Simple jQuery code snippet to count the number of checkboxes checked in a table.
$('#table :input[type="checkbox"]:checked').length
Frequently Asked Questions (FAQs) about jQuery Count Number of Checked Checkboxes
How can I count the number of checked checkboxes using pure JavaScript?
While jQuery provides a simple and efficient way to count the number of checked checkboxes, you can also achieve this using pure JavaScript. You can use the querySelectorAll
method to select all checkboxes and then use the Array.prototype.filter
method to filter out the checked checkboxes. Here’s a simple example:let checkboxes = document.querySelectorAll('input[type="checkbox"]');
let checked = Array.from(checkboxes).filter(input => input.checked);
console.log(checked.length);
This code will log the number of checked checkboxes to the console.
Can I count the number of checked checkboxes in real-time?
Yes, you can count the number of checked checkboxes in real-time using jQuery’s .change()
event. This event is triggered whenever the state of the checkbox changes. You can use this event to update the count whenever a checkbox is checked or unchecked. Here’s an example:$('input[type="checkbox"]').change(function() {
let count = $('input[type="checkbox"]:checked').length;
console.log(count);
});
This code will log the current number of checked checkboxes to the console whenever a checkbox is checked or unchecked.
How can I display the count of checked checkboxes on my webpage?
You can display the count of checked checkboxes on your webpage by updating the text content of an HTML element. Here’s an example:$('input[type="checkbox"]').change(function() {
let count = $('input[type="checkbox"]:checked').length;
$('#count').text(count);
});
In this example, #count
is the id of the HTML element where you want to display the count.
Can I count the number of checked checkboxes in a specific group?
Yes, you can count the number of checked checkboxes in a specific group by using a more specific selector. For example, if your checkboxes are inside a div with the id group1
, you can count the number of checked checkboxes in this group like this:$('div#group1 input[type="checkbox"]').change(function() {
let count = $('div#group1 input[type="checkbox"]:checked').length;
console.log(count);
});
This code will log the number of checked checkboxes in group1
to the console.
How can I count the number of unchecked checkboxes?
You can count the number of unchecked checkboxes by using the :not(:checked)
selector. Here’s an example:let count = $('input[type="checkbox"]:not(:checked)').length;
console.log(count);
This code will log the number of unchecked checkboxes to the console.
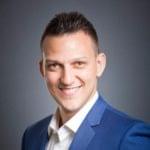
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.