Over the years, WordPress has become a target for spammers due to it increasing popularity.
Unfortunately, automated software exists whose purpose is to crawl the web in search of websites that are built with any popular platform, such as WordPress, and to submit hundreds, even thousands of spam comments. Spam comments are very annoying, they consume our precious time when it comes to moderating and deleting them.
I know you hate spam comments as much as I do and would love to know how to combat them. One way of deterring bots from submitting spam comments is by integrating a CAPTCHA to the comment form.
In previous tutorials, we learned how to integrate CAPTCHAs to the WordPress login and registration form.
In similar fashion, we’ll now run through how to integrate a CAPTCHA with the WordPress comment system.
There are many CAPTCHA plugins available in the WordPress plugin directory such as WP-reCAPTCHA and Securimage-WP-Fixed.
The aim of this tutorial is to not create yet another CAPTCHA plugin but to:
- Demonstrate how the WordPress HTTP API can be used in a plugin.
- How to include additional form fields to the WordPress comment form.
- How to validate and utilise the values added to custom fields.
Without further ado, let’s get started with the plugin development.
Plugin Development
First off, head over to reCAPTCHA, register your domain name and grab your public and private API keys.
Include the plugin header.
<?php
/*
Plugin Name: Add reCAPTCHA to comment form
Plugin URI: https://www.sitepoint.com
Description: Add Google's reCAPTCHA to WordPress comment form
Version: 1.0
Author: Agbonghama Collins
Author URI: http://w3guy.com
License: GPL2
*/
Create a class with three properties that will store the reCAPTCHA’s private & public key as well as the CAPTCHA error message (errors are generated when the CAPTCHA form is left empty and a user fails the challenge).
class Captcha_Comment_Form {
/** @type string private key|public key */
private $public_key, $private_key;
/** @type string captcha errors */
private static $captcha_error;
The class magic constructor method will contain two pairs of action and filter hooks.
/** class constructor */
public function __construct() {
$this->public_key = '6Le6d-USAAAAAFuYXiezgJh6rDaQFPKFEi84yfMc';
$this->private_key = '6Le6d-USAAAAAKvV-30YdZbdl4DVmg_geKyUxF6b';
// adds the captcha to the WordPress form
add_action( 'comment_form', array( $this, 'captcha_display' ) );
// delete comment that fail the captcha challenge
add_action( 'wp_head', array( $this, 'delete_failed_captcha_comment' ) );
// authenticate the captcha answer
add_filter( 'preprocess_comment', array( $this, 'validate_captcha_field' ) );
// redirect location for comment
add_filter( 'comment_post_redirect', array( $this, 'redirect_fail_captcha_comment' ), 10, 2 );
}
Code explanation: First, my reCAPTCHA public and private keys are saved to their class properties.
The captcha_display()
method that will output the reCAPTCHA challenge is added to the comment form by the comment_form
Action.
The wp_head
Action includes the callback function delete_failed_captcha_comment()
that will delete any comment submitted that fail the CAPTCHA challenge.
The filter preprocess_comment
calls the validate_captcha_field()
method to ensure the CAPTCHA field isn’t left empty and also that the answer is correct.
The filter comment_post_redirect
call redirect_fail_captcha_comment()
to add some query parameters to the comment redirection URL.
Here is the code for captcha_display()
that will output the CAPTCHA challenge.
Additionally, it check if there is a query string attached to the current page URL and display the appropriate error message depending on the value of $_GET['captcha']
set by redirect_fail_captcha_comment()
/** Output the reCAPTCHA form field. */
public function captcha_display() {
if ( isset( $_GET['captcha'] ) && $_GET['captcha'] == 'empty' ) {
echo '<strong>ERROR</strong>: CAPTCHA should not be empty';
} elseif ( isset( $_GET['captcha'] ) && $_GET['captcha'] == 'failed' ) {
echo '<strong>ERROR</strong>: CAPTCHA response was incorrect';
}
echo <<<CAPTCHA_FORM
<style type='text/css'>#submit {
display: none;
}</style>
<script type="text/javascript"
src="http://www.google.com/recaptcha/api/challenge?k=<?= $this->public_key; ?>">
</script>
<noscript>
<iframe src="http://www.google.com/recaptcha/api/noscript?k=<?= $this->public_key; ?>"
height="300" width="300" frameborder="0"></iframe>
<br>
<textarea name="recaptcha_challenge_field" rows="3" cols="40">
</textarea>
<input type="hidden" name="recaptcha_response_field"
value="manual_challenge">
</noscript>
<input name="submit" type="submit" id="submit-alt" tabindex="6" value="Post Comment"/>
CAPTCHA_FORM;
}
/**
* Add query string to the comment redirect location
*
* @param $location string location to redirect to after comment
* @param $comment object comment object
*
* @return string
*/
function redirect_fail_captcha_comment( $location, $comment ) {
if ( ! empty( self::$captcha_error ) ) {
$args = array( 'comment-id' => $comment->comment_ID );
if ( self::$captcha_error == 'captcha_empty' ) {
$args['captcha'] = 'empty';
} elseif ( self::$captcha_error == 'challenge_failed' ) {
$args['captcha'] = 'failed';
}
$location = add_query_arg( $args, $location );
}
return $location;
}
The method validate_captcha_field()
as its name implies to validate the CAPTCHA answer by making sure the CAPTCHA field isn’t left empty and the supplied answer is correct.
/**
* Verify the captcha answer
*
* @param $commentdata object comment object
*
* @return object
*/
public function validate_captcha_field( $commentdata ) {
// if captcha is left empty, set the self::$captcha_error property to indicate so.
if ( empty( $_POST['recaptcha_response_field'] ) ) {
self::$captcha_error = 'captcha_empty';
}
// if captcha verification fail, set self::$captcha_error to indicate so
elseif ( $this->recaptcha_response() == 'false' ) {
self::$captcha_error = 'challenge_failed';
}
return $commentdata;
}
Let’s take a closer look at validate_captcha_field()
, specifically the elseif
conditional statement, a call is made to recaptcha_response()
to check if the CAPTCHA answer is correct.
Below is the code for the recaptcha_response()
.
/**
* Get the reCAPTCHA API response.
*
* @return string
*/
public function recaptcha_response() {
// reCAPTCHA challenge post data
$challenge = isset( $_POST['recaptcha_challenge_field'] ) ? esc_attr( $_POST['recaptcha_challenge_field'] ) : '';
// reCAPTCHA response post data
$response = isset( $_POST['recaptcha_response_field'] ) ? esc_attr( $_POST['recaptcha_response_field'] ) : '';
$remote_ip = $_SERVER["REMOTE_ADDR"];
$post_body = array(
'privatekey' => $this->private_key,
'remoteip' => $remote_ip,
'challenge' => $challenge,
'response' => $response
);
return $this->recaptcha_post_request( $post_body );
}
Allow me to explain how the recaptcha_response()
works.
A POST
request is sent to the endpoint http://www.google.com/recaptcha/api/verify with the following parameters.
privatekey
: Your private keyremoteip
The IP address of the user who solved the CAPTCHA.challenge
The value of recaptcha_challenge_field sent via the form.response
The value of recaptcha_response_field sent via the form.
The challenge and response POST data sent by the form is captured and saved to $challenge
and $response
respectively.
$_SERVER["REMOTE_ADDR"]
capture the user’s IP address and it to $remote_ip
.
WordPress HTTP API the POST
parameter to be in array form hence the code below.
$post_body = array(
'privatekey' => $this->private_key,
'remoteip' => $remote_ip,
'challenge' => $challenge,
'response' => $response
);
return $this->recaptcha_post_request( $post_body );
The recaptcha_post_request()
is a wrapper function for the HTTP API
which will accept the POST
parameter/body, make a request to the reCAPTCHA API and return true
if the CAPTCHA test was passed and false
otherwise.
/**
* Send HTTP POST request and return the response.
*
* @param $post_body array HTTP POST body
*
* @return bool
*/
public function recaptcha_post_request( $post_body ) {
$args = array( 'body' => $post_body );
// make a POST request to the Google reCaptcha Server
$request = wp_remote_post( 'https://www.google.com/recaptcha/api/verify', $args );
// get the request response body
$response_body = wp_remote_retrieve_body( $request );
/**
* explode the response body and use the request_status
* @see https://developers.google.com/recaptcha/docs/verify
*/
$answers = explode( "\n", $response_body );
$request_status = trim( $answers[0] );
return $request_status;
}
Any comment made by a user who failed the captcha challenge or left the field empty get deleted by delete_failed_captcha_comment()
/** Delete comment that fail the captcha test. */
function delete_failed_captcha_comment() {
if ( isset( $_GET['comment-id'] ) && ! empty( $_GET['comment-id'] ) ) {
wp_delete_comment( absint( $_GET['comment-id'] ) );
}
}
Finally, we close the plugin class.
} // Captcha_Comment_Form
We are done coding the plugin class. To put the class to work, we need to instantiate it like so:
new Captcha_Comment_Form();
On activation of the plugin, a CAPTCHA will be added to the WordPress comment form as show below.
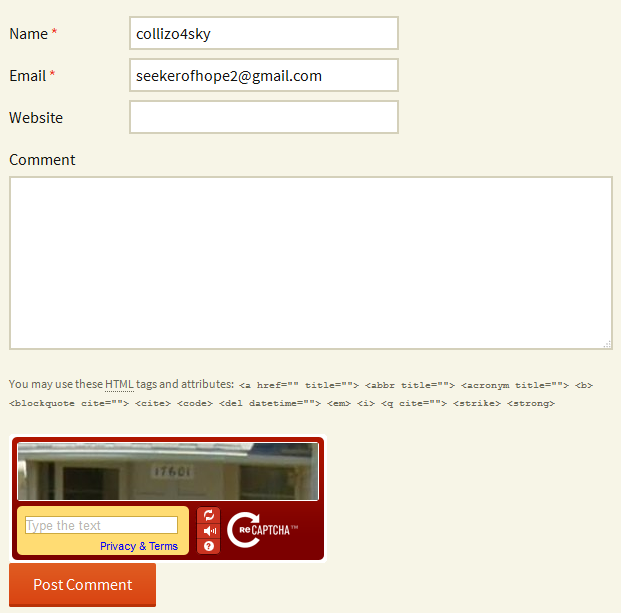
Wrap Up
At the end of this tutorial, you should be able to add extra form fields to the comment form and implement just about any feature you wish to have in the comment system thanks to the filters and actions mentioned.
If you wish to use the plugin on your WordPress site or to study the code in-depth, download the plugin from GitHub.
Until I come your way again, happy coding!
Frequently Asked Questions (FAQs) about Integrating a CAPTCHA with the WordPress Comment Form
What is the Importance of Integrating a CAPTCHA with the WordPress Comment Form?
Integrating a CAPTCHA with the WordPress comment form is crucial for several reasons. Firstly, it helps to prevent spam comments, which can clutter your website and deter genuine users. Secondly, it adds an extra layer of security, protecting your site from bots and automated scripts. Lastly, it saves you time and resources that would otherwise be spent moderating and deleting spam comments.
How Can I Customize the CAPTCHA on My WordPress Comment Form?
Customizing the CAPTCHA on your WordPress comment form can be done through the settings of the CAPTCHA plugin you are using. Most plugins offer options to change the complexity, design, and layout of the CAPTCHA. Some even allow you to create your own custom CAPTCHA.
Are There Any Alternatives to CAPTCHA for WordPress Comment Forms?
Yes, there are several alternatives to CAPTCHA for WordPress comment forms. These include Akismet, a spam filtering service, and Honeypot, a method that tricks bots into revealing themselves by interacting with a hidden form field.
Can I Use CAPTCHA on Other Forms on My WordPress Site?
Absolutely. CAPTCHA can be integrated with any form on your WordPress site, including contact forms, registration forms, and login forms. This provides additional security and spam prevention across your entire site.
What Should I Do If CAPTCHA Is Not Working on My WordPress Comment Form?
If CAPTCHA is not working on your WordPress comment form, first check to ensure that the plugin is properly installed and activated. If the problem persists, try clearing your browser cache or disabling other plugins to see if there is a conflict.
How Can I Make CAPTCHA More Accessible for Users with Disabilities?
To make CAPTCHA more accessible, consider using an audio CAPTCHA or a logic-based CAPTCHA, which asks users to answer a simple question. Also, ensure that your CAPTCHA plugin complies with Web Content Accessibility Guidelines (WCAG).
Is CAPTCHA Effective Against All Types of Spam?
While CAPTCHA is highly effective at preventing bot-generated spam, it may not be as effective against human-generated spam. For this, consider using additional measures such as comment moderation or blacklisting certain words or IP addresses.
Does Integrating CAPTCHA with the WordPress Comment Form Affect Site Performance?
Integrating CAPTCHA with the WordPress comment form should not significantly affect site performance. However, like any plugin, it does use some resources. If you notice a slowdown, consider using a lightweight CAPTCHA plugin or optimizing your site’s performance in other ways.
Can I Integrate CAPTCHA with the WordPress Comment Form Without a Plugin?
While it is technically possible to integrate CAPTCHA with the WordPress comment form without a plugin, it requires advanced coding knowledge and is not recommended for most users. Using a plugin simplifies the process and ensures that the CAPTCHA is correctly implemented.
How Often Should I Update My CAPTCHA Plugin?
It’s recommended to update your CAPTCHA plugin whenever a new version is released. This ensures that you have the latest security features and that the plugin remains compatible with the latest version of WordPress.
Collins is a web developer and freelance writer. Creator of the popular ProfilePress and MailOptin WordPress plugins. When not wrangling with code, you can find him writing at his personal blog or on Twitter.