Quick jQuery code snippet on how to keep an element in view. For demo scroll down on any page on the blog and see ads on right sidebar ads, they remain inview as you scroll down the page. Then go back to normal view when you scroll up again.
Demo
Scroll down this page and look at the right hand sidebar.The Code
//keep element in view
(function($)
{
$(document).ready( function()
{
var elementPosTop = $('#jq4u-sidebar-ads').position().top;
$(window).scroll(function()
{
var wintop = $(window).scrollTop(), docheight = $(document).height(), winheight = $(window).height();
//if top of element is in view
if (wintop > elementPosTop)
{
//always in view
$('#jq4u-sidebar-ads').css({ "position":"fixed", "top":"10px" });
}
else
{
//reset back to normal viewing
$('#jq4u-sidebar-ads').css({ "position":"inherit" });
}
});
});
})(jQuery);
Turning it into a plugin
Could quite easily be made into a jQuery plugin. Actually I’ll code one out real quick and include it below./**
* jQuery keep element in view plugin.
*
* @author Sam Deering
* @copyright Copyright (c) 2012 jQuery4u
* @license http://jquery4u.com/license/
* @link http://jquery4u.com
* @since Version 1.0
* @notes Plugin only works on scroll down elements.
*
*/
(function($)
{
$.fn.keepElementInView = function()
{
var elemPosTop = this.position().top;
$(window).scroll(function()
{
var wintop = $(window).scrollTop(), docheight = $(document).height(), winheight = $(window).height();
if (wintop > elemPosTop)
{
this.css({ "position":"fixed", "top":"10px" });
}
else
{
this.css({ "position":"inherit" });
}
});
return this;
};
$(document).ready( function()
{
jQuery('#jq4u-sidebar-ads').keepElementInView();
});
})(jQuery);
Frequently Asked Questions (FAQs) about jQuery Element View Scrolling
How can I animate the scroll to a specific element using jQuery?
To animate the scroll to a specific element using jQuery, you can use the ‘animate’ function along with the ‘scrollTop’ property. The ‘scrollTop’ property gets the current vertical position of the scroll bar for the first element in the set of matched elements. Here’s a simple example:$('html, body').animate({
scrollTop: $("#elementID").offset().top
}, 2000);
In this code, ‘#elementID’ is the ID of the element you want to scroll to, and ‘2000’ is the duration of the animation in milliseconds.
How can I scroll to a specific element without animation?
If you want to scroll to a specific element instantly without any animation, you can use the ‘scrollTop’ function directly. Here’s how you can do it:$('html, body').scrollTop($("#elementID").offset().top);
In this code, ‘#elementID’ is the ID of the element you want to scroll to.
How can I scroll to a specific element horizontally using jQuery?
To scroll to a specific element horizontally, you can use the ‘scrollLeft’ property. Here’s an example:$('html, body').animate({
scrollLeft: $("#elementID").offset().left
}, 2000);
In this code, ‘#elementID’ is the ID of the element you want to scroll to, and ‘2000’ is the duration of the animation in milliseconds.
How can I check if an element is in the viewport using jQuery?
You can use the ‘offset’ method to get the current coordinates of an element relative to the document, and then compare it with the scroll position and the window’s height and width. Here’s a simple function that does this:function isInViewport(element) {
var elementTop = $(element).offset().top;
var elementBottom = elementTop + $(element).outerHeight();
var viewportTop = $(window).scrollTop();
var viewportBottom = viewportTop + $(window).height();
return elementBottom > viewportTop && elementTop < viewportBottom;
}
In this function, ‘element’ is the jQuery object of the element you want to check.
How can I scroll to a specific element when a button is clicked?
You can attach a click event handler to the button, and inside the handler, you can use the ‘animate’ function to scroll to the specific element. Here’s an example:$("#buttonID").click(function() {
$('html, body').animate({
scrollTop: $("#elementID").offset().top
}, 2000);
});
In this code, ‘#buttonID’ is the ID of the button, and ‘#elementID’ is the ID of the element you want to scroll to when the button is clicked.
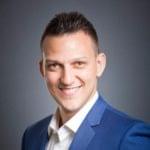
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.