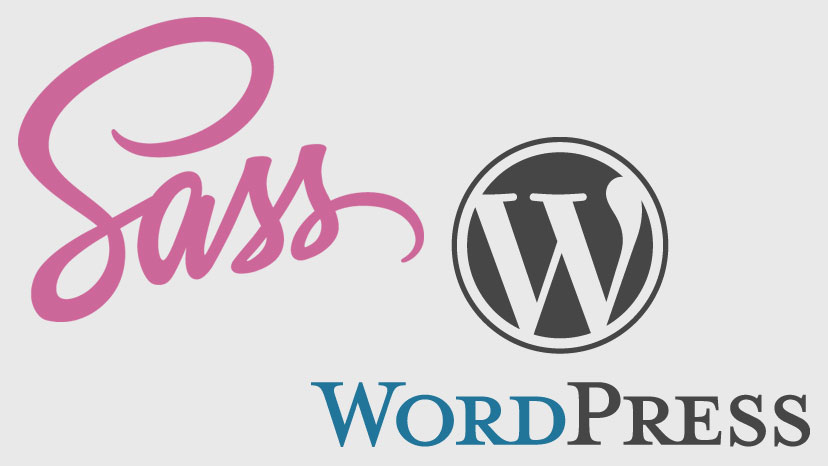
Convert a Stylesheet to Sass
The best way to start using Sass in WordPress development is to use a theme that has Sass files included. The Underscores theme is my favorite starting place for a new theme. However, if you’re starting with a theme that doesn’t have Sass files included, you’ll need to convert the existing stylesheet to Sass. The good news is that if you’re using the .scss syntax (which I recommend), your existing CSS is all valid Sass. You can simply copystyle.css
to style.scss
and that .scss file will compile properly.
Setting Up Partials
At this point, you’ve got a long .scss file with just as much code as your original CSS file. You can now use variables and mixins, but this still isn’t organized better yet. We can get some much-needed organization by breaking the long stylesheet up into smaller files. Copy each “section” ofstyle.css
to a separate .scss file in your Sass folder. Name this file after what it contains with an underscore prefix. For example, your styles relating to your navigation go to _navigation.scss
.
Once you’ve moved code to a partial, you’ll need to import that partial in your main style.scss file. Essentially, you’ll copy all the navigation code to _navigation.scss
and replace it in style.scss
with an import command. Note: the file name in the import does not have the underscore or the extension.
[sass]
@import ‘navigation’;
[/sass]
CSS is still cascades (earlier styles are overridden by later styles of the same specificity), so be sure to keep these @import
statements in the order you need for your final output.
Refactor as Necessary
Once you’ve broken the long stylesheet into smaller partials (so you can find code more easily), you can work on refactoring those partials. This step helps make things easier to maintain, but I wouldn’t consider it essential. One way to refactor is to nest selectors. If you have several styles defined with in a certain parent selector, you’ll have this in your original stylesheet: [sass] .header .logo { //styles } .header .tagline { //styles } .header .menu { //styles } [/sass] You can refactor those styles to make them easier to scan and edit by nesting the child selectors like this. The following block of Sass will compile to the same CSS we had above. [sass] .header { .logo { //styles } .tagline { //styles } .menu { //styles } } [/sass] You can also refactor by replacing repeated prefixed properties with short mixins. [sass] .menu a { -webkit-transition: color 0.2s; transition: color 0.2s; } [/sass] A mixin can do the same thing. Note: I haven’t include the@mixin
statement here: I recommend using the Bourbon or Compass mixin libraries for standard things like this.
[sass]
.menu a {
@include transition(color 0.2s);
}
[/sass]
Further note: I actually don’t recommend using mixins for prefixing: AutoPrefixer is a much better solution, but that’s a different article.
Compile Your Sass for WordPress
Now that we’ve broken the bigstyle.css
file down: how do we put it back together?
Compiler Requirements
WordPress has a few unique requirements for the CSS output. This file needs to be named style.css, it must live in the theme root, and it needs a special block of WordPress comments at the top. In addition those WordPress-specific considerations, I’d also add the following requirements for our compile process. It’s best to keep all the Sass files in a subdirectory (/sass/
) so we can keep the theme root clean. We should also be able to compress CSS output for our deployed code.
GUI Compile Methods
If you prefer an app to compile your Sass, the following apps provide a GUI for selecting and watching folders of .scss files.- Codekit ($30)
- Koala (free)
- Scout (free)
- Compass.app ($10)
CLI Compile Methods
I don’t use those apps often: I prefer the command line. There are two tools to compile your Sass from the command line: Sass and Compass. You can find instructions for installing Sass and Compass as command line tools (Ruby gems): Sass / Compass. Sass’s CLI provides thesass
command for compiling a Sass input file to CSS output: sass style.scss:style.css
. Of course, re-running that command every time you change something in your Sass partials isn’t ideal. You can add the --watch
parameter and Sass will watch the .scss file (and the partials it imports) and compile every time it detects a change: sass --watch style.scss:style.css
.
We can improve our compile method even more by using Compass instead of Sass. The biggest benefit of Compass is that it operates on a config.rb
file. You can save your configuration options (expanded vs. compressed, input & output directories, etc) in a file, then tell Compass to watch and compile your Sass by running compass watch
in the directory that contains config.rb
.
Here’s how I use my Compass config.rb
file:
- It lives in
/{theme-name}/sass/
- It ouputs
style.css
up a level to the theme root:css_dir = '..'
- The .scss files live in the
/sass/
directory:sass_dir = ''
- During development:
output_style = :expanded
- For deployment:
output_style = :compressed
WordPress style.css Comments
As we noted above, WordPress requires some specific comments at the top of the theme stylesheet. We can control those instyle.scss
in our /sass/
directory. Since Compass will remove comments with output_style = :compressed
, we’ll need to start the comment block with an exclamation mark (!) to preserve these comments.
[sass]
/*!
Theme Name: Sassy Theme
Theme URI: http://jamessteinbach.com/themes/sassy/
Author: James Steinbach
Author URI: http://jamessteinbach.com
Description: From CSS to Sass Sample Theme Code
*/
//import all your partials
[/sass]
Organize Your Partials
Now that we know how to turn a stylesheet into Sass and put it back together, let’s talk about how to organize your Sass partials. The first thing to keep in mind is that cascades still matter. Make sure to import your broadest styles first and your most specific styles last. You can use folders to organize your partials. For a partial in a folder, your@import
statement would include the directory name like this:
[sass]
@import ‘base/variables’;
// imports _variables.scss from the /base/ directory
[/sass]
There are many ways to group your partials into folders. The “Sass Resources” list below contains some good philosophies for organizing partials. Here’s one example:
- /base/ (variables, mixins, reset, typography)
- /layout/ (grid, header, footer)
- /vendors/ (plugins, vendors, etc)
- /components/ (buttons, menus, forms, widgets)
- /pages/ (home, landing page, portfolio)
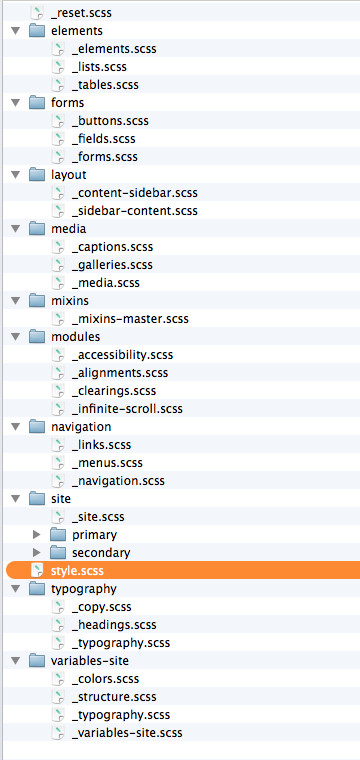
Sass Resources
We’ve just scratched the surface of using Sass to build WordPress themes. If this is your first read on the topic, you might be wondering, “Where do I get more information?” The following lists provide more information on methods for using Sass for WordPress development. Some of their advice differs from this post: you can follow the path that works best for you.Additional Sass Reading
- “Compass Compiling and WordPress Themes,” Chris Coyier
- “How to Use Sass with WordPress,” Andy Leverenz
- “Architecture for a Sass Project,” Hugo Giraudel
Frequently Asked Questions (FAQs) about Using SASS for WordPress Development
How can I integrate SASS into my WordPress theme?
Integrating SASS into your WordPress theme involves a few steps. First, you need to install Node.js and npm on your computer. Then, you can install SASS globally using npm. Once SASS is installed, you can create a new SASS file in your theme directory and start writing your SASS code. To compile your SASS code into CSS, you can use a task runner like Gulp or Grunt. These tools will automatically compile your SASS code whenever you save your file.
What are the benefits of using SASS in WordPress development?
SASS, or Syntactically Awesome Stylesheets, is a CSS preprocessor that can make your stylesheets more readable, maintainable, and DRY (Don’t Repeat Yourself). It allows you to use variables, nested rules, mixins, and functions, which can greatly simplify your CSS code and make it easier to manage. This can be particularly beneficial in WordPress development, where themes can often involve complex and extensive stylesheets.
Can I use SASS with existing WordPress themes?
Yes, you can use SASS with existing WordPress themes. However, it requires some setup. You’ll need to create a new SASS file in your theme directory and then import your existing CSS files into this SASS file. Once this is done, you can start refactoring your CSS code into SASS.
How can I compile my SASS files in WordPress?
To compile your SASS files in WordPress, you can use a task runner like Gulp or Grunt. These tools will automatically compile your SASS code into CSS whenever you save your file. You can also use a WordPress plugin like WP-SCSS, which compiles your SASS files on the fly.
What is the difference between SASS and SCSS?
SASS and SCSS are both syntaxes for the SASS preprocessor. The main difference between them is that SASS uses a strict indentation syntax and doesn’t use semicolons or braces, while SCSS uses a syntax similar to CSS, with braces and semicolons.
How can I use variables in SASS?
In SASS, you can define variables with the $ symbol. For example, you could define a variable for your primary color like this: $primary-color: #333;. Then, you can use this variable throughout your SASS code, like this: body { background-color: $primary-color; }.
What are mixins in SASS and how can I use them?
Mixins in SASS are a way to define reusable chunks of CSS code. You can define a mixin with the @mixin directive and then include it in your code with the @include directive. For example, you could define a mixin for a clearfix like this: @mixin clearfix { &::after { content: “”; display: table; clear: both; } }. Then, you can include this mixin in your code like this: .container { @include clearfix; }.
Can I use SASS in WordPress without a build tool?
Yes, you can use SASS in WordPress without a build tool by using a WordPress plugin like WP-SCSS. This plugin compiles your SASS files on the fly, so you don’t need to use a task runner like Gulp or Grunt.
How can I debug my SASS code in WordPress?
To debug your SASS code in WordPress, you can use source maps. Source maps are files that map your compiled CSS code back to your original SASS code, making it easier to debug your code in the browser’s developer tools.
Can I use SASS with the WordPress Customizer?
Yes, you can use SASS with the WordPress Customizer. However, since the Customizer works with CSS code, you’ll need to compile your SASS code into CSS before you can use it in the Customizer. You can do this with a task runner like Gulp or Grunt, or with a WordPress plugin like WP-SCSS.
James is a Senior Front-End Developer with almost 10 years total experience in freelance, contract, and agency work. He has spoken at conferences, including local WordPress meet-ups and the online WP Summit. James's favorite parts of web development include creating meaningful animations, presenting unique responsive design solutions, and pushing Sass’s limits to write powerful modular CSS. You can find out more about James at jamessteinbach.com.