Sometimes you may wish to simulate a delay of events such as simulating loading of results before showing on the page. This example uses a recursive setTimeout() to call a function which loops through an array of data which has the results of a system precheck to check for things such as JavaScript, Flash, Browser Version etc… When I get time I might code this into a jQuery plugin which will be easy just need to determine which options to provide to cater for different usage.
Demo
jQuery Code Recursive setTimeout()
//data and settings
var result = 'Precheck passed.', //html for main result
delay = 500, //delay of sub results
data = Array(
'Javascript ',
'System ',
'Device ',
'Browser ',
'Flash '
);
//self executing function starting from array index 0
(function process_els(el_index) {
var el = data[el_index],
precheckUl = $('#precheck ul'),
loadingLi = $(''),
sysPreId = "syspre_"+el_index;
//show loading image
precheckUl.append(loadingLi.clone().attr("id",sysPreId));
//after simulated delay replace loading image with sub check result
setTimeout( function()
{
precheckUl.find('li.loading:first').replaceWith(data[el_index]);
}, delay);
//to simulate the delay recursively call itself until all array elements have been processed
if (el_index + 1 < data.length) {
setTimeout(function() { process_els(el_index + 1); }, delay);
}
else
{
setTimeout(function()
{
//append the final result after all sub checks have been inserted
precheckUl.after(result);
}, (delay*2));
}
})(0);
HTML
System Check
System Check
Frequently Asked Questions (FAQs) about Simulating Delay with SetTimeout
What is the purpose of using SetTimeout in JavaScript?
SetTimeout is a method in JavaScript that allows you to schedule a function or a specific block of code to run after a delay. It’s a way to delay the execution of a function. This can be useful in various scenarios, such as creating animations, delaying alerts, or making a function wait for some data.
How does SetTimeout work?
SetTimeout works by taking two arguments: a function and a delay in milliseconds. When you call SetTimeout, it starts a timer. Once that timer reaches the specified delay, it will execute the function. It’s important to note that SetTimeout doesn’t pause the execution of the rest of your code. It simply schedules the function to run later.
Can I cancel a SetTimeout?
Yes, you can cancel a SetTimeout using the clearTimeout function. When you call SetTimeout, it returns a unique ID. You can pass this ID to clearTimeout to stop the timer and prevent the function from being executed.
How can I use SetTimeout to create a delay in jQuery?
While jQuery has its own delay method, you can still use SetTimeout in a jQuery context. You would use it in the same way as you would in regular JavaScript, by passing a function and a delay to SetTimeout.
What is the difference between SetTimeout and jQuery’s delay method?
While both SetTimeout and jQuery’s delay method can be used to create a delay, they work in slightly different ways. SetTimeout works with JavaScript functions, while delay works with jQuery animations. Delay is specifically designed to work with jQuery’s effects queue.
Can I use SetTimeout with async/await?
Yes, you can use SetTimeout with async/await to create a delay in an asynchronous function. To do this, you would need to wrap SetTimeout in a Promise.
Why is my SetTimeout not working?
There could be several reasons why your SetTimeout is not working. You might have made a syntax error, or you might be trying to use a variable that hasn’t been defined yet. It’s also possible that the delay is too short to notice, or that the function you’re trying to execute is causing an error.
Can I use SetTimeout in a loop?
Yes, you can use SetTimeout in a loop. However, because SetTimeout is non-blocking, all of the timeouts will start at the same time. If you want to create a sequence of delays, you would need to increase the delay for each iteration of the loop.
How can I test a function that uses SetTimeout?
Testing a function that uses SetTimeout can be tricky because of the delay. One approach is to use a testing framework that supports asynchronous testing. Another approach is to mock SetTimeout, so you can control when the function is executed.
Is there a limit to the delay I can set with SetTimeout?
The maximum delay you can set with SetTimeout is 2147483647 milliseconds, or about 24.8 days. If you try to set a delay longer than this, it will be reduced to 1.
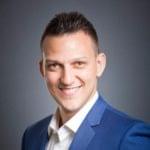
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.