JavaScript is an increasingly popular language thanks to the proliferation of web apps and the adoption of HTML5. Part of JavaScript’s appeal is the ease by which one can begin writing useful and fun stuff with it. There’s no need for heavyweight integrated development environments (IDEs) or third-party apps. Just open any text editor, save the file, and open it in a web browser. JavaScript’s lure can easily turn into a trap for novice programmers. The malleability of the language can create monstrous bugs in sophisticated scripts. For example, a missing local variable declaration can mysteriously manifest itself elsewhere on the page by altering global variables.
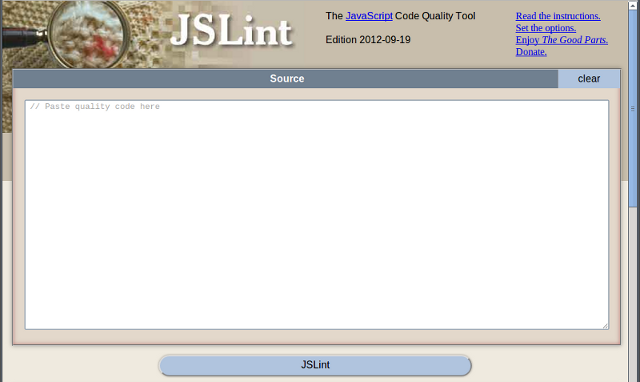
Using JSLint
Let’s run through an example usage of JSLint. We are writing a simple jQuery plugin that displays a message specified in themsg
parameter with a prefix. The prefix is hidden if we pass the value false
through the type
parameter.
(function ($) {
$.fn.loading = function(msg, type, cssClass){
var prefixes = {
warning: 'Warning: ' + msg,
error: 'Error: ' + msg,
info: 'Info: ' + msg,
warning: 'Caution: ' + msg,
};
if (type) {
concatMsg = prefixes[type];
} else {
concatMsg = msg;
}
$(this).each(function() {
var tis = $(this)
if (msg == false) {
tis.html('');
} else {
tis.html(concatMsg);
}
});
}
})(jQuery);
Although this piece of code works fine as a plugin for jQuery, when you use it in Firefox or Chrome, you can see that there are some glaring mistakes and some very subtle ones. Instead of spending your mental energy digging out the problems, let’s use JSLint to help us. Copy the function code into the text area on the JSLint site and click the “JSLint” button. Some of the resulting JSLint output is shown in the figure below.

"use strict"
statement is missing. This error indicates that the function is not executed in strict mode. To correct this error, enable strict mode by adding the following string literal to the beginning of the function body.
'use strict';
After enabling strict mode, click the “JSLint” button again. The reported error of the missing "use strict"
should be gone. We can now move on to the next error, shown in the following figure. This error deals with whitespace, and is more cosmetic than functional. Since this is not an actual error, you can safely ignore it.

function
keyword, and suppress the error message by scrolling to the bottom of the page and switching the “messy white space” option to true
. For now, however, we want to keep the default behavior because this option also checks for other whitespace issues as we will see later.
Also notice that the second and third errors reported by JSLint are also on the same line but at different positions. Looks like a space between the closing parenthesis and the opening brace is also recommended by JSLint, so fix that now.
By clicking the “JSLint” button again, we see that the next problem is on line 8, at position 39. The prefixes
object literal contains two identical warning
properties, which is obviously a mistake. Let’s correct the problem by replacing the second occurrence of warning
with caution
.


concatMsg
was used before it was defined. When a variable is not defined in the current scope, JavaScript checks the enclosing scopes to see if it was defined elsewhere. If you are using code from a third-party source that happens to define this exact variable in the global scope, it can take you countless, hair-pulling hours to find the bug. Fortunately, with JSLint, we are able to nip the problem in the bud.

concatMsg
is msg
, we can assign this value immediately and change it later if need be. The code for concatMsg
can now be rewritten as shown below.
var concatMsg = msg;
if (type) {
concatMsg = prefixes[type];
}
Next, we encounter the same whitespace issue as earlier, which can be corrected in the same way. Next, JSLint reports that a semicolon is missing. This message is shown below. Without the semicolon, JSLint assumes that the statement is never terminated. That is why it saw if
while expecting a semicolon. While the language specification says that the ending semicolon is optional, it is good practice to include it. This is another area where sloppy coding can lead to hard-to-find bugs in large scale productions. By linting our code, we can fix such problems quickly and easily.

false
is specified as the first parameter. To correct the error, use the strict equality operator.

concatMsg
is right after prefixes
, JSLint prefers that you group the variable declarations in a single statement, separated by commas.
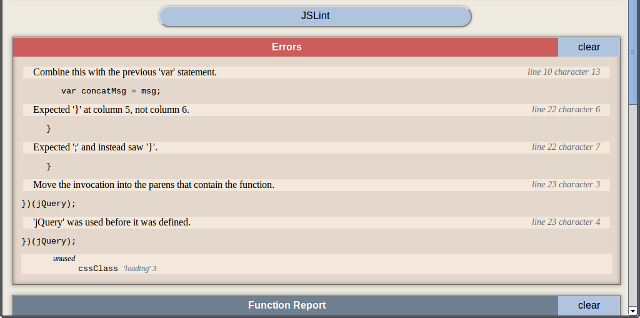


jQuery
into the bottom text field.

true
, as shown below. Choose this option only if you really want to keep the parameter in the function signature for some reason.
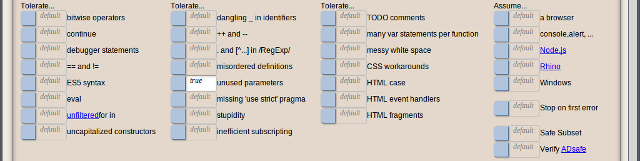
(function ($) {
'use strict';
$.fn.loading = function (msg, type, cssClass) {
var prefixes = {
warning: 'Warning: ' + msg,
error: 'Error: ' + msg,
info: 'Info: ' + msg,
caution: 'Caution: ' + msg
}, concatMsg = msg;
if (type) {
concatMsg = prefixes[type];
}
$(this).each(function () {
var tis = $(this);
if (msg === false) {
tis.html('');
} else {
tis.html(concatMsg);
}
});
};
}(jQuery));
JSLint Directives
JSLint directives allow you to define variables and provide other options to JSLint from directly within the source code. This frees you from having to set the JSLint GUI options repeatedly. For example, the comments in the following example define a global variable namedjQuery
and set the “unparam” option to true
.
/*global jQuery*/
/*jslint unparam: true */
(function ($) {
‘use strict’;
…
}(jQuery));
Conclusion
In this short example, JSLint has pointed out some critical errors and some seemingly insignificant ones. The fact that JSLint helps us catch these issues before we actually run the code is hugely beneficial in terms of developer productivity and application quality. If you are serious about writing production quality code, always run it through JSLint before pushing it to the server. JSLint is even contained in a single JS file, so you can download it use it offline too!Frequently Asked Questions (FAQs) about Using JSLint to Refine Your Code
What is the significance of the “use strict” statement in JavaScript?
The “use strict” directive is a way to enforce stricter parsing and error handling on your JavaScript code at runtime. It helps catch common coding bloopers, preventing potentially harmful actions. This statement can be placed at the beginning of a script or a function. When used, it can prevent the use of undeclared variables, the deletion of variables, functions, or function arguments, and the duplication of parameter names, among other things. It’s a good practice to use this statement in your code to avoid common pitfalls and keep your code safe.
How does JSLint help in refining JavaScript code?
JSLint is a static code analysis tool used in software development for checking if JavaScript source code complies with coding rules. It takes a JavaScript source and scans it, identifying potential problems within the code that may not necessarily make it into runtime errors, but could lead to unexpected behavior. These problems could be syntax errors, structural problems, typographical mistakes, or non-adherence to coding standards. By using JSLint, developers can catch these issues early, making the code more reliable and maintainable.
Why am I getting a “missing ‘use strict’ statement” error in JSLint?
JSLint expects a “use strict” statement at the top of your JavaScript files. This is a directive that helps catch common mistakes in your code. If it’s missing, JSLint will throw an error. To fix this, simply add “use strict”; at the beginning of your file or function. This will enforce stricter parsing and error handling in your code.
Can I ignore the “missing ‘use strict’ statement” error in JSLint?
While it’s technically possible to ignore this error, it’s not recommended. The “use strict” directive helps catch common coding mistakes and prevents potentially harmful actions. Ignoring this error could lead to unexpected behavior in your code. It’s best to include the “use strict” statement at the beginning of your JavaScript files or functions to ensure your code is safe and reliable.
How can I add the “use strict” statement to my JavaScript code?
Adding the “use strict” statement to your JavaScript code is simple. Just add the following line at the top of your JavaScript file or function: “use strict”;. This will enforce stricter parsing and error handling in your code, helping to catch common coding mistakes and prevent potentially harmful actions.
What are some common issues that JSLint can help identify?
JSLint can help identify a variety of issues in your JavaScript code. These include syntax errors, structural problems, typographical mistakes, and non-adherence to coding standards. By using JSLint, you can catch these issues early, making your code more reliable and maintainable.
How can I use JSLint to check my JavaScript code?
To use JSLint, simply paste your JavaScript code into the JSLint text area and click the “JSLint” button. JSLint will then scan your code and identify any potential problems. These problems will be displayed in the “JSLint Report” area, allowing you to easily identify and fix any issues in your code.
Can JSLint help improve the performance of my JavaScript code?
While JSLint primarily focuses on identifying potential problems in your code that could lead to unexpected behavior, using it can indirectly improve the performance of your code. By catching issues early and ensuring your code adheres to coding standards, JSLint can help make your code more efficient, reliable, and maintainable.
Is it necessary to use JSLint for JavaScript development?
While it’s not strictly necessary to use JSLint for JavaScript development, it’s highly recommended. JSLint can help catch common coding mistakes and ensure your code adheres to coding standards. This can make your code more reliable and maintainable, and can save you time and effort in the long run.
Are there alternatives to JSLint for checking JavaScript code?
Yes, there are several alternatives to JSLint for checking JavaScript code. These include JSHint, ESLint, and TSLint, among others. Each of these tools has its own set of features and rules, so you can choose the one that best fits your needs. However, JSLint is a popular choice due to its strict and comprehensive set of rules.
Chee How is a technology enthusiast and is especially interested in all Web related stuff. He currently works as a Web developer in a company he co-founded, and writes JavaScript, Java and PHP on a day-to-day basis.