We’ve previously showed you how to use the WordPress Links Manager to store and manage a list of links, we’ve also covered how to use wp_list_bookmarks()
from the Links Manager API.
We saw that we can do many things with this function, but it’s not perfect for all cases. In this article, I’ll show you how to use the other functions contained in the WordPress Links Manager API.
Contrary to wp_list_bookmarks()
, these functions don’t display the links: they return them and you decide if you want to display them and how, in a much more precise way than using the options of wp_list_bookmarks()
.
Retrieving One Link
Before looking at how to retrieve a list of links, we’ll first retrieve one link. In fact, playing with only one link is a more practical way to understand how the objects returned by the functions we will use are built.
A Function to Retrieve All Data about a Link
The first function we’ll study here is get_bookmark()
. It’s used to retrieve all the data about a link and requires one parameter: the wanted link’s ID. To retrieve this ID you can, for example, go to your administration panel and edit the link: the ID can be seen in the link_id
variable in the URL.
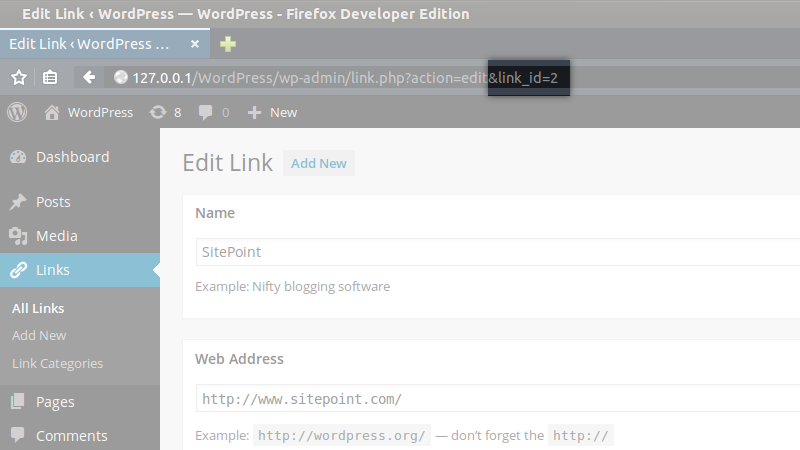
The Link Object
To retrieve the data we’re after, indicate this ID as a parameter in get_bookmark()
.
$link = get_bookmark(8);
The $link
variable is an object listing of all the data about the targeted link: for each piece of information you can add when you create a link, this object has a corresponding property. This is, for example, the content of the object I got with the previous line.
stdClass Object
(
[link_id] => 8
[link_url] => http://jeremyheleine.me
[link_name] => Jérémy Heleine
[link_image] =>
[link_target] =>
[link_description] => My personal webpage.
[link_visible] => Y
[link_owner] => 1
[link_rating] => 7
[link_updated] => 0000-00-00 00:00:00
[link_rel] => me
[link_notes] =>
[link_rss] =>
[link_category] => Array
(
[0] => 5
)
)
Most of these properties are clear, as their name is same as the corresponding fields in the ‘Add Link’ form: link_url
is the URL of the link, link_name
is its name, etc. Note that you can get the link’s ID with the property link_id
: it seems a bit useless here as you already know this, but later on I’ll show you that it can be useful.
echo '<a href="' . $link->link_url . '">' . $link->link_name . '</a>';
I’ll now describe some of the properties in further detail. For example, the link_category
: why is this property an array? This is because in the ‘Add Link’ form, you can select several categories for your link: this property lists all the selected category IDs in an array (even if you selected only one category).
The link_owner
property gives us the ID of the user who added the link. If you need to display more information about this user, you can use the get_user_by()
function.
// Get the owner's name
$owner_name = get_user_by('id', $link->link_owner)->display_name;
// Echoes a string containing the owner's name and their link
echo 'Here is ' . $owner_name . '\'s link: <a href="' . $link->link_url . '">' . $link->link_name . '</a>';
The link_target
property contains the value you indicated in the ‘Target’ section of the ‘Add Link’ form. You can display its value directly in the target
attribute of your link.
echo '<a href="' . $link->link_url . '" target="' . $link->link_target . '">' . $link->link_name . '</a>';
Note, if you chose to open the link in the same tab, WordPress stores an empty string in link_target
, so you can easily detect it if you don’t want to display the target
attribute.
// Build the target attribute
$target = (empty($link->link_target)) ? '' : ' target="' . $link->link_target . '"';
// Echoes the link with its target attribute (if it needs one)
echo '<a href="' . $link->link_url . '"' . $target . '>' . $link->link_name . '</a>';
Like the link_target
property, the link_rel
property contains the value to indicate in the rel
attribute so you don’t have to do anything before displaying it.
The link_image
and link_rss
properties contain the URLs you indicated in the corresponding fields. That means their values are URLs: in particular, you must generate your own img
tag if you want to display an image. The advantage is that you can do what you want with this image, even displaying it as a background image for example. In the example below, we don’t display the links name, only its image.
<a href="<?php echo $link->link_url; ?>">
<img src="<?php echo $link->link_image; ?>" alt="<?php echo $link->link_name; ?>" />
</a>
Note that this example doesn’t test if the link_image
property is empty. If the script you built is not only for you (e.g. if it is for a plugin or theme), you should test this before generating an img
tag.
Finally, we find the link_visible
property to know if the link is a private one or not. Sadly its value is not a boolean, but a string: if the link is visible (i.e. if it is not a private link), link_visible
is set to 'Y'
, otherwise it is set to 'N'
. Below we’ll display the link, only if it is visible.
if ($link->link_visible == 'Y')
echo '<a href="' . $link->link_url . '">' . $link->link_name . '</a>';
What If I Want an Array?
We described the properties of the object returned by get_bookmark()
. However, this function does not always return an object: it is the default behavior, but you can choose to retrieve an array if you prefer or if you don’t have a choice, for whatever reason.
To choose the type of the data you’ll get with this function, you can use the second parameter. It accepts a PHP constant as a value and is set by default to OBJECT
.
To get an associative array, you can set it to ARRAY_A
. The keys of this array will be the properties we saw in the object above so you should be familiar with this.
$link = get_bookmark(8, ARRAY_A);
echo '<a href="' . $link['link_url'] . '">' . $link['link_name'] . '</a>';
You can also choose to get a numbered array with ARRAY_N
. It will then be more difficult to get a specific data but the data is ordered in the same way that they were in the associative array or in the object: we will find the ID in the first entry, then the URL, then the name, and so on.
The Not so Useless Filter
To finish with get_bookmark()
, you should know that this function admits a third parameter: the filter to apply to the retrieved data.
WordPress does not give you the data directly returned by the database. In fact, it applies some filters to be sure that you have the right data in the right format. For example, the link ID is not a string containing the ID but a real number, and these filters are the reason why the link target is empty if it is set to _none
.
By default, the third parameter of get_bookmark()
is set to 'raw'
and WordPress returns the data right after its default filters. Most of the time, it is enough, but you can also choose to apply other filters.
There is essentially one reason for choosing another filter other than raw
: you want to retrieve the data to display in a form to let the users edit them. That can be useful if you want to use the Links Manager in your theme for example.
In this case, you can use the edit
filter. Then, WordPress will apply some other modifications, especially for escaping the HTML characters so you don’t have to do it yourself.
$link = get_bookmark(2, OBJECT, 'edit');
echo '<textarea>' . $link->link_notes . '</textarea>';
A Function to Retrieve Specific Information
Now that we’ve seen what information we can retrieve for one link, it’s time to look at a new function: get_bookmark_field()
. This function is useful in the case you want to retrieve specific information for one link, without retrieving the other information.
The get_bookmark_field()
function requires two parameters: the field (i.e. the information to retrieve) and the ID of the link in question. The field is a string corresponding with the properties of the object (or the keys of the associative array) returned by get_bookmark()
. For example, if you want to retrieve the URL of a link, you’ll write the line below:
$url = get_bookmark_field('link_url', 8);
which is equivalent to this one:
$url = get_bookmark(8)->link_url;
Retrieving Several Links
Above, we looked at how we can retrieve one link, but what about retrieving several links? Good news: there’s a function for this: get_bookmarks()
.
Known Output
You can try get_bookmarks()
without any parameters: it will then return an array containing all the links stored in the Links Manager.
This array is a numbered, each entry an object representing one link. This object is the same as we’ve covered above. For example, we can list all the links, regardless of their categories.
$links = get_bookmarks();
echo '<ul>';
foreach ($links as $link)
echo '<li><a href="' . $link->link_url . '">' . $link->link_name . '</a></li>';
echo '</ul>';
Filtering
If you don’t need all the links, filtering the output of get_bookmarks()
can be a good idea. That can be achieved easily, as only parameter is accepted by this function. Once again, you should be familiar with this as this parameter is similar to the one we covered in wp_list_bookmarks()
.
In fact, this parameter is an array listing all the options we need to filter the output. We described most of these options when we looked at wp_list_bookmarks()
. Here’s the list of the known options:
orderby
to sort the links,order
to reverse (or not) the order,limit
to only retrieve a limited number of links for each category,category
to choose the categories to retrieve,category_name
to choose only one category to retrieve (by using its name),hide_invisible
don’t retrieve the private links.
In addition to these options, we find three new options. First, we have include
which is a string in which you indicate a list of link IDs you want to retrieve. Note that if you use this option WordPress will ignore the values in category
, category_name
and exclude
(the next option we’ll cover).
The example below retrieves three links and orders the output following the rating given to these links.
$args = array(
'orderby' => 'rating',
'order' => 'DESC',
'include' => '4,5,10'
);
$links = get_bookmarks($args);
The exclude
option allows us to exclude a list of links using their IDs. You can combine this option with the other filters (except for include
) to, for example, retrieve a category without a given link.
$args = array(
'category_name' => 'tools',
'exclude' => '6'
);
Finally, we find the option search
which accepts a string to search links, URLs, names and descriptions. The example below will get all the links containing the word ‘sitepoint’ in the previously listed fields.
$args = array(
'search' => 'sitepoint'
);
Note that you can combine this option, for example, to only search in some categories. The search is case-insensitive.
In Conclusion
Managing a list of links is easy with the WordPress Links Manager. By default, the CMS provides us some useful tools but now, with the API we’ve explored, you can create your own tools.
For example, you can integrate a carousel into your theme, with two images per link: one in background, one in foreground, positioned by the user, using a parameter stored in the link’s notes. You can now do whatever you want, as you now know how to create your own forms.
Frequently Asked Questions about Mastering the WordPress Links Manager API
What is the WordPress Links Manager API and why is it important?
The WordPress Links Manager API is a powerful tool that allows developers to manage links within their WordPress sites. It provides a set of functions and methods to add, update, delete, and retrieve links. This API is crucial because it helps in organizing and managing links efficiently, which can significantly improve the user experience and SEO ranking of your site.
How can I enable the Links Manager in my WordPress site?
By default, the Links Manager is hidden in new WordPress installations. To enable it, you need to install and activate the Link Manager plugin. Once activated, the ‘Links’ option will appear in your WordPress admin panel.
How can I add a new link using the Links Manager API?
To add a new link, you can use the wp_insert_link()
function. This function accepts an array of link data as a parameter and returns the ID of the newly created link.
How can I update an existing link using the Links Manager API?
To update an existing link, you can use the wp_update_link()
function. This function accepts an array of link data, including the link ID, as a parameter.
How can I delete a link using the Links Manager API?
To delete a link, you can use the wp_delete_link()
function. This function requires the ID of the link you want to delete as a parameter.
How can I retrieve a link using the Links Manager API?
To retrieve a link, you can use the get_bookmark()
function. This function requires the ID of the link you want to retrieve as a parameter.
What are the best practices for managing links in WordPress?
Some best practices include categorizing your links, using descriptive link text, regularly checking for broken links, and using the Links Manager API for efficient link management.
Can I use the Links Manager API to manage external links?
Yes, the Links Manager API can be used to manage both internal and external links on your WordPress site.
What is the difference between the Links Manager API and other link management tools?
The Links Manager API is a built-in feature of WordPress, which means it’s fully integrated with your site and doesn’t require any additional plugins. Other link management tools may offer additional features, but they may also require additional setup and configuration.
How can I troubleshoot issues with the Links Manager API?
If you’re experiencing issues with the Links Manager API, make sure you’re using the correct function names and parameters. Check the WordPress Codex for detailed information on each function. If you’re still having trouble, consider reaching out to the WordPress community for support.
Currently a math student, Jérémy is a passionate guy who is interested in many fields, particularly in the high tech world for which he covers the news everyday on some blogs, and web development which takes much of his free time. He loves learning new things and sharing his knowledge with others.