A few code snippets to Get Client IP Using JavaScript/jQuery.
1. One way
This one gives you longitude/latitude and timezone. Try it!$(document).ready( function() { $.getJSON( "https://smart-ip.net/geoip-json?callback=?", function(data){ alert( data.host); } );});
Returns:
?(
{
source: "smart-ip.net",
host: "14.200.158.65",
lang: "en",
countryName: "Australia",
countryCode: "AU",
city: "South Sydney Municipality",
region: "New South Wales",
latitude: "-33.9000",
longitude: "151.2000",
timezone: "Australia/NSW"
}
)
2. Two way
This one provides JSON. Try it!function myIP() {
if (window.XMLHttpRequest) xmlhttp = new XMLHttpRequest();
else xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
xmlhttp.open("GET"," http://api.hostip.info/get_html.php ",false);
xmlhttp.send();
hostipInfo = xmlhttp.responseText.split("n");
for (i=0; hostipInfo.length >= i; i++) {
ipAddress = hostipInfo[i].split(":");
if ( ipAddress[0] == "IP" ) return ipAddress[1];
}
return false;
}
// console.log(myIP());
Returns:
{
country_name: "UNITED STATES",
country_code: "US",
city: "(Unknown city)",
ip: "14.200.158.65"
}
3. Three way
Try it!https://l2.io/ip.js
Syntax : https://l2.io/ip
Diplay Client IP address
--> x.x.x.x
Syntax :
Display Client IP address in your HTML page using javascript
--> document.write('x.x.x.x');
Syntax :
Set javascript variable "myip" to client IP address
--> myip = "x.x.x.x";
Frequently Asked Questions (FAQs) about Getting Client IP using jQuery
What is the significance of getting a client’s IP address using jQuery?
The client’s IP address is a unique identifier that can be used to track the user’s location, device, and other details. This information can be useful for various purposes such as personalizing content, implementing location-based features, or for security reasons like detecting suspicious activities or blocking certain IP addresses. jQuery, being a fast, small, and feature-rich JavaScript library, makes the process of obtaining the client’s IP address relatively simple and efficient.
Is it possible to get a client’s IP address using only jQuery?
jQuery itself does not have a built-in method to get the client’s IP address. However, it can be used in conjunction with AJAX and a server-side script to retrieve this information. The server-side script can obtain the client’s IP address, and jQuery can then use an AJAX request to retrieve this information from the server.
How can I use jQuery and AJAX to get a client’s IP address?
You can use jQuery’s AJAX method to send a request to a server-side script. This script can then return the client’s IP address, which can be accessed in the success callback of the AJAX request. Here’s a simple example:$.get("getIP.php", function(data) {
alert("Your IP is " + data);
});
In this example, “getIP.php” is a server-side script that returns the client’s IP address.
Can I get a client’s IP address without using a server-side script?
Yes, you can use a public IP address API service to get the client’s IP address. These services can return the client’s IP address directly, so you don’t need a server-side script. Here’s an example using the ipify API:$.getJSON("https://api.ipify.org?format=jsonp", function(data) {
alert("Your IP is " + data.ip);
});
Is it possible to get a client’s local IP address using jQuery?
Unfortunately, it’s not possible to get a client’s local IP address using only jQuery or JavaScript due to security reasons. The local IP address is considered private information, and browsers do not provide a way to access it directly from JavaScript or jQuery.
Can I use the client’s IP address to get their location?
Yes, you can use a geolocation API service to get the client’s location based on their IP address. These services can return information such as the client’s city, region, country, and even latitude and longitude coordinates.
Is it always accurate to use the IP address to determine the client’s location?
While using the IP address can give you a general idea of where the client is located, it’s not always 100% accurate. The accuracy can vary depending on several factors, including whether the client is using a VPN or proxy, and the accuracy of the geolocation database being used.
Is it legal to get and use a client’s IP address?
The legality of getting and using a client’s IP address can vary depending on the laws of the country where you or the client are located. In general, it’s important to respect the client’s privacy and to use their IP address responsibly. It’s also a good practice to inform the client if you are collecting their IP address and how you plan to use it.
Can I block certain IP addresses from accessing my website?
Yes, you can use the client’s IP address to block certain IP addresses from accessing your website. This can be done on the server-side, for example, by configuring your web server or using a server-side script.
Can a client change or hide their IP address?
Yes, a client can change or hide their IP address using various methods such as using a VPN, proxy, or TOR network. This can make it more difficult to accurately determine the client’s location or to block specific IP addresses.
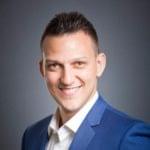
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.