Many WordPress sites allow visitors to share comments on blog posts. There are several WordPress plugins that also enable visitors to leave a reply to comments. However, this process can get confusing when more than one author is replied to or referenced in the comment.
In this tutorial, I’ll be covering how to write a handy WordPress plugin that brings a Twitter-like mention feature right into our WordPress site, to allow readers to tag each other in comments.
Key Takeaways
Coding the Plugin
All WordPress plugins are PHP files located at/wp-content/plugins/
directory. In here, we create a PHP file wp-mention-plugin.php.
Next, we include the plugin header, otherwise WordPress won’t recognize our plugin.
<?php
/**
* Plugin Name: WP Mention Plugin
* Plugin URI: https://sitepoint.com
* Description: Mention both registered and non registered @comment__author in comments
* Version: 1.0.0
* Author: John Doe
* Author URI: https://sitepoint.com
* License: GPLv2
*/
Now we create the plugin class as follows:
class wp_mention_plugin {
//codes
All our action and filter hooks will go into the initialize()
method, since we’re making this our constructor, the first method called when the class is instantiated.
public static function initialize() {
add_filter( 'comment_text', array ( 'wp_mention_plugin', 'wpmp_mod_comment' ) );
add_action( 'wp_set_comment_status', array ( 'wp_mention_plugin', 'wpmp_approved' ), 10, 2 );
add_action( 'wp_insert_comment', array ( 'wp_mention_plugin', 'wpmp_no_approve' ), 10, 2 );
}
Code Explanation
Thecomment_text
filter hook filters the text of comments to be displayed. The wp_set_comment_status
action hook is triggered immediately when the status of a comment changes, and the wp_insert_comment
action hook is triggered immediately when a comment is submitted.
We will be styling any name with a preceding ‘@’ symbol without quotes, and this is why we need the comment_text
filter hook.
In WordPress sites where comments are held for moderation before being approved, we need the wp_set_comment_status
action hook to track newly approved comments for the mentioned authors.
The wp_insert_comment
is needed to track comments for the mentioned author, in cases where comments were never held for moderation but automatically approved.
Now let’s create the functions called in our initialize()
method. First off, the wpmp_mod_comment()
public static function wpmp_mod_comment( $comment ) {
// we set our preferred @mention text color here
$color_code = '#00BFFF';
$pattern = "/(^|\s)@(\w+)/";
$replacement = "<span style='color: $color_code;'>$0</span>";
$mod_comment = preg_replace( $pattern, $replacement, $comment );
return $mod_comment;
}
Here, we create a method wpmp_mod_comment()
meaning: WP Mention Plugin Modify Comment. The function accepts a single parameter, which is the comment we’re modifying. Don’t worry about this parameter, WordPress handles it.
The $pattern
variable creates a simple regular expression pattern, which determines whether @any__comment__author__name is being mentioned in any comments. When things like admin@sitepoint are being included in a comment, they don’t get recorded as a valid mention. Valid mentions will be styled in a deep sky blue color.
If you would like to change the color of @mention text, just change the value of the $color_code
variable.
Before we proceed further, let’s create the method that handles the notification email sent to the mentioned comment authors.
private static function wpmp_send_mail( $comment ) {
$the_related_post = $comment->comment_post_ID;
$the_related_comment = $comment->comment_ID;
$the_related_post_url = get_permalink( $the_related_post );
$the_related_comment_url = get_comment_link( $the_related_comment );
// we get the mentions here
$the_comment = $comment->comment_content;
$pattern = "/(^|\s)@(\w+)/";
// if we find a match of the mention pattern
if ( preg_match_all( $pattern, $the_comment, $match ) ) {
// remove all @s from comment author names to effectively
// generate appropriate email addresses of authors mentioned
foreach ( $match as $m ) {
$email_owner_name = preg_replace( '/@/', '', $m );
$email_owner_name = array_map( 'trim', $email_owner_name );
}
/**
* This part is for For full names, make comment authors replace spaces them with
* two underscores. e.g, John Doe would be mentioned as @John__Doe
*
* PS: Mentions are case insensitive
*/
if ( preg_match_all( '/\w+__\w+/', implode( '', $email_owner_name ) ) ) {
$email_owner_name = str_ireplace( '__', ' ', $email_owner_name );
}
// we generate all the mentioned comment author(s) email addresses
$author_emails = array_map( 'self::wpmp_gen_email', $email_owner_name );
// ensure at least one valid comment author is mentioned before sending email
if ( ! is_null( $author_emails ) ) {
$subj = '[' . get_bloginfo( 'name' ) . '] Someone mentioned you in a comment';
$email_body = "Hello, someone mentioned you in a comment($the_related_comment_url)".
" at MyBlog.com. Here is a link to the related post: $the_related_post_url";
wp_mail( $author_emails, $subj, $email_body );
}
}
}
Code Explanation
In the above method, we filter the comment to check if anyone is being mentioned. And if true, we remove the preceeding ‘@’ symbol before the comment author name, so that we can generate the comment author info from the database using the name. At times, comment authors do fill in full names, for example ‘John Doe’, or names with spaces as their comment author name. In cases like this, anyone mentioning them must replace spaces in the name with double underscores. So starting from the lineif ( preg_match_all( '/\w+__\w+/', implode( '', $email_owner_name ) ) ) ....
we ensure that all double underscores in @mention text gets converted into spaces.
We then generate the mentioned comment author email address using the wpmp_gen_email()
method we’ll be creating next. The $email_body
variable and the wp_mail()
function stores and sends the notification email respectively, with a link to the corresponding comment and post.
NOTE If you noticed in the codes, mentioned comment authors and their email addresses are stored in an array, this is just in case more than one comment author is mentioned.
Remember to change the line MyBlog.com
in $email_body
to your desired site name.
public static function wpmp_gen_email( $name ) {
global $wpdb;
$name = sanitize_text_field( $name );
$query = "SELECT comment_author_email FROM {$wpdb->comments} WHERE comment_author = %s ";
$prepare_email_address = $wpdb->prepare( $query, $name );
$email_address = $wpdb->get_var( $prepare_email_address );
return $email_address;
}
Code Explanation
I’ve talked much on this method earlier, it simply generates the mentioned comment author email. The$name
string parameter it accepts is the comment author name. Then it gets the mentioned comment author info from the database using the comment author name. To prevent an SQL attack, we use the WordPress prepare()
function to ensure queries are safely handled.
Now we create two more methods which track whether a comment is first held for moderation or not, before sending the notification email.
public static function wpmp_approved( $comment_id, $status ) {
$comment = get_comment( $comment_id, OBJECT );
( $comment && $status == 'approve' ? self::wpmp_send_mail( $comment ) : null );
}
public static function wpmp_no_approve( $comment_id, $comment_object ) {
( wp_get_comment_status( $comment_id ) == 'approved' ? self::wpmp_send_mail( $comment_object ) : null );
}
The wpmp_approved()
firstly checks if a comment with $comment_id
held for moderation is approved, before calling the wpmp_send_mail()
method which sends the notification email.
While wpmp_no_approve()
calls the wpmp_send_mail()
method which sends the email notification immediately when a comment is being automatically approved, without first holding for moderation.
Before the plugin can do what we’ve just coded it to do, we have to instantiate the class wp_mention_plugin
we’ve just worked on, and call the initialize()
method which registers our needed action and filter hooks.
}
$wp_mention_plugin = new wp_mention_plugin;
$wp_mention_plugin->initialize();
?>
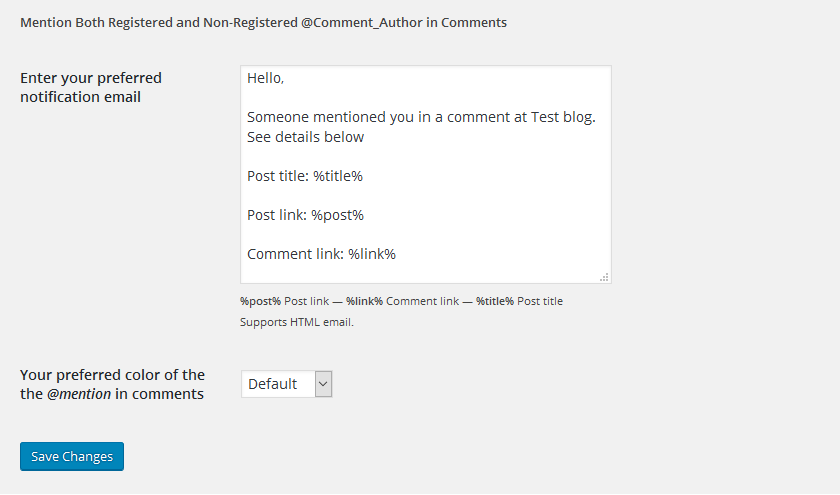
Conclusion
In this tutorial, I covered how to create a handy WordPress plugin that brings a Twitter-like mention feature right into our WordPress site.Frequently Asked Questions about WordPress Comments Mention Feature
How can I enable the WordPress comments mention feature on my website?
Enabling the WordPress comments mention feature is quite straightforward. You need to install and activate the ‘Comments – wpDiscuz’ plugin. After activation, navigate to the plugin settings page and enable the ‘User Mentioning’ option. This will allow your website users to mention each other in the comments section.
What is the purpose of the wp_insert_comment function in WordPress?
The wp_insert_comment function in WordPress is used to insert a new comment into the database. This function is useful when you want to programmatically add a comment to a post. It takes an array of comment data as an argument and returns the ID of the newly inserted comment.
How can I manage comments on my WordPress site?
WordPress provides a built-in system for managing comments. You can access this system by navigating to the ‘Comments’ section in your WordPress dashboard. Here, you can approve, disapprove, reply to, edit, or delete comments. You can also mark comments as spam or move them to the trash.
Can I use hooks with the wp_insert_comment function?
Yes, you can use hooks with the wp_insert_comment function. The ‘wp_insert_comment’ hook is triggered immediately after a comment is inserted into the database. You can use this hook to perform additional actions when a comment is inserted, such as sending a notification email.
How can I insert comments into WordPress programmatically?
You can insert comments into WordPress programmatically by using the wp_insert_comment function. This function takes an array of comment data as an argument and inserts a new comment into the database. The comment data array should include the comment content, the comment author’s name, email, and URL, the comment post ID, and other optional parameters.
What is the Jetpack plugin and how does it help with comments?
Jetpack is a popular WordPress plugin that offers a variety of features, including enhanced comment functionality. With Jetpack, you can enable social media login for comments, allow users to subscribe to comments, and more. It also provides a more modern, streamlined comment form compared to the default WordPress comment form.
How can I prevent spam comments on my WordPress site?
There are several strategies to prevent spam comments on your WordPress site. You can use a plugin like Akismet to automatically filter out spam comments. You can also enable comment moderation in your WordPress settings, which means that comments won’t appear on your site until you approve them.
Can I customize the appearance of the comments section on my WordPress site?
Yes, you can customize the appearance of the comments section on your WordPress site. This can be done by modifying the comments.php file in your theme, or by using a plugin that provides comment customization options.
How can I disable comments on my WordPress site?
You can disable comments on your WordPress site by navigating to the ‘Discussion’ settings in your WordPress dashboard. Here, you can uncheck the box that says ‘Allow people to post comments on new articles’. This will disable comments on all future posts. If you want to disable comments on existing posts, you will need to do so on a post-by-post basis.
Can I import and export comments in WordPress?
Yes, you can import and export comments in WordPress. This can be done by using the built-in WordPress import and export tool, which can be found in the ‘Tools’ section of your WordPress dashboard. This tool allows you to export your comments to an XML file, which can then be imported into another WordPress site.
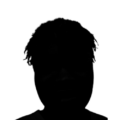
Software developer and marketing consultant. Loves experimenting with product development and design.