, track errors, and receive alerts when issues arise. You can also use logging services like Logentries or Papertrail to keep track of your application’s logs.
How do I automate the deployment process for my Rails application?
Automating the deployment process for your Rails application can save you time and reduce the risk of errors. You can use continuous integration and continuous deployment (CI/CD) tools like Jenkins, Travis CI, or CircleCI to automatically build, test, and deploy your application whenever you push changes to your code. You can also use configuration management tools like Ansible, Chef, or Puppet to automate the setup and configuration of your server.
Andy Hawthorne is from Coventry in the UK. He is a senior PHP developer by day, and a freelance writer by night, although lately that is sometimes the other way around.