This is the third in a series of four posts over four weeks that will show you how to create a simple Windows 8 game, using HTML5, JavaScript, WinJS, and CreateJS. The game is based on the XNA sample game “Catapult Wars Lab”. We’ll reuse the assets from that game as we develop a new version for Windows 8 that’s based on web technologies. In this post, we’ll bring things to life with some game logic and JavaScript.
The Game Loop
The heartbeat of any game is the game loop. It’s a function that runs many times per second and has two primary jobs – update what’s going on then draw the new scene. In Part 2, we already put the outline in place: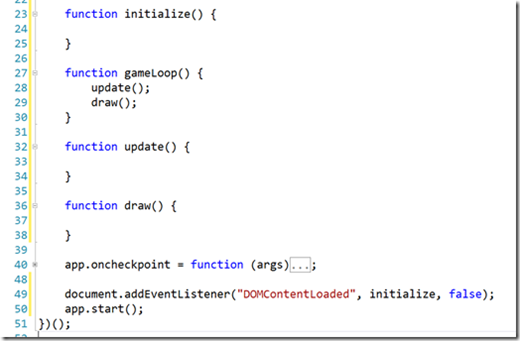
gameLoop()
function started, and keep it running many times per second?
EaselJS Ticker Class
Fortunately, EaselJS has a Ticker class that has some features we’ll use:- Manage the timing of the game loop
- Pause/resume the game loop
- Measure elapsed time
default.js
, add a new startGame()
function and call it at the end of prepareGame()
:
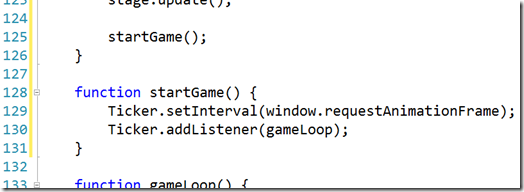
window.requestAnimationFrame
to control how frequently the gameLoop function is called.
requestAnimationFrame
is a relatively new API for web applications that helps ensure work isn’t being done unnecessarily. To understand why this can be better than setting a fixed timer (e.g. with setTimeout()
), see the requestAnimationFrame sample on the IE Test Drive site.
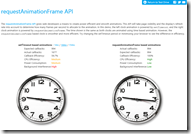
requestAnimationFrame
is ready, our game loop will run.
“Ready, Aim, Fire!”
Okay, now we have a game with a running game loop, so it’s time to add some fun! Each player/catapult is going to be firing the ammo/rock toward the other. We need to know if a shot is currently flying, who’s turn it is, and how the shot is moving. First, let’s add more variables todefault.js
:
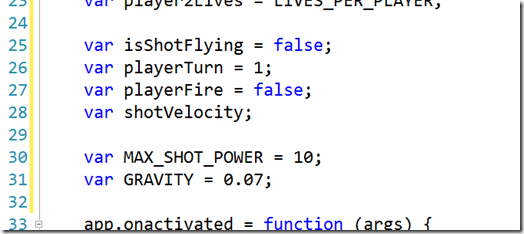
update()
function:
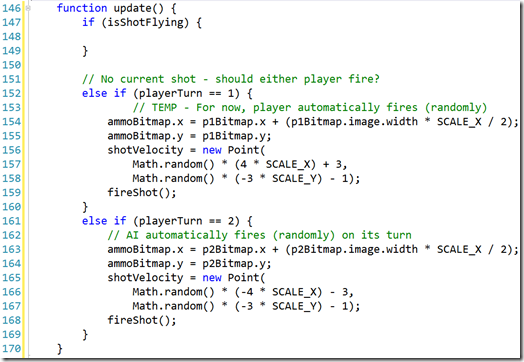
ammoBitma
p is moved to the top center of the firing catapult, and shotVelocity
is given a random value within a bounded range (adjusted for screen resolution.)
We’ll also add a fireShot()
function to show the shot and tell the game it’s in the air:
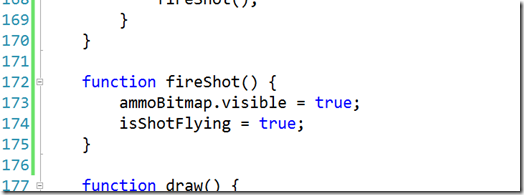
Updating the Display
Before we move the shot through the air, let’s focus on the 2nd half of the game loop equation – drawing to the screen. This can often be very complex, but EaselJS Stage takes care of drawing our content (all of the children – Bitmaps, Text, etc. – we added to Stage) to the canvas, so this is all we need: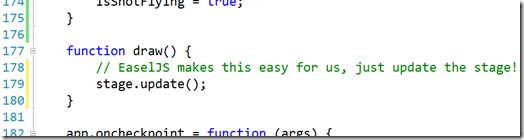
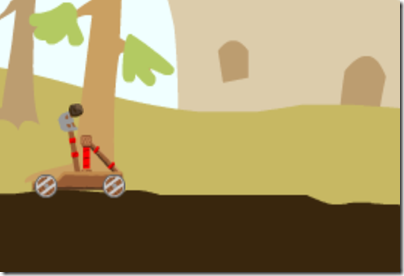
Moving the Shot
Let’s return to theupdate()
function and add logic to the if (shotIsFlying)
statement:
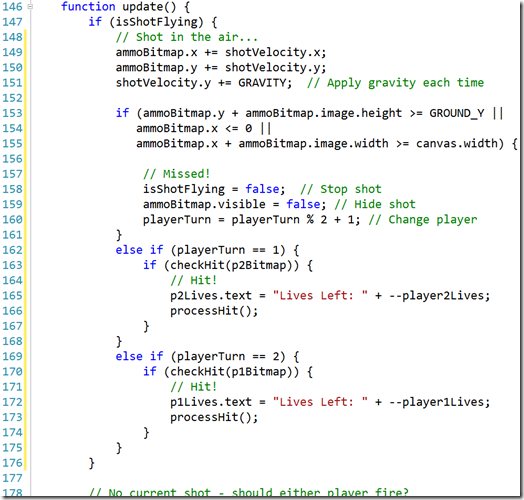
- Lines 149 & 150 – Move the shot by adding velocity (which may be negative to go up and/or left)
- Line 151 – Apply gravity to slow velocity
- Lines 153-155 – Has the shot hit the ground or gone of the left or right edge of the screen?
- Lines 157-160 – Missed – end the shot and change players
- Lines 162-168 – Player 1’s shot – see if it hit player 2. If so, update player 2’s lives.
- Lines 169-175 – Player 2’s shot – see if it hit player 1. If so, update player 1’s lives.
checkHit(Bitmap)
function:
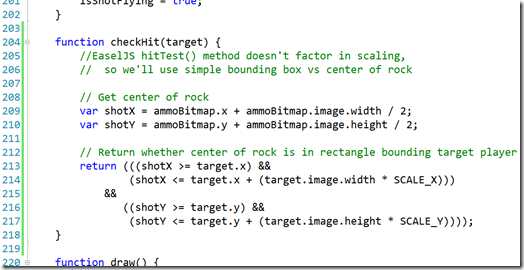
Bitmap
is based) supports a hitTest()
method, which makes it very easy to see if a point is over the current object’s position. Unfortunately, we’re scaling objects and hitTest only works with the original sizes, so we’ll need to check for hits ourselves. Just a bit of math and we’re set.
Handling Hits
Now, add the processHit() function: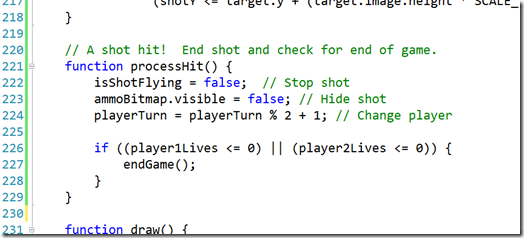
Ending the Game
Let’s end this post by ending the game. Add theendgame(Image)
function:
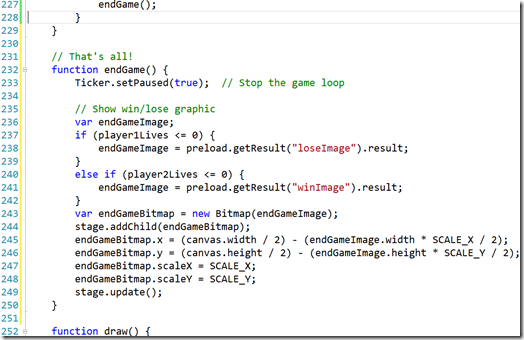
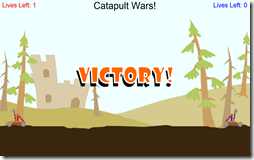
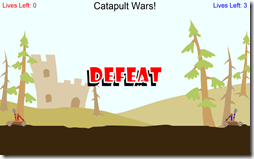
What’s Next?
We’ve added a lot in this part – things are drawing, moving, hitting, ending… but there are two gaping holes. First, the player doesn’t actually play, so we’ll add input processing next. Second, things are awfully quiet, especially for a war, so we’ll toss in some sounds as well. On to part 4: next week!Frequently Asked Questions about Creating a Simple Windows 8 Game with JavaScript
How can I start creating a game with JavaScript for Windows 8?
To start creating a game with JavaScript for Windows 8, you need to have a basic understanding of JavaScript and HTML5. You will also need to install Visual Studio, which is the development environment for Windows 8 applications. Once you have these prerequisites, you can start by creating a new project in Visual Studio and selecting the “Blank App” template. From there, you can start writing your game logic in JavaScript and designing your game interface with HTML5 and CSS.
What are some examples of games I can create with JavaScript?
JavaScript is a versatile programming language that can be used to create a wide variety of games. Some examples include puzzle games, strategy games, platformers, and even 3D games. The possibilities are endless, and with the right tools and resources, you can create any type of game you can imagine.
How can I add interactivity to my JavaScript game?
Interactivity in a JavaScript game can be achieved through event handlers. These are functions that are triggered when a specific event occurs, such as a mouse click or a key press. For example, you can use the ‘onclick’ event to trigger a function when the user clicks on a game element, or the ‘onkeydown’ event to move a character when the user presses a key.
How can I test and debug my JavaScript game?
Testing and debugging are crucial parts of game development. Visual Studio provides a powerful debugging tool that allows you to step through your code, inspect variables, and identify any errors or bugs. You can also use the console to log messages and track the execution of your code.
How can I optimize my JavaScript game for performance?
Optimizing a JavaScript game for performance involves a number of techniques. These include minimizing the use of global variables, using requestAnimationFrame for game loops, optimizing your images and assets, and using efficient algorithms for game logic. It’s also important to test your game on a variety of devices and browsers to ensure it runs smoothly.
How can I add sound and music to my JavaScript game?
Sound and music can be added to a JavaScript game using the HTML5 Audio API. This allows you to load and play sound files, control the volume, and even create sound effects. You can also use libraries like Howler.js to simplify the process and add more advanced audio features.
How can I publish my JavaScript game on the Windows Store?
Once you’ve finished developing your game, you can publish it on the Windows Store by creating a Store account, registering your app, and submitting it for certification. You’ll need to provide screenshots, a description, and other information about your game. Once your game is approved, it will be available for download on the Windows Store.
Can I monetize my JavaScript game?
Yes, you can monetize your JavaScript game in several ways. One option is to sell your game on the Windows Store. You can also include in-app purchases, where players can buy additional content or features. Another option is to display ads in your game, although this should be done sparingly to avoid disrupting the gameplay experience.
Can I use JavaScript to create games for other platforms?
Yes, JavaScript is not limited to Windows 8 or any specific platform. With the right tools and libraries, you can create games that run on a variety of platforms, including web browsers, mobile devices, and even game consoles. Some popular libraries for cross-platform game development with JavaScript include Phaser, Pixi.js, and Three.js.
Where can I learn more about game development with JavaScript?
There are many resources available online for learning about game development with JavaScript. Websites like W3Schools, Mozilla Developer Network, and Codecademy offer tutorials and guides on JavaScript and game development. There are also many books, online courses, and forums where you can learn and get help from other developers.
Chris Bowen is a Principal Technical Evangelist with Microsoft, based in the Boston area and specializing in Windows 8 development. An architect and developer with over 19 years in the industry, he joined Microsoft after holding senior technical positions at companies including Monster.com, VistaPrint, and Staples. He is coauthor of two books (with Addison-Wesley and WROX) and holds an M.S. in Computer Science and a B.S. in Management Information Systems, both from Worcester Polytechnic Institute.