This series of posts will show you how to create a simple Windows 8 game, using HTML5, JavaScript, WinJS, and CreateJS. The game is based on the XNA sample game “Catapult Wars Lab”. We’ll reuse the assets from that game as we develop a new version for Windows 8 that’s based on web technologies. In this post, we’ll respond to user input and make things a bit more lively by adding sound.
Handling User Input
Of course there are many ways we could have shot aiming work – random, time-based, precision-based (e.g. “click… NOW!”), but in this case we’ll let the user draw a line indicating both direction and speed. First, let’s add new variables near the top ofdefault.js
:
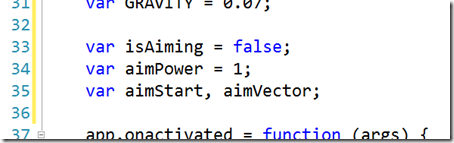
MSPointerDown/Up
to begin and end aiming, plus MSPointerMove
to provide feedback as the user adjusts the aim.
By the way, there’s also great support via MSGesture
for detecting gestures such as tap, double-tap, etc. A good example for handling input and gestures is the “BallInEight” sample on MSDN.
These events are used by pointing to listener functions, called when they fire. Let’s take care of that now, adding near the top of the initialize()
function:
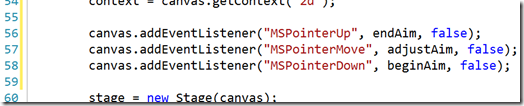
update()
and fireShot()
:
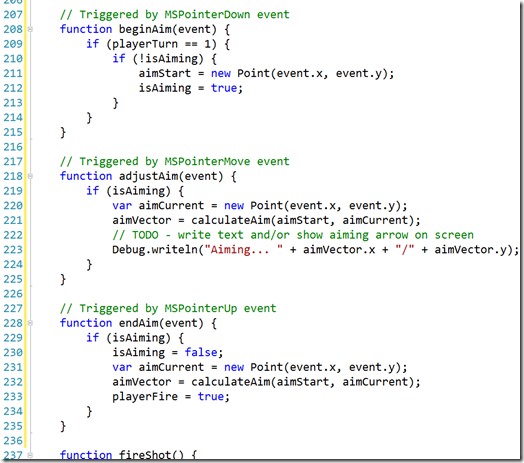
adjustAim()
function. Go ahead and try adding that – from earlier parts, we’re already using the very same techniques you’ll need.
And a function to help us calculate the aim:
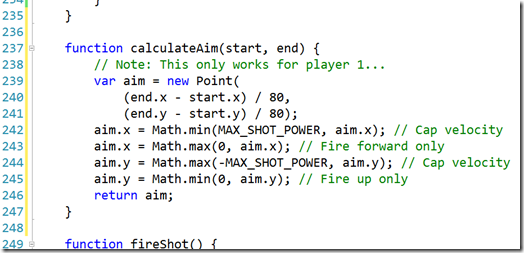
- Lines 239-241 – Create a new
Point
from the distance from the start to endPoint
s. Scale it down to a good velocity to apply per frame/update. - Lines 242 & 244 – Make sure the
x
andy
are limited so the shot doesn’t simply disappear off the screen, going too quickly - Lines 243 & 245 – Make sure the shot is going toward the enemy … more or less.
update()
to have player 1 always fire randomly. Now we can replace that to use the new aimVector
:
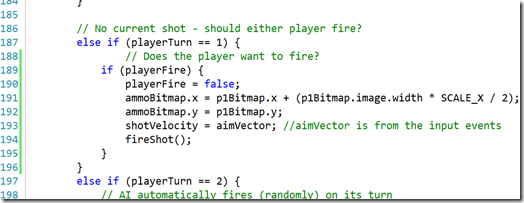
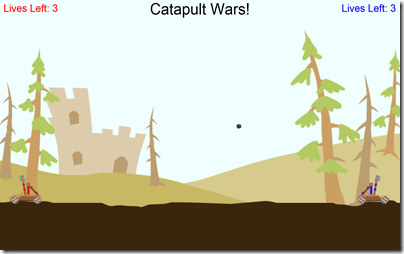
“I must be hearing things!” – Adding Sound
Our job as a game dev isn’t done until we have some sound. We’ve already added the sound files back in Part 2 so let’s take the next step by loading them. First, a few variables indefault.js
to keep things tidy:
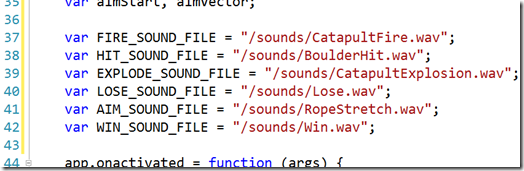
PreloadJS
to ensure our resources are ready when the game starts? We can use the same approach with sounds, so let’s add them to the manifest:
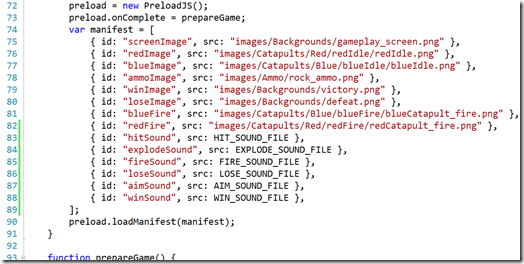
audio
elements. Long story short, if you simply create one audio instance per sound file, you’ll probably run into clipping as one sound fails to play before the prior play of that instance completes. There are several approaches for dealing with this (for example, see “HTML5 Audio and JavaScript Control”), but we’ll do the simple & inefficient thing of using one instance each time we play a sound.
Note, we will not be using SoundJS (another part of CreateJS) in this example, but of course you’re welcome to take it for a spin!
Add the playSound(file)
helper function:
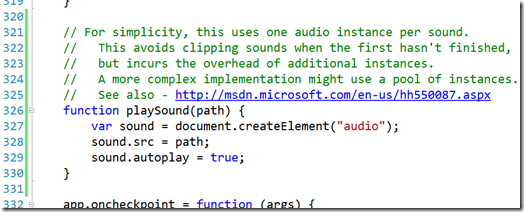
fireShot()
:
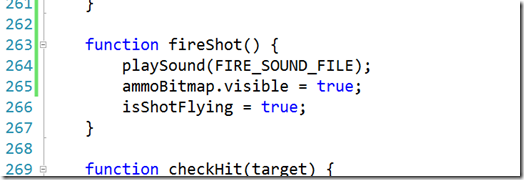
processHit()
:
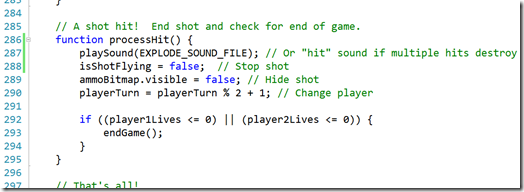
endGame()
:
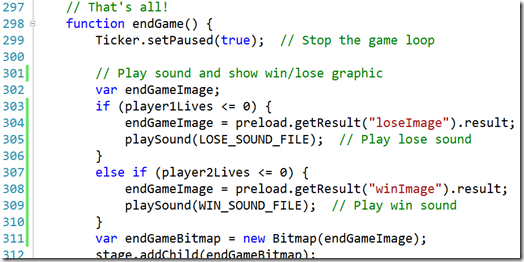
Game On… And On… And On…
Congratulations! You’ve made a game! We’ve covered a lot of ground in these posts, but like anything, there’s plenty of other things to try. Why not take a stab at some of them?- Screen adjustments – Portrait/landscape, snapped/filled. A great chance to learn about CSS Media Queries!
- Improving Touch/Gesture support
- Sprites/animations – Shot hit/miss, catapult pullback/fire, catapult destroyed
- Gameplay dynamics – Adding wind, new scenes, new catapult features/upgrades, choices in ammo, etc.
- Add a mountain and moving clouds to background (the assets are already there)
- Add some intelligence to the currently very artificial intelligence
- Consider using a live tile – Maybe show the player’s last/high score?
Frequently Asked Questions about Creating a Simple Windows 8 Game with JavaScript
How can I add more complex movements to my game characters?
To add more complex movements to your game characters, you can use the ‘requestAnimationFrame’ method. This method allows you to create smoother animations by calling your animation function before the browser’s next repaint. You can use this method to update the position of your game characters based on user input or game logic. For example, you can use it to make a character jump when the user presses a specific key.
How can I add sound effects to my game?
Adding sound effects to your game can enhance the gaming experience. You can use the ‘Audio’ object in JavaScript to play sound files. To play a sound, you need to create a new Audio object and call the ‘play’ method. You can also control the volume, loop the sound, and stop the sound using the ‘volume’, ‘loop’, and ‘pause’ methods respectively.
How can I make my game responsive to different screen sizes?
To make your game responsive, you can use the ‘window.innerWidth’ and ‘window.innerHeight’ properties in JavaScript. These properties return the width and height of the browser window respectively. You can use these properties to adjust the size and position of your game elements based on the size of the browser window.
How can I add multiplayer functionality to my game?
Adding multiplayer functionality to your game can be quite complex. You will need to use WebSockets to enable real-time communication between the players. You will also need to handle issues such as latency and synchronization.
How can I optimize my game for better performance?
There are several ways to optimize your game for better performance. One way is to use the ‘requestAnimationFrame’ method for your animations. This method is more efficient than ‘setInterval’ or ‘setTimeout’ because it pauses the animation when the user switches to another tab. Another way is to minimize the use of global variables and closures, as they can take up a lot of memory.
How can I add touch controls to my game?
To add touch controls to your game, you can use the ‘touchstart’, ‘touchmove’, and ‘touchend’ events in JavaScript. These events are fired when the user touches the screen, moves their finger, and lifts their finger respectively. You can use these events to control the movement of your game characters.
How can I publish my game on the Windows Store?
To publish your game on the Windows Store, you need to create a Windows Store developer account. Once you have an account, you can submit your game for certification. If your game passes the certification process, it will be published on the Windows Store.
How can I add in-app purchases to my game?
To add in-app purchases to your game, you need to use the Windows.ApplicationModel.Store namespace. This namespace provides the classes and methods you need to implement in-app purchases.
How can I add achievements to my game?
To add achievements to your game, you can use the Windows.ApplicationModel.UserDataAccounts namespace. This namespace provides the classes and methods you need to implement achievements.
How can I test my game on different devices?
To test your game on different devices, you can use the Windows Device Portal. This tool allows you to remotely debug and test your game on a Windows device.
Chris Bowen is a Principal Technical Evangelist with Microsoft, based in the Boston area and specializing in Windows 8 development. An architect and developer with over 19 years in the industry, he joined Microsoft after holding senior technical positions at companies including Monster.com, VistaPrint, and Staples. He is coauthor of two books (with Addison-Wesley and WROX) and holds an M.S. in Computer Science and a B.S. in Management Information Systems, both from Worcester Polytechnic Institute.