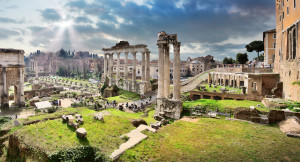
Have you ever wanted to set up a forum for your website? There are tons of solutions out there that you can use for this task. However, what about a forum that is integrated directly into your Rails app? One that can be modified the way you want? A while back, I tried to answer exactly the same question and found that there are only a few forum gems available.
Searching for a Perfect Forum
First of all, there is Altered Beast. It used to be somewhat popular, however it was last updated 5 years ago and it does not support even Rails 3.
Forum Monster seems to be a better option. It provides a handful of features and was last updated in July 2013. However, it is not being actively maintained – there are only three commits in 2013 and no commits in 2014.
I also found Savage Beast (seems like forum developers love monsters) but it was last updated in 2009, so no dice.
The last solution I found was rBoard but its homepage says that the project is no longer maintained as it was transferred to Forem. So,I visited Forem’s page and… EUREKA! Forem appeared to be a full-fledged forum engine with an active (well, not very active community) and a lot of useful features, including:
- Basic operations (creating/updating/deleting categories/forums/topics)
- Locking/pinning/hiding topics
- Replying and quoting posts
- Managing user groups and assigning moderators
- Text formatting with pluggable formatters, code highlighting, and an ability to use smileys
- Integration with Gravatar
- Theming (with an ability to create custom themes)
- Permissions system
- Translation to a variety of languages
- Ability to integrate with RefineryCMS.
Forem was created (and is primarily maintained) by Ryan Bigg and (at the time of writing) has more than 1300 commits.
In this article, I will demonstrate how to set up Forem and point out some gotchas.
The working demo can be found at http://sitepoint-forem.herokuapp.com.
The source code is available on GitHub.
Some Gotchas (As Promised)
Well, I hope that you’re excited about the features Forem provides and are eager to start integrating it in your app. However, before we get there, I feel compelled to warn you about the problems you might face.
First of all, understand that Forem has no stable version at the moment. It is being actively developed, so new features might be added and old features may be removed or altered without notice. There is a Roadmap available, but it is really hard to say when version 1.0 will be out.
Also, Forem has some issues that are not yet resolved (some of them are pretty serious) and you may discover new ones, as well. This probably means that Forem is not an option for beginners (however, I encourage you to give it a try.)
Furthermore, Forem currently provides only a single theme which is based on Bootstrap. This theme is partially broken, so you will surely need to tweak some styles or use your own theme.
However, all of these problems can be coped with and Forem is still a very cool forum engine with a lot of useful features. Also, at this point, it has no viable alternatives.
Preparing the App
I will use Rails 4.1.1 but you can implement the same solution with Rails 3
Okay, enough chatting, let’s dive into the coding. Start by creating a new app without a default testing suite:
rails new forum -T
Here is the list of the gems that we are going to use for this demo:
gem 'forem', github: "radar/forem", branch: "rails4"
gem 'forem-bootstrap', github: "radar/forem-bootstrap"
gem 'forem-redcarpet', github: "radar/forem-redcarpet"
gem 'devise'
We will also need a gem for pagination, you can choose either will_paginate
or kaminari
, as Forem supports both:
gem 'will_paginate'
# or
gem 'kaminari'
If you’re using Rails 3, specify the rails3
branch like this:
gem 'forem', github: "radar/forem", branch: "rails3"
To begin, we need to set up some authentication. The cool thing about Forem is that it allows you to choose any solution or write your own from scratch. For this article we are going to use Devise, a flexible authentication system created by Plataformatec. If you are not familiar with it, check the guides. To get started, you only need a couple of steps.
Run the Devise generator:
rails generate devise:install
You may want to adjust some settings in initializers/devise.rb (you will definitely want to do so when working with a real app). For the purposes of this demo, the default settings will be okay. When you finish, run another generator to prepare the model:
rails generate devise User
Lastly, run the migrations:
rake db:migrate
Devise ships with a bunch of views for login, register, and other forms that you might want to customize. To do that issue this command:
rails g devise:views
This copies the default views into your app and you can adjust them the way you like.
Installing Forem
At this point, we are ready to install Forem. It comes with a nice generator that will ask you a couple of questions to create an initializer file and migrations:
rails g forem:install
The questions are:
- What is your user class called? (default is
User
) - What is the
current_user
helper called in your app? (default iscurrent_user
)
If you chose Devise and called your model User
, you can safely hit “Enter” for both questions. Forem’s migrations will run automatically, so we are done with the command prompt.
Open your routes file (note that there are a couple of routes already present – they were added by Devise and Forem automatically) and add a root route:
routes.rb
[...]
root to: 'pages#home'
Then create PagesController
and the corresponding pages/home.html.erb
view with no content in it. If you are using Rails 3, do not forget to remove the public/index.html
file.
Now it is time to tweak our layout.
application.html.erb
<% flash.each do |key, value| %>
<%= content_tag(:div, value, class: "alert alert-#{key}") %>
<% end %>
<%= link_to 'Home', main_app.root_path %>
<%= link_to 'Forums', forem.forums_path %>
<header>
<nav>
<% if user_signed_in? %>
<%= link_to current_user.email, main_app.edit_user_registration_path %>
| <%= link_to "Sign out", main_app.destroy_user_session_path, :method => :delete %>
<% if current_user.forem_admin %>
| <%= link_to "Administrate", forem.admin_root_url %>
<% end %>
<% else %>
<%= link_to "Sign up", main_app.new_user_registration_path %>
| <%= link_to "Sign in", main_app.new_user_session_path %>
<% end %>
</nav>
</header>
Note that instead of just using root_path
, I’ve specified main_app.root_path
. This is because Forem is a Rails engine and has its own isolated routing system. Otherwise, it might clash with your app’s routes. So to access Forem’s routes, use forem.route_name
.
Setting Up Forem
The next step is to add a method to your model file:
user.rb
[...]
def forem_name
email
end
The forem_name
method is used to display the user’s name next to his/her post. Devise only provides an email
column, so we use it instead of the name. In your app you might want to add a name
column to the users
table and provide your users a way to change their name.
Also, bear in mind that Forem uses Gravatar to display user avatars, using email to find the avatar. If you do not have email on your users table, you can add another method to the model file and tell Forem where the users’ emails are stored:
user.rb
[...]
def forem_email
email_address
end
Open the initializers/forem.rb file. There are a couple of settings that you can change here. For now, add this piece of code:
initializers/forem.rb
Rails.application.config.to_prepare do
Forem.layout = "application"
end
By default, Forem will use its own layout to display forum pages, but we want to specify our own layout. Please note that the documentation says to use Forem::ApplicationController.layout "application"
but I’ve found an issue with this setting. As such, we have to stick with Forem.layout = "application"
. Hopefully, it will be fixed soon.
Forem also creates a couple of files inside the vendor directory. If your application.js already hooks up jQuery (by default it does), remove the //= require jquery
line from vendor/assets/javascripts/forem.coffee.js. Now modify the forem.css.scss file:
vendor/assets/stylesheets/forem.css.scss
@import 'forem-bootstrap';
This line imports Bootstrap theme for Forem (provided by the forem-bootstrap
gem). The final task to do is require these two files:
javascripts/application.js
[...]
//= require forem
stylesheets/application.css.scss
@import 'forem/base';
Also, if you are on Rails 4, add Forem’s assets for precompilation:
initializer/asset.rb
Rails.application.config.assets.precompile += %w( forem.css forem.js )
Cool, we’ve finished with the basic setup. Now you can launch your server, register a new user, and assign admin rights (to do that open users
table and switch forem_admin
attribute to true
). Try to create some categories, forums, topics – everything should be working. Note that, by default, all created topics are marked as ‘should be moderated’ before they are visible to the users.
Formatters and Smileys
What about formatting of the posts? Thanks to the forem-redcarpet
gem, it should be working fine – even code highlighting is provided. Currently, highlighting is supported by Redcarpet, but I’ve created a pull request proposing to switch to Coderay – you can check it out here.
There are other formatters available, but (as I’ve found out) some of them have problems with quoting – check this issue if you wish to know the details.
Users may also use smileys in their posts provided by emoji. Unfortunately, I’ve found a problem with them when using Rails 4.1 – you can read about it here.
Conclusion
That is all for today, folks! We’ve successfully set up Forem to work with our app in no time. By the way, you can check the real projects that are using Forem here.
If you are having problems while setting up or using Forem, please do not hesitate to open an issue on GitHub providing all the relevant information. Bug fixes and new features are also very welcome, so you can go ahead and clone the Forem repo to start tweaking it right now!
What forum solution do you use for your website? Share your experience in the comments :).
Happy chatting in your newly created forum and see you soon!
Frequently Asked Questions about Forem Rails Forum Engine
How does Forem Rails Forum Engine differ from other forum engines like Thredded?
Forem Rails Forum Engine is a robust, open-source software that is designed to provide a comprehensive solution for creating online communities. Unlike Thredded, which is primarily a message board, Forem offers a more holistic approach to community building. It includes features like moderation tools, social networking, and customizable user profiles. Forem also has a more modern and user-friendly interface compared to Thredded.
Can I customize the look and feel of my forum with Forem Rails Forum Engine?
Yes, Forem Rails Forum Engine offers extensive customization options. You can modify the layout, color scheme, and even the functionality of your forum to suit your specific needs. This level of customization allows you to create a unique and engaging online community that reflects your brand’s identity.
How does Forem Rails Forum Engine handle moderation and community management?
Forem Rails Forum Engine has built-in moderation tools that allow you to manage your community effectively. You can set rules for posting, monitor user activity, and even ban users if necessary. These tools make it easier to maintain a positive and respectful community environment.
Is Forem Rails Forum Engine suitable for large communities?
Absolutely. Forem Rails Forum Engine is designed to handle communities of all sizes. It has a scalable architecture that can accommodate a large number of users without compromising performance. This makes it an ideal choice for large online communities.
How does Forem Rails Forum Engine compare to Reddit’s Rails forum?
While Reddit’s Rails forum is a popular platform for discussing Rails-related topics, Forem Rails Forum Engine offers a more focused and customizable solution for creating online communities. With Forem, you have complete control over the structure and functionality of your forum, which is not possible with Reddit.
What kind of support is available for Forem Rails Forum Engine?
Forem Rails Forum Engine has a strong community of developers who are always ready to help. You can also find extensive documentation online that covers everything from installation to advanced features. This makes it easier to get started and troubleshoot any issues you may encounter.
How does Forem Rails Forum Engine rank in the Ruby Toolbox’s list of forum systems?
Forem Rails Forum Engine is highly regarded in the Ruby community and is often ranked among the top forum systems in the Ruby Toolbox. Its robust features, ease of use, and strong community support make it a popular choice for developers.
Is Forem Rails Forum Engine compatible with other Ruby gems?
Yes, Forem Rails Forum Engine is designed to work seamlessly with other Ruby gems. This allows you to extend the functionality of your forum and integrate it with other applications.
How easy is it to install and set up Forem Rails Forum Engine?
Installing and setting up Forem Rails Forum Engine is straightforward. The process is well-documented and there are plenty of resources available online to guide you through the process.
Can I migrate my existing forum to Forem Rails Forum Engine?
Yes, it is possible to migrate your existing forum to Forem Rails Forum Engine. However, the process can be complex and may require some technical expertise. It’s recommended to consult with a developer or the Forem community for assistance.

Ilya Bodrov is personal IT teacher, a senior engineer working at Campaigner LLC, author and teaching assistant at Sitepoint and lecturer at Moscow Aviations Institute. His primary programming languages are Ruby (with Rails) and JavaScript. He enjoys coding, teaching people and learning new things. Ilya also has some Cisco and Microsoft certificates and was working as a tutor in an educational center for a couple of years. In his free time he tweets, writes posts for his website, participates in OpenSource projects, goes in for sports and plays music.