A social sharing plugin allows your website visitors to share your website content easily on social media sites. This helps to increase the overall awareness of your website.
There are already dozens of existing social sharing plugins that you can just install and be done with it, but where’s the fun in that?
In this tutorial, I’ll show you how to build your very own social sharing plugin for WordPress from scratch, which can add social sharing buttons below every post. Users can share the post simply by clicking on the desired social media site button.
Why Do You Need Social Media Share Buttons?
It’s often reported that more than 80% of users consider reading content based on their friends’ recommendations. With social sharing, you give users the ability to share your content with their own networks of friends.
More than 40 billion shares are clicked each day on the web, therefore adding social sharing buttons on your WordPress website is first step to helping to market your site.
Plugin Directory and Files
To kick things off, create a directory called social-share and create the following files in it:
--social-share
-social-share.php
-style.css
In the social-share.php file add the following text to make the plugin installable.
<?php
/*
Plugin Name: Social Share
Plugin URI: https://www.sitepoint.com
Description: Displays Social Share icons below every post
Version: 1.0
Author: Narayan Prusty
*/
Creating a Admin Menu Item
We need to create a options page for our plugin where user can select buttons for which social media sites should be displayed. For creating a options page first we need to create a menu item to which the options page will be attached to.
Here is the code to create a admin menu item under Settings top level menu item.
function social_share_menu_item()
{
add_submenu_page("options-general.php", "Social Share", "Social Share", "manage_options", "social-share", "social_share_page");
}
add_action("admin_menu", "social_share_menu_item");
Here we’re adding a menu item using add_submenu_page
which is indeed called inside the admin_menu
action. social_share_page
is the callback function which needs to display the contents of the options page.
Here is what our menu item looks like:
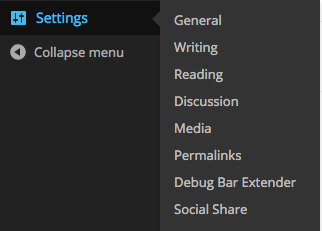
Creating an Options Page
Let’s code the social_share_page
function to display the options page content.
function social_share_page()
{
?>
<div class="wrap">
<h1>Social Sharing Options</h1>
<form method="post" action="options.php">
<?php
settings_fields("social_share_config_section");
do_settings_sections("social-share");
submit_button();
?>
</form>
</div>
<?php
}
Here we’re adding a section named social_share_config_section
, and registering the settings as social-share
.
Now lets display the section and its option fields.
function social_share_settings()
{
add_settings_section("social_share_config_section", "", null, "social-share");
add_settings_field("social-share-facebook", "Do you want to display Facebook share button?", "social_share_facebook_checkbox", "social-share", "social_share_config_section");
add_settings_field("social-share-twitter", "Do you want to display Twitter share button?", "social_share_twitter_checkbox", "social-share", "social_share_config_section");
add_settings_field("social-share-linkedin", "Do you want to display LinkedIn share button?", "social_share_linkedin_checkbox", "social-share", "social_share_config_section");
add_settings_field("social-share-reddit", "Do you want to display Reddit share button?", "social_share_reddit_checkbox", "social-share", "social_share_config_section");
register_setting("social_share_config_section", "social-share-facebook");
register_setting("social_share_config_section", "social-share-twitter");
register_setting("social_share_config_section", "social-share-linkedin");
register_setting("social_share_config_section", "social-share-reddit");
}
function social_share_facebook_checkbox()
{
?>
<input type="checkbox" name="social-share-facebook" value="1" <?php checked(1, get_option('social-share-facebook'), true); ?> /> Check for Yes
<?php
}
function social_share_twitter_checkbox()
{
?>
<input type="checkbox" name="social-share-twitter" value="1" <?php checked(1, get_option('social-share-twitter'), true); ?> /> Check for Yes
<?php
}
function social_share_linkedin_checkbox()
{
?>
<input type="checkbox" name="social-share-linkedin" value="1" <?php checked(1, get_option('social-share-linkedin'), true); ?> /> Check for Yes
<?php
}
function social_share_reddit_checkbox()
{
?>
<input type="checkbox" name="social-share-reddit" value="1" <?php checked(1, get_option('social-share-reddit'), true); ?> /> Check for Yes
<?php
}
add_action("admin_init", "social_share_settings");
Here we’re letting the user to choose from Facebook, Twitter, LinkedIn and Reddit sharing buttons. We are providing a checkbox interface to allow administrators to choose which buttons to display. You can expand the list to support more social media sites as needed.
Here is what our final options page looks like:
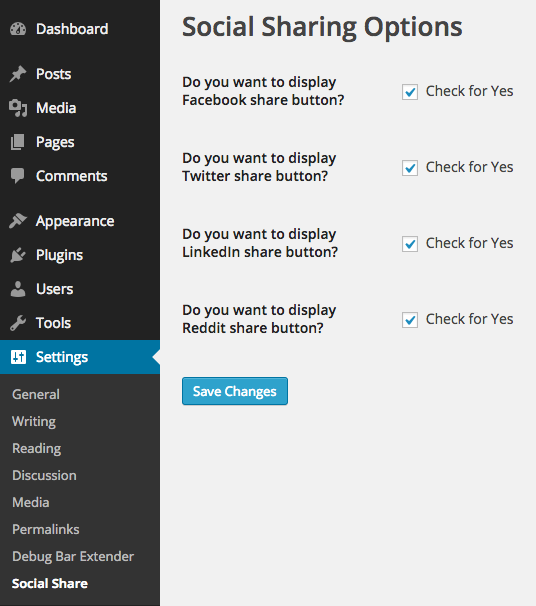
Displaying the Social Sharing Buttons
To display social sharing buttons below every post, we need to filter the content of every post before it’s sent out. We need to use the the_content
filter to add social sharing buttons to the end of the posts.
Here is code on how to filter post content and display social media buttons.
function add_social_share_icons($content)
{
$html = "<div class='social-share-wrapper'><div class='share-on'>Share on: </div>";
global $post;
$url = get_permalink($post->ID);
$url = esc_url($url);
if(get_option("social-share-facebook") == 1)
{
$html = $html . "<div class='facebook'><a target='_blank' href='http://www.facebook.com/sharer.php?u=" . $url . "'>Facebook</a></div>";
}
if(get_option("social-share-twitter") == 1)
{
$html = $html . "<div class='twitter'><a target='_blank' href='https://twitter.com/share?url=" . $url . "'>Twitter</a></div>";
}
if(get_option("social-share-linkedin") == 1)
{
$html = $html . "<div class='linkedin'><a target='_blank' href='http://www.linkedin.com/shareArticle?url=" . $url . "'>LinkedIn</a></div>";
}
if(get_option("social-share-reddit") == 1)
{
$html = $html . "<div class='reddit'><a target='_blank' href='http://reddit.com/submit?url=" . $url . "'>Reddit</a></div>";
}
$html = $html . "<div class='clear'></div></div>";
return $content = $content . $html;
}
add_filter("the_content", "add_social_share_icons");
Here’s how this code works:
- First we are adding a wrapper for our social media sharing links.
- Then, we are retrieving the complete URL of the current post which will be shared on the social media sites. We are also escaping the URL using WordPress provided
esc_url
function. - Then we are checking which buttons users want to display and add the respective button markup to the post content.
- Finally, we’re adding the current post URL to the end of the social sharing links of the respective social media sites.
Here is how our social media buttons looks on the front-end below every post:
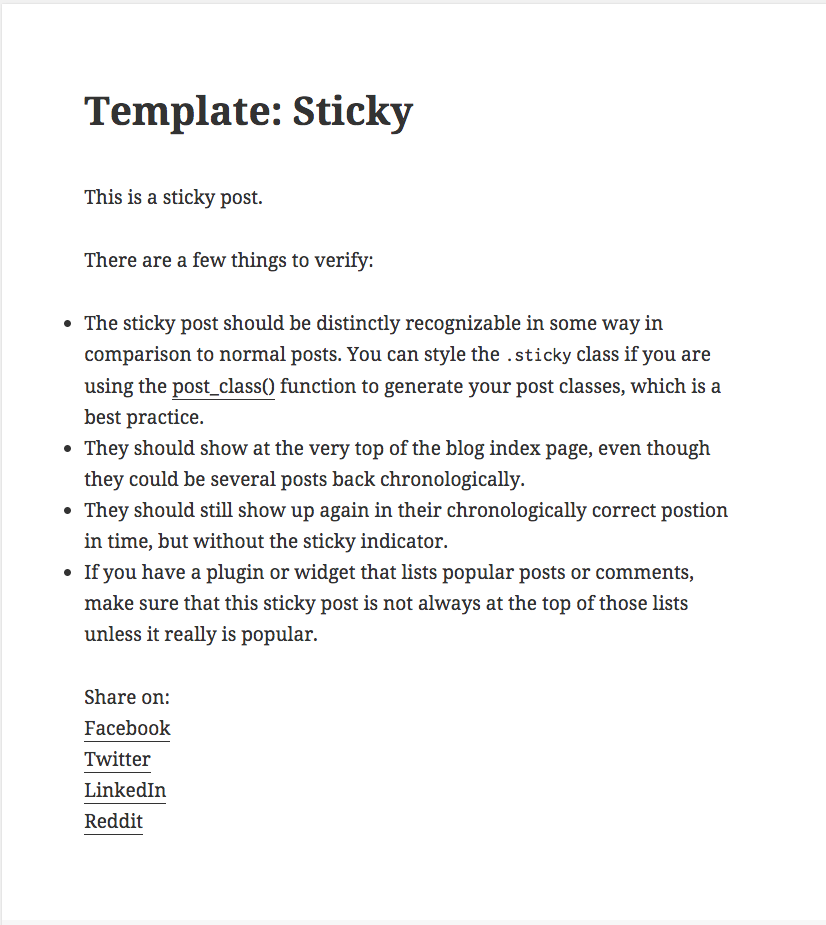
Styling the Social Media Buttons
Let’s attach style.css
on the front-end inside which we will place the code for styling the buttons. Here’s the code enqueue the style.css
file.
function social_share_style()
{
wp_register_style("social-share-style-file", plugin_dir_url(__FILE__) . "style.css");
wp_enqueue_style("social-share-style-file");
}
add_action("wp_enqueue_scripts", "social_share_style");
Here’s the CSS code for styling the buttons:
.social-share-wrapper div
{
float: left;
margin-right: 10px;
}
.social-share-wrapper div.share-on
{
padding: 5px;
font-weight: bold;
}
.social-share-wrapper div.facebook
{
background-color: #3a5795;
padding: 5px;
}
.social-share-wrapper div.facebook a
{
color: white;
}
.social-share-wrapper div.twitter
{
background-color: #55acee;
padding: 5px;
}
.social-share-wrapper div.twitter a
{
color: white;
}
.social-share-wrapper div.linkedin
{
background-color: #007bb6;
padding: 5px;
}
.social-share-wrapper div.linkedin a
{
color: white;
}
.social-share-wrapper div.reddit
{
background-color: #ACD4FC;
padding: 5px;
}
.social-share-wrapper div.reddit a
{
color: white;
}
.social-share-wrapper div a
{
text-decoration: none;
border: none;
}
.clear
{
clear: left;
}
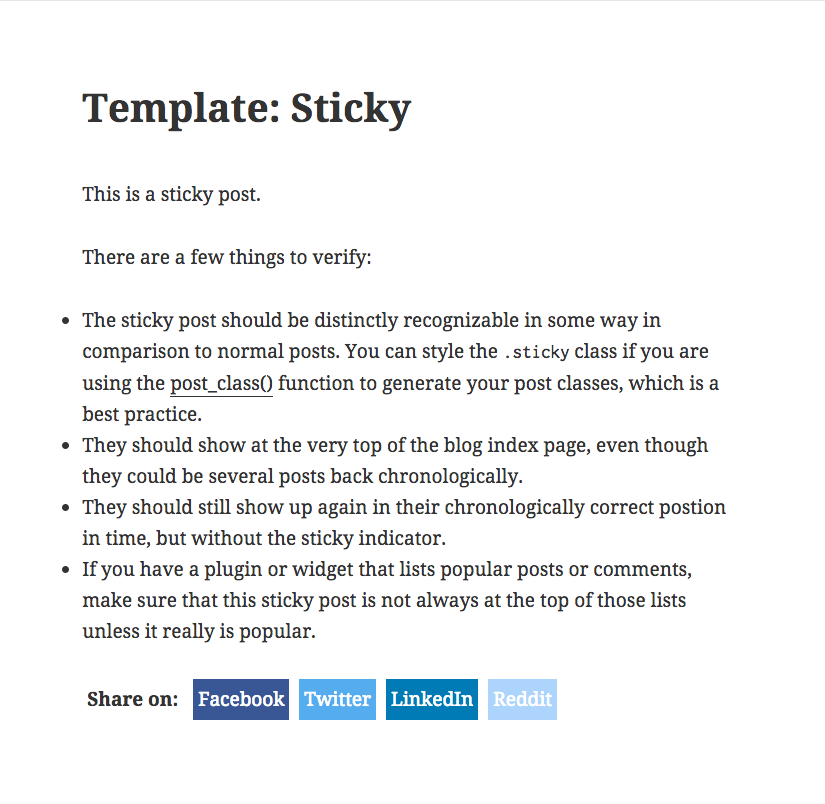
Conclusion
In this article I’ve shown you how to easily build your own a social media sharing plugin. You can now go ahead and expand on this to add buttons for more social media sites and also display the number of shares along with the buttons. Please share your experience with your own plugins below.
Frequently Asked Questions about Building Your Own Social Sharing Plugin for WordPress
How can I customize my social sharing plugin for WordPress?
Customizing your social sharing plugin for WordPress can be done by modifying the CSS styles. You can change the appearance of your buttons, their size, color, and even their hover effects. You can also decide where you want your buttons to appear on your website, whether it’s on the top, bottom, or sides of your posts. Remember to always test your changes to ensure they work as expected and don’t interfere with the functionality of your website.
Can I add more social media platforms to my plugin?
Yes, you can add more social media platforms to your plugin. This can be done by adding more button elements in your PHP code and linking them to the respective social media sharing URLs. Make sure to use the correct URL structure for each platform to ensure the sharing functionality works correctly.
How can I make my social sharing buttons responsive?
Making your social sharing buttons responsive involves using CSS media queries. These allow you to set different styles for different screen sizes, ensuring your buttons look good on all devices. You can specify different sizes, positions, and even different images for your buttons depending on the screen size.
Is it possible to track the performance of my social sharing buttons?
Yes, you can track the performance of your social sharing buttons by integrating them with analytics tools like Google Analytics. This can be done by adding tracking codes to your button links. This will allow you to see how many times each button is clicked and how much traffic they’re driving to your website.
How can I add a share count to my social sharing buttons?
Adding a share count to your social sharing buttons can be done by using the APIs provided by the social media platforms. These APIs allow you to retrieve the number of times a URL has been shared on their platform. You can then display this number next to your sharing buttons. Note that not all platforms provide this feature, and some may require you to register an application to use their API.
Can I add social sharing buttons to custom post types?
Yes, you can add social sharing buttons to custom post types. This can be done by modifying the PHP code that generates your buttons. You’ll need to add a condition that checks the post type and adds the buttons accordingly. Make sure to test your changes to ensure they work correctly.
How can I optimize my social sharing buttons for SEO?
Optimizing your social sharing buttons for SEO involves adding the appropriate meta tags to your pages. These tags provide information about your content to the social media platforms, such as the title, description, and image to display when your content is shared. This can improve the visibility and click-through rate of your shared content.
Can I use SVG icons for my social sharing buttons?
Yes, you can use SVG icons for your social sharing buttons. SVG icons are vector-based, meaning they can be scaled without losing quality. This makes them a great choice for responsive designs. You can either use pre-made SVG icons or create your own using graphic design software.
How can I add a social sharing button to my WordPress menu?
Adding a social sharing button to your WordPress menu can be done by using the WordPress menu editor. You can add a custom link to your menu and use CSS to style it as a button. Note that this will create a static link, not a dynamic one that changes based on the current page.
How can I make my social sharing buttons load faster?
Making your social sharing buttons load faster can be achieved by optimizing your code and resources. This includes minifying your CSS and JavaScript files, optimizing your images, and using efficient code. You can also use caching and content delivery networks (CDNs) to further improve loading times.

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.