Java variables enable programmers to store single data points, bits of information, for later use. For efficiency in later use, Java variables have types. Those types are referred to as data types because they allow us to store different types of data respectively for convenience and predictability. It’s necessary for any Java programmer to understand the basics of variables and data types in Java before moving on to more advanced topics. To illustrate how Java variables work, let’s imagine a photo sharing application. The app would store a whole bunch of information about the state of our application and the photos its users share: the number of users, the number of photos shared, and the total number of comments shared. That data has to be stored in order for us to manipulate and display them to our users when necessary. Enter Java variables.
Java Variables
Variables can hold data and that data can be changed over the lifetime of our program. A variable must have a type, a name, and be provided some sort of data to hold. The most widely used type of data in Java is the character string, represented by Java’sString
class. A string, such as “SitePoint” is simply an instance of the String
class.
Naming Variables
There are a few rules you must follow and a few you should. Java’s variable names are case senstive and can be an unlimited number of letters and numbers. However, variable names must start with a letter, underscore character_
, or a dollar sign $
.
When creating variables in Java, it is best to follow the convention of using numbers and full words that are descriptive of the purpose of the variable while avoiding use of the underscore character and dollar sign. Finally, variables should be in lower camel case, a popular programming convention that dictates that the first letter of the first word should be in lower case and the following words should be capitalized.
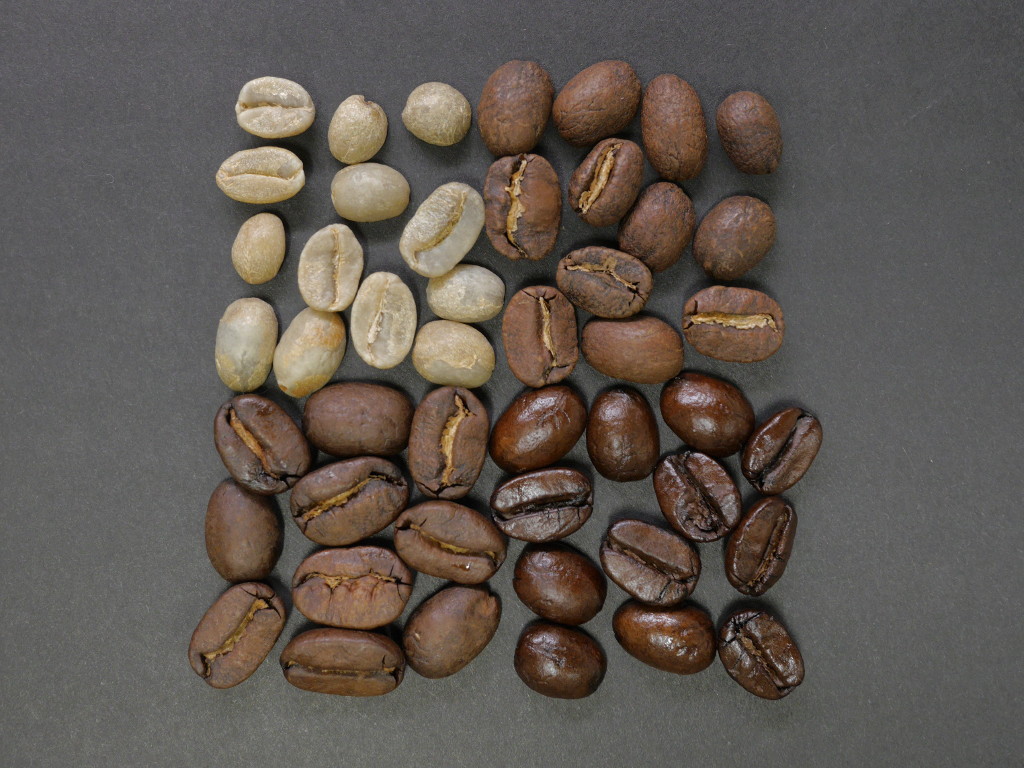
Using Variables
Let’s create the skeleton of our application’s main class to see how we can store each of the aforementioned data points about our application inString
variables:
public class SitePointGram {
public static void main(String[] args) {
String nameOfApp = "SitePointGram";
String numberOfUsers = "";
String numberOfPhotos;
String numberOfComments = null;
//...
}
}
So what’s going on there? Let’s skip to the third line of that block of Java code. On each line, we create a new variable of type String
to store a single point of information about our application. Observe that to create a variable in Java, we start by stating the type of the data to be stored in the variable, followed by the name of the variable in lower camel case, then the assignment operator =
, and finally the data to be stored in the variable.
In the first line of our main
method, we store the name of our application in the nameOfApp
String
variable and the data stored in it is “SitePointGram”. The next line has a String
variable that will store the number of users on our application. Notice that it stores an empty string ""
. Hold that thought as we move to the next two lines.
Every variable in Java has a default value; the default value for String variables is null
, “nothing”. If we don’t know the value of a variable at the time of declaration, we can omit explicitly initializing it with a value and allow Java to implicitly provide it an appropriate default value. That is exactly what we do with the numberOfPhotos
variable. Similarly, on the fourth line, we initialize the numberOfComments
String
variable to null
explicitly, although we do not have to. It’s important to understand that an empty string is an actual character string, albeit an empty one, while null
means the variable does not yet have valid data. Let’s continue.
SitePointGram is becoming popular and people are flocking to it. Let’s represent the growth of our application in Java:
public static void main(String[] args) {
//...
numberOfUsers = "500";
numberOfPhotos = "1600";
numberOfComments = "2430";
//..
}
After initializing our String
variables, it is now apparent that our application has 500 users, 1600 shared photos, and a whopping 2430 total comments across those photos. We’re doing well, so it’s time we learn how to use data types in Java.
Java Data Types
We currently have all of our data points stored inString
variables even though some of them are numbers. Strings are good for representing character strings such as text, but when we want to represent numbers and other types of data (and perform operations on that data), we can use the data types provided by Java or create our own.
Let’s see how we can more appropriately store our numerical data points in variables so we can use them as we would expect:
public static void main(String[] args) {
String appName = "SitePointGram";
boolean appIsAlive = true;
int numUsers = 500;
int numPhotos = 1600;
int numComments = 2430;
//...
}
Moving away from our original main
method, we have a new block of code with new variables of appropriate data types. In the first line of our main
method’s body, the variable holding our application’s name is now more precise: instead of nameOfApp
, we have appName
. On the next line, we have a Boolean variable which stores the status of our application. A boolean
can only be true
or false
and therefore is best when you want to store a data point that represents validity; in our case, it is true that our app is alive until we need to shut it down for maintenance. The next three variables are of type int
. The int
data type represents integer values in Java. Following the same pattern as appName
, instead of naming our number variables along the lines of numberOfX
, we should do numX
to be precise while maintaining readability.
The data types int
, boolean
, and double
are three of the eight primitive data types in Java. Primitive data types are special values, not objects constructed from a class, provided by Java. Remember that strings are instances of the String
class and so they are objects, not primitives. The default value for numerical data types is 0
and false
for the boolean
.
Unlike in our previous main
method, our new set of variables store numbers appropriately, so we are able to manipulate them as we would expect. With our numerical data points stored in variables of types that represent numbers, we can execute mathematical operations on them:
//a new user joined, increase the number of users by 1
numUsers += 1;
//multiply the number of photos by 2
numPhotos = numPhotos * 2;
//get the average number of photos by doing division
double avgPhotosPerUser = 1.0 * numPhotos / numUsers;
The last variable in our main
method holds a floating point value of the average number of photos per user and this is represented by the double
data type. We obtained this by dividing the number of photos by the number of users. Notice we multiplied the first number by 1.0 so that the result isn’t rounded to the nearest whole number. We can store a floating point number as either a float
or a double
; the only difference here is that a double
(64 bit) can hold a much larger range of numbers than a float
(32 bit) and is used more often for that reason.
The last thing to do is see how we can represent our data in our own data types.
String user1 = "id: 1, username: LincolnWDaniel, isOnline: true";
While it would be easy to make a bunch of strings that hold information about users like we have in user1
, it would be better to create a class to construct user objects from:
Custom Java Data Types (Classes)
public class User {
public static int numUsers = 0;
private int id;
private String username;
private boolean isOnline;
/*other information about this user,
perhaps a list of associated photos*/
public User(String username) {
id = numUsers++;
this.username = username;
this.isOnline = true;
System.out.println(username + " signed up!");
}
public void logOn() {
isOnline = true;
printOnlineStatus();
}
public void logOff() {
isOnline = false;
printOnlineStatus();
}
private void printOnlineStatus() {
System.out.println(username + " is online: " + isOnline);
}
//...
}
There, we have a class called User
. This class simply defines properties and behaviors instances created from it can exhibit. The properties of this class are simply variables of varying data types that will hold information about a user in our application. An instance of the User
class can have information about itself from its identification number to its username, and its online status is held in a boolean
variable that can be updated when the user logs on or off. When a user is created, or logs on or off, we print that information to the console.
Every time a new user is created in our application, the numUsers
variable’s value is increased by one so that our application knows how many users there are at all times. You could add more information to this class by adding more instance variables. Now, let’s create an instance of our new data type, User
, in our application’s main method:
public static void main(String[] args) {
String appName = "SitePointGram";
boolean appIsAlive = true;
/*declare a new variable of type User
and store a new User instance with the name of "Lincoln" in it*/
User lincoln = new User("Lincoln");
//log lincoln off
lincoln.logOff();
//print out the number of users in our app
System.out.println("Number of users: " + User.numUsers);
//...
}
In that block of code, we changed up our main
method again. The first two lines remained unchanged, but we now have three new lines. The third line in the method creates a new User
instance, or object, and stores it in a variable called “lincoln”, the next line logs lincoln
off our application, and the next line prints out the number of User
instances in our application by accessing the public
static
numUsers
variable from the User
class. It’s important to note that static
variables of a class belong to the class and not instances of that class, so we did not need an instance of User
to access numUsers
.
Conclusion
That’s it! You now know all you need to know about Java variables and data types to get started building your own data types, or classes. Take a look at the source code for this tutorial on our GitHub repository and see how you can build upon it. References: Oracle Documentation on Java Strings Oracle Documentation on Java’s Primitive Data TypesFrequently Asked Questions (FAQs) about Java Variables and Data Types
What is the difference between local and instance variables in Java?
In Java, variables are categorized into local, instance, and class variables. Local variables are declared within a method, constructor, or block and are only accessible within the scope they are declared. They don’t have a default value, so they must be initialized before use. On the other hand, instance variables are declared in a class but outside a method. They are object-specific and get memory each time an object is created. Unlike local variables, instance variables have default values based on their data types.
How does Java handle type conversion?
Java handles type conversion through two methods: implicit and explicit conversion. Implicit conversion, also known as automatic type conversion, occurs when the two types are compatible and the target type is larger than the source type. Explicit conversion, also known as casting, is when we manually convert one data type into another. This is necessary when the target type is smaller than the source type or when the types are not compatible.
What are the default values of variables in Java?
In Java, instance and class variables are automatically initialized to default values if not explicitly initialized. The default values depend on the data type. For instance, byte, short, int, and long default to 0, float and double to 0.0, char to ‘\u0000’, and boolean to false. Non-primitive data types like arrays and classes default to null.
What is the significance of ‘final’ keyword in Java variables?
The ‘final’ keyword in Java is used to declare a constant variable, meaning its value cannot be changed once assigned. It can be applied to primitive data types, objects, and methods. For instance, ‘final int x = 10;’ means that the value of x will always be 10 and cannot be modified.
How does Java handle string variables?
In Java, strings are not primitive data types, but they are special classes. Strings are immutable, meaning their values cannot be changed once created. Instead, a new string object is created. Java provides a special String pool area in the heap memory where it tries to maintain unique string values to optimize memory usage.
What is the difference between ‘==’ and ‘equals()’ in Java?
In Java, ‘==’ is a relational operator that compares the memory locations of two objects, while ‘equals()’ is a method that compares the content of two objects. For primitive data types, ‘==’ checks if the values are equal. But for objects, it checks if they refer to the exact same memory location, not their content.
What is type promotion in Java?
Type promotion in Java is the automatic conversion of one primitive data type into another to prevent data loss. It usually occurs in expressions or method invocations when the operands are of different types. The smaller type is promoted to the larger type. For instance, if an int and a float are used in an expression, the int is promoted to a float.
What is the scope of variables in Java?
The scope of a variable in Java refers to the part of the code where the variable can be accessed. Local variables can only be accessed within the method or block they are declared. Instance variables can be accessed by all methods in the class unless the method is static. Class or static variables can be accessed by all methods in the class and even outside the class if they are public.
What is the difference between static and non-static variables in Java?
In Java, static variables, also known as class variables, belong to the class and not to individual objects. They are initialized only once at the start of the execution and share the same value among all objects of the class. Non-static variables, also known as instance variables, belong to individual objects and each object has its own copy of the variable.
How are arrays handled in Java?
In Java, arrays are objects that store multiple variables of the same type. They are dynamically allocated and can store primitives or objects. The length of an array is established when the array is created and cannot be changed. Arrays have a ‘length’ property that returns the number of elements in the array. Elements in an array are accessed through their index, starting from 0.

Lincoln W Daniel is a software engineer who has worked at companies big and small. Receiving internship offers from companies like NASA JPL, he has worked at IBM and Medium.com. Lincoln also teaches programming concepts on his ModernNerd YouTube channel by which he emphasizes relating coding concepts to real human experiences.