The React useEffect
hook is a powerful tool in the React developer’s arsenal. It allows you to perform side effects in your functional components, such as data fetching, subscriptions, or manually changing the DOM. This article aims to provide a comprehensive understanding of the useEffect
hook, its usage, and best practices.
What is React useEffect
?
The useEffect
hook is a function provided by React that allows you to handle side effects in your functional components. Side effects are any operations that don’t involve the rendering of components, such as API calls, timers, event listeners, and more.
Before hooks were introduced in React 16.8, side effects were handled in lifecycle methods in class components. But with the introduction of hooks, you can now use side effects in your functional components with the useEffect
hook.
Basic syntax of useEffect
The useEffect
hook takes two arguments: a function where you define your side effect and a dependency array. The function runs after every render, including the first one, unless you specify a dependency array.
The dependency array is a way to tell React when to run your effect. If you pass an empty array ([]
), the effect will only run once after the first render. If you pass variables in the array, the effect will run every time those variables change.
How to Use React useEffect
Using the useEffect
hook is straightforward. You call useEffect
and pass a function to it. This function contains your side effect. Let’s look at an example:
useEffect(() => {
document.title = 'Hello, world!';
});
In this example, we’re changing the document’s title. This is a side effect, and we’re using useEffect
to perform it.
Using the dependency array
The dependency array is a powerful feature of useEffect
. It allows you to control when your effect runs. Here’s an example:
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
In this example, the effect runs every time the count state changes because we’ve included count in the dependency array.
Common Use Cases for useEffect
There are many use cases for the useEffect
hook. Here are a few common ones.
Data fetching
One of the most common use cases for useEffect
is data fetching. You can use useEffect
to fetch data from an API and update your component’s state with the fetched data.
Event listeners
You can use useEffect
to add event listeners to your component. This is useful for handling user interactions, such as clicks or key presses.
Timers
useEffect
is also useful for setting up timers, such as setTimeout
or setInterval. You can use it to perform an action after a certain amount of time has passed.
Best Practices for Using useEffect
While useEffect
is a powerful tool, it’s important to use it correctly to avoid potential issues. Here are some best practices to keep in mind.
Clean up your effects
Some effects should be cleaned up before the component is unmounted to avoid memory leaks. This is especially true for effects that create subscriptions or event listeners. To clean up an effect, you can return a function from your effect that performs the cleanup.
Use multiple effects to separate concerns
If you have multiple unrelated side effects, it’s a good practice to use multiple useEffect
calls to separate concerns. This makes your code easier to understand and test.
Don’t forget the dependency array
The dependency array is a crucial part of useEffect
. Forgetting to include it can lead to unexpected behavior. Make sure to include all the variables your effect depends on in the array.
In conclusion, the React useEffect
hook is a versatile tool that allows you to handle side effects in your functional components. By understanding its usage and best practices, you can write more efficient and maintainable React code.
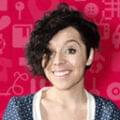
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.