WordPress has a big market share of the web. REST APIs are a growing technique and a big opportunity for developers. Knowing how to create APIs and how to consume them gives you a great advantage. A REST API can be consumed everywhere. On mobile applications, on front-end (web apps) or any other devices that have access on the net. Maybe your customer already has a site that is running on WordPress and also wants a mobile application. You can create the API using custom code and relying on the database but that can cause a lot of frustration. Maybe they’re in a hurry and want the API as soon as possible. In WordPress, it is as simple as installing a plugin. In this article, we will give a general overview of the JSON REST API plugin. We will cover how this plugin works and the basic philosophy of the REST architecture.
Preparation
Grab the latest version of JSON REST API on WordPress plugin directory. Install and activate it. Now you have a REST API ready to use. You need a REST Client to test and explore it. There are many tools for that job. Don’t limit yourself to just these four tools. There are many other REST Clients. The first one is cURL. It is a command line tool that can send different requests to the given endpoint. It is not only REST API related but it is a general HTTP Request tool. Many test examples on other articles are made using this tool. It’s the universal HTTP tool that can be translated to any programming language. One of the tools I usually use is the PHPStorm REST Client. As I mainly work with this IDE when developing, it is easier for me using a tool that I can access faster. Many other IDE-s come with REST Client integrated to make REST API developing easier and faster. The third one and the one that I highly recommend is Postman. It is the easiest and more intuitive REST client I have ever worked with. Unfortunately, is only available as a Chrome extension. If you are using Firefox there is a nice extension called RESTClient.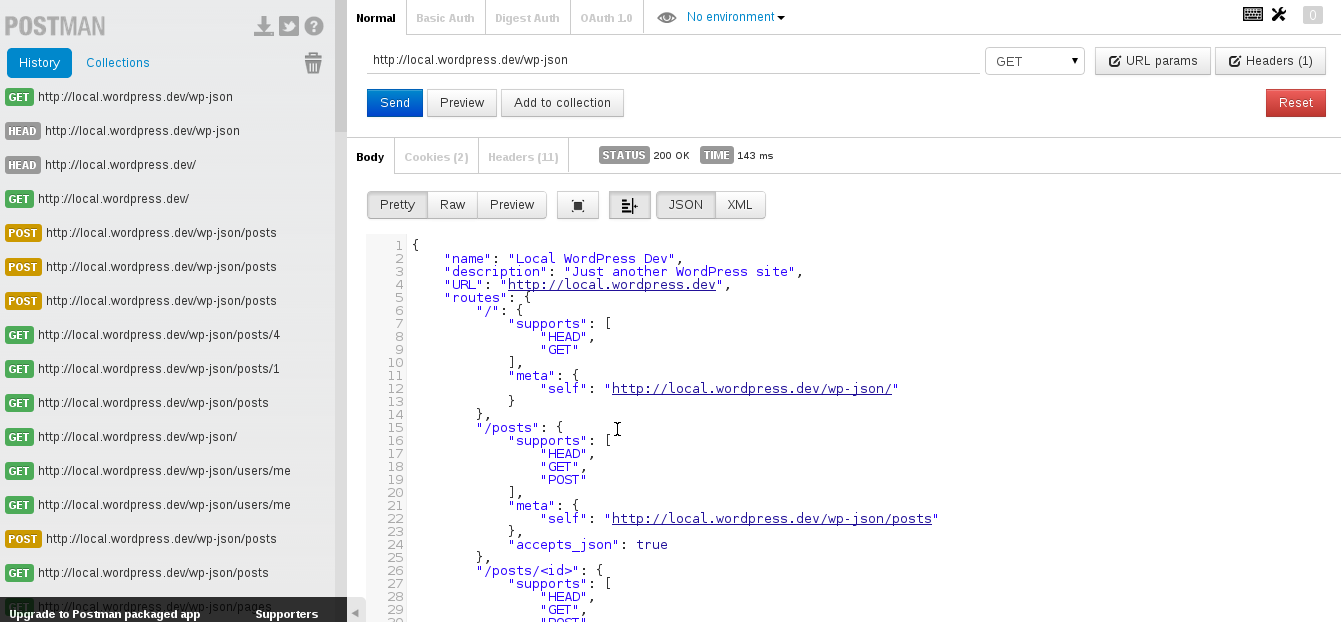
Exploring the REST Architecture
The first thing you have to do is to check if this plugin works, where is its endpoint, the base URL that holds all the information about the schema, all the Resources (Collections) and the routes. The plugin adds a new field called “`Link`” on the header so check for that in the header of the Response. If you use Postman or any other GUI clients just inspect the header. If you use cURL execute this command to your homepage:curl -I http://yoursite.com/
The header option grabs only the header. In my case the endpoint is local.wordpress.dev/wp-json
. If you left the permalink to it’s default value on the settings, then you may have the link value something like this: http://local.wordpress.dev/?json_route=/
.
It changes only the rewrite rules but not any other essential changes. Everything is the same in the two cases.
The index endpoint
(or API endpoint) is the starting point. You can explore the whole API from there. It gives you all the information about the API. It gives information about the Collections, Entities and how they are mapped to routes. Make a GET request to your endpoint and see what it returns. On REST Clients it’s easy because you are dealing with GUI interface. Let’s see how is done using cURL.
curl http://local.wordpress.dev/wp-json/
In my case it returns a big JSON file that looks like this.
{
"name": "Local WordPress Dev",
"description": "Just another WordPress site",
"URL": "http://local.wordpress.dev",
"routes": {
"/": {
"supports": [
"HEAD",
"GET"
],
"meta": {
"self": "http://local.wordpress.dev/wp-json/"
}
},
"/posts": {
"supports": [
"HEAD",
"GET",
"POST"
],
"meta": {
"self": "http://local.wordpress.dev/wp-json/posts"
},
"accepts_json": true
},
"/posts/<id>": {
"supports": [
"HEAD",
"GET",
"POST",
"PUT",
"PATCH",
"DELETE"
],
"accepts_json": true
},
"/posts/<id>/revisions": {
"supports": [
"HEAD",
"GET"
]
}
},
"authentication": [],
"meta": {
"links": {
"help": "https://github.com/WP-API/WP-API",
"profile": "https://raw.github.com/WP-API/WP-API/master/docs/schema.json"
}
}
}
Actually the response is too long so I removed most of it. The index route (endpoint) gives gives information about the title of the site, the description and it’s URL. All the Routes that you can find on the API are also mapped in the ‘routes’ field. posts, users, media, pages are Collections. Collections are a group of Entities. An Entity is a single post or a single page with its ID.
Let’s say we want to see the latest posts. The endpoint for the Collection is api_endpoint/posts
. This returns the latest posts. The Entity is api_endpoint/posts/ID
with the given ID as an argument. Using cURL, the command would be:
curl http://local.wordpress.dev/wp-json/posts
And:
curl http://local.wordpress.dev/wp-json/1
Retrieving data is a good thing but there are times when we want to post data.
POST, UPDATE, DELETE
I want to share some problems that I got when tried to post or delete data. First, you need to authenticate yourself. There are three ways you can authenticate. The first one is using the cookies. This is one method not widely used when working with APIs. When a user navigates on the web, pages tend to store data on users side. The cookie method here is a bit different by using the nonces method. The second one is the OAuth method. This plugin implements the version 1.0a of OAuth. This method is widely used. Google, Facebook, Twitter and Flickr use OAuth for third party authentication. OAuth is in version two but they have chosen to use version 1.0a. There is a big debate for the version two. Some big companies didn’t shift in version two for different reasons but mainly concerned about security. The third one is the Basic Authentication. Using this method you have to send your username and password each time you make a request. Client tools for REST testing usually have this method implemented themselves. This method is mainly used when developing. Is rarely used on production. On production consider using OAuth. Unfortunately, the Basic Authentication plugin is not updated to often. They concentrated all their efforts on the OAuth plugin. This plugin can’t be found on wordpress.org but only on it’s GitHub repository. You have to manually install it. On the plugin directory execute this command:git clone https://github.com/WP-API/Basic-Auth basicAuth
Alternatively visit the GitHub page and download the plugin and install it manually via FTP.
Then go to the dashboard and activate it. This plugin has some problems with non-Apache servers. As I mainly use WordPress VVV which is a Vagrant installation, it has problems because VVV comes with nginx. Change the permalink configuration if yours is default. That worked for me.
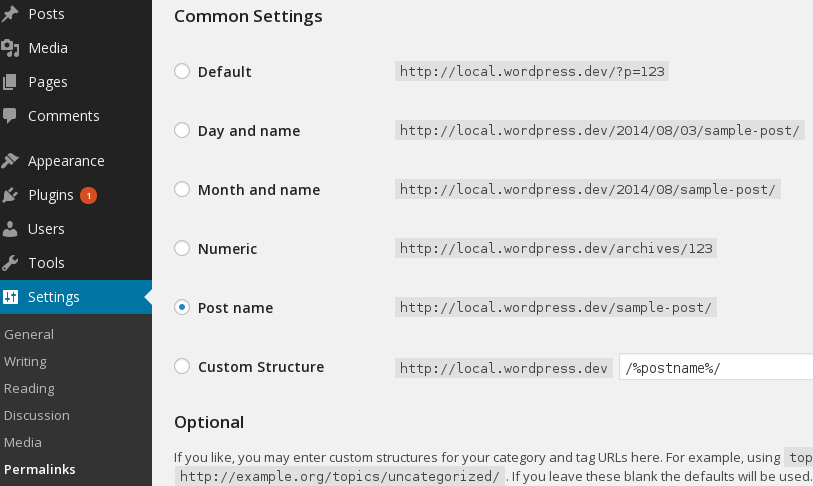
[
{
"code": "json_not_logged_in",
"message": "You are not currently logged in."
}
]
Let’s try to make a request via cURL and also include the Basic Auth method:
curl --user admin:password http://local.wordpress.dev/wp-json/users/me
The --user
option makes the admin:password
argument which is basically just 64 encoding of that plain text. cURL builds itself the Request headers for the Basic Auth by adding an additional field. This is what it adds in my case:
Authorization: Basic YWRtaW46cGFzc3dvcmQ=
This is what is returned when I try to access my profile using the given credentials.
{
"ID": 1,
"username": "admin",
"name": "admin",
"first_name": "",
"last_name": "",
"nickname": "admin",
"slug": "admin",
"URL": "",
"avatar": "https://gravatar.com/avatar/06e92fdf4a9a63441dff65945114b47f?s=96",
"description": "",
"registered": "2014-07-17T22:59:59+00:00",
"roles": [
"administrator"
],
"capabilities": {
"switch_themes": true,
"edit_themes": true,
"activate_plugins": true,
"edit_plugins": true,
"edit_users": true,
"edit_files": true,
"manage_options": true,
"moderate_comments": true,
"manage_categories": true,
"manage_links": true,
"upload_files": true,
"import": true,
"administrator": true
},
"email": false,
"meta": {
"links": {
"self": "http://local.wordpress.dev/wp-json/users/1",
"archives": "http://local.wordpress.dev/wp-json/users/1/posts"
}
}
}
Let’s try to delete one post. To delete one post we must know it’s URL. It’s URL is api_endpoint/posts/ID
. Replace the ID with 1 or any other post ID that you want. Don’t forget that you should use authentication to delete something.
curl --user admin:password -X DELETE http://local.wordpress.dev/wp-json/posts/4
If using Postman or similar send the URL above (http://local.wordpress.dev/wp-json/posts/4) and select the ‘Delete’ request method from the dropdown list next to the URL field.
And this is what I get back:
{
"message":"Deleted post"
}
Posting a new record (post for example) is easy too. Create a new JSON file with two fields. One for the title and one for the content.
{
"title": "This is a post",
"content_raw": "This is some content"
}
Send that new data to the Collection of that Entity that we want to create. Remember that when posting new Entity, you should post it in the Collection endpoint. Here we used Basic Auth again. -X POST
makes this request a POST Request. --data
captures a file that is in that folder and sends it as raw data.
curl --user admin:password -X POST http://local.wordpress.dev/wp-json/posts --data @data.json
Further Exploration
Don’t limit yourself. WordPress REST API team has also some other tools that are tightly integrated with this plugin. They have a Client Cli, Client JS and a Client PHP to work with your API right out of the box. Also head over their documentation for deeper technical information. Knowing how to use this plugin is one thing but the best advice I can give in this case is: ‘don’t limit yourself’. There is so much information out there about REST. Here on SitePoint we have a nice series about building a REST API from scratch by Vito Tardia.Conclusion
Creating a REST API on WordPress is easy. You don’t have to create one from scratch if you’re using WordPress. As a matter of fact, the index end-point tells you everything about this plugin and how to use it. We covered Collections, Entities and how to manipulate some data using different verbs (GET, POST, PUT, DELETE). An important aspect when developing is also authentication so we covered this as well. Consider OAuth 1.0a when developing for production. You can also use Basic Auth but OAuth is already tested and backed by a number of big companies. What do you think about REST APIs on WordPress? Does it compete with the hand crafted APIs? Do you know any other WordPress plugins about REST? Please let us know in the comments below.Frequently Asked Questions (FAQs) about WordPress JSON REST API
What is the difference between WordPress JSON REST API and other APIs?
The WordPress JSON REST API is a specific API that allows developers to interact with WordPress data in a more flexible and efficient way. Unlike other APIs, it uses JSON (JavaScript Object Notation), a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. This makes it a powerful tool for developers who want to create, read, update, and delete WordPress content from client-side JavaScript or from external applications.
How can I extend the WordPress REST API?
Extending the WordPress REST API involves adding new routes and endpoints, which allow you to interact with additional types of data. This can be done by using the register_rest_route() function, which allows you to specify the URL of the new route, the methods it responds to, and the callback function that should be used to handle requests.
What are the benefits of using WordPress REST API?
The WordPress REST API offers several benefits. It allows developers to interact with WordPress data in a more flexible and efficient way, making it easier to create, read, update, and delete content. It also enables the creation of more dynamic and interactive web applications, as it allows for the retrieval and manipulation of data from client-side JavaScript or from external applications.
How secure is the WordPress REST API?
The WordPress REST API includes several security features to help protect your data. It uses a permissions callback to check if a user has the right to perform a certain action, and it supports nonces for CSRF protection. However, like any API, it’s important to use it responsibly and follow best practices for security.
Can I use the WordPress REST API with other programming languages?
Yes, the WordPress REST API can be used with any programming language that can send HTTP requests and parse JSON. This includes languages like Python, Ruby, and Java, among others.
How can I troubleshoot issues with the WordPress REST API?
Troubleshooting issues with the WordPress REST API can involve several steps. First, check the HTTP status code returned by the API to see if it indicates an error. You can also use the ‘rest_pre_serve_request’ filter to examine the server’s response before it’s sent.
What are some common use cases for the WordPress REST API?
The WordPress REST API can be used for a wide range of applications, from creating mobile apps that interact with your WordPress site, to integrating your site with third-party services, to building fully-fledged web applications using WordPress as a backend.
How can I optimize the performance of the WordPress REST API?
Optimizing the performance of the WordPress REST API can involve several strategies, such as caching responses, limiting the number of requests, and using the ‘fields’ parameter to limit the amount of data returned by the API.
Can I use the WordPress REST API to create custom post types?
Yes, the WordPress REST API supports custom post types. You can register a custom post type with the ‘show_in_rest’ parameter set to true, which will make it available via the API.
How can I authenticate requests to the WordPress REST API?
The WordPress REST API supports several methods of authentication, including cookie authentication, OAuth, and basic authentication. However, it’s important to note that some methods may not be suitable for all use cases, and you should choose the method that best fits your needs.

Aleksander is young developer who loves to play with the newest web technologies. In his free time, he reads about PHP, Firefox OS or experiments with a new language. Currently, his main interests are PHP design patterns, laravel, dart and cloud.