Everyone knows how cool LESS is. If you’re not, then here’s the elevator speech. LESS extends CSS with dynamic behavious such as variables, mixins, namespaces and functions, it makes CSS easy to work with. Now, it’s important to remember it doesn’t code your CSS for you – it isn’t a magic CSS editor. You still need to know how to work with CSS. It allows you to write CSS once and use it in multiple places. This is something I’ve wanted for a long time and now it’s here. There are other libraries which perform similar functions, such as SASS, but I’ll focus on LESS as that’s what I’m familiar with. I’m going to be concentrating on how to use this with ASP.NET. I’m going to be using Visual Studio 2010 for this demonstration, as I had a few issues using LESS with Visual Studio 11.
Running LESS On the Client
LESS can be run purely on the client or from the server. To run it on the client is a simple three step process.- Add a reference to the LESS JavaScript file
- Update the rel value in the LINK tag to rel=”stylesheet/less”
- Add a new .less file to your project and reference that in your LINK tag
<link href="styles/my.less" rel="stylesheet/less" />
<script src="http://lesscss.googlecode.com/files/less-1.3.0.min.js"></script>
I’m referencing a file called my.less, so let’s define some LESS code to ensure this is working.
@back-color: #000;
@font-color: #fff;
body {
background-color: @back-color;
font-size: .85em;
font-family: "Trebuchet MS", Verdana, Helvetica, Sans-Serif;
margin: 0;
padding: 0;
color: @font-color;
}
I’ll skip over the syntax for now, but if you run the website and look in the developer tools, you’ll see that LESS code is being served as valid CSS.
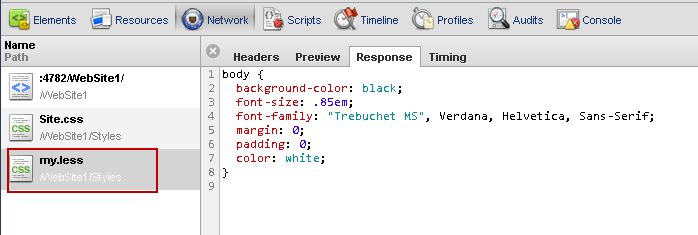
Running LESS On the Server
There are several ways to install LESS on the server. The easiest approach is via NuGet. There’s a package called dotless. Install it with the following command inside Visual Studio.
<link href="styles/my.less" rel="stylesheet" />
The package has also added some entries to your web.config file. There’s a new configSection defined.
<configSections>
<section name="dotless" type="dotless.Core.configuration.DotlessConfigurationSectionHandler, dotless.Core" />
</configSections/>
<dotless minifyCss="false" cache="true" web="false" />
And a new HTTP handler has been added to cater for .less requests.
<system.webServer>
<handlers>
<add name="dotless" path="*.less" verb="GET" type="dotless.Core.LessCssHttpHandler,dotless.Core" resourceType="File" preCondition="" />
</handlers>
</system.webServer>
The nice feature about dotless is that it can automatically minify the CSS for you via the minifyCss attribute. If you update that to true and run the website now, you’ll see the minified CSS.
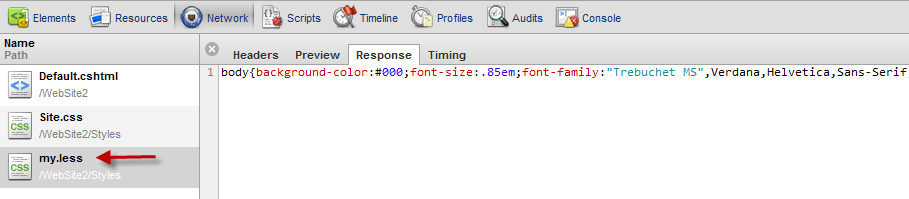
When To Use It?
I think LESS is great for development, but when you need your site to run as fast as possible, you don’t want to transform each .less request on the fly. This is why I’d recommend using this only during development. The good news is when you install dotless, it installs the dotless compiler. This can be in the packagesdotless1.3.0.0Tool folder in your website folder. You can add this to your pre-build event from the build properties tab. “$(SolutionDir)packagesdotless.1.3.0.0tooldotless.Compiler.exe” “$(ProjectDir)contentmy.less” “$(ProjectDir)contentmy.css”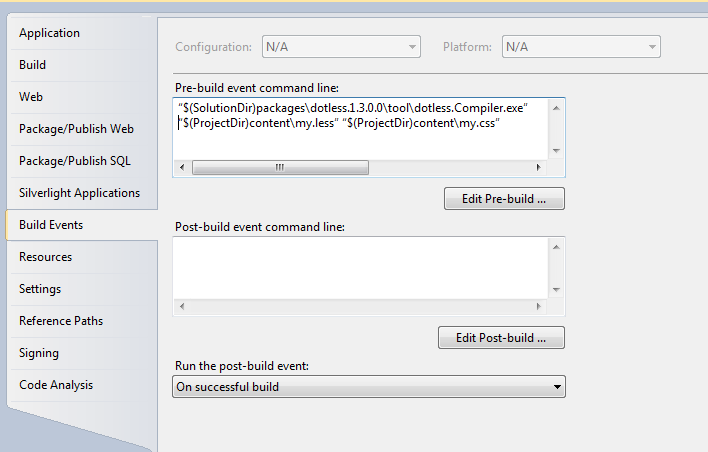
LESS Syntax
I haven’t covered any of the syntax in this article. I wanted to focus on LESS with ASP.NET. Ivaylo Gerchev has a good article on the syntax and that can be found here. I think LESS is a must tool to have during development. It will make your life easier when coding CSS.Frequently Asked Questions (FAQs) about Combining LESS with ASP.NET
What is the benefit of combining LESS with ASP.NET?
Combining LESS with ASP.NET can significantly enhance your web development process. LESS, a dynamic preprocessor style sheet language, allows you to use techniques like variables, nesting, and mixins, making your CSS more maintainable, themable, and extendable. When used with ASP.NET, a popular framework for building web apps, you can leverage these benefits to create more efficient, scalable, and robust web applications.
How do I install the Web Compiler in Visual Studio for LESS and ASP.NET integration?
To install the Web Compiler in Visual Studio, go to Extensions > Manage Extensions > Online, then search for ‘Web Compiler’. Click on ‘Download’ and restart Visual Studio to complete the installation. This extension will allow you to compile LESS files directly in your ASP.NET projects.
What is the role of the Web Compiler in LESS and ASP.NET integration?
The Web Compiler plays a crucial role in LESS and ASP.NET integration. It compiles your LESS files into CSS directly within your ASP.NET projects. This means you can write in LESS, with all its added functionality, and the Web Compiler will automatically convert it into CSS that the browser can interpret.
How do I resolve issues with the Web Compiler?
If you encounter issues with the Web Compiler, first ensure that you have the latest version installed. If the problem persists, check the error message for clues about what might be wrong. You can also refer to the Web Compiler’s GitHub issues page for solutions to common problems.
What does ‘netless’ mean?
Netless’ is not directly related to the topic of combining LESS with ASP.NET. In a general context, ‘netless’ could mean without a net or network. However, in the context of web development, it doesn’t have a specific meaning.
How do I use variables in LESS?
Variables in LESS are defined with an @ symbol. For example, @color: #4D926F; You can then use this variable anywhere in your LESS file, like this: #header { color: @color; } This makes your LESS code more maintainable and easier to update.
Can I use nesting in LESS?
Yes, LESS supports nesting, which can make your stylesheets more readable and easier to maintain. For example, instead of writing separate selectors for #header h1 and #header p, you can nest them like this: #header { h1 { … } p { … } }
What are mixins in LESS and how do I use them?
Mixins in LESS allow you to reuse whole classes or properties. You can define a mixin like a class, and then include it in another class by simply calling its name. For example: .rounded-corners (@radius: 5px) { border-radius: @radius; } .box { .rounded-corners; }
Can I use LESS with other web development frameworks?
Yes, LESS is not limited to ASP.NET. It can be used with any web development framework that supports CSS, as it ultimately compiles down to CSS. This includes frameworks like Django, Flask, Ruby on Rails, and many others.
How does combining LESS with ASP.NET improve scalability?
Combining LESS with ASP.NET can improve scalability by making your stylesheets more maintainable and easier to update. This is particularly beneficial for large projects where the CSS can become complex and difficult to manage. By using LESS, you can use variables, mixins, and nesting to keep your CSS organized and easy to modify, which is crucial for scaling a project.
Malcolm Sheridan is a Microsoft awarded MVP in ASP.NET, ASPInsider, Telerik Insider and a regular presenter at conferences and user groups throughout Australia and New Zealand. Follow him on twitter @malcolmsheridan.