The Hypertext Transfer Protocol (HTTP) is inarguably the most significant protocol used on the Internet today.
Web services, network enabled appliances and the growth of network computing continues to expand the role of the HTTP protocol beyond user driven web browsers, while increasing the number of applications that require HTTP support.
In recent times, companies offering products or services to end-users are ‘APIfying’ their websites and/or applications.
An API – Application Programming Interface – at its most basic level allows product or service to talk to other products or services. In this way, an API allows you to open up data and functionality to other developers, to other businesses or even between departments and locations within your company. It is increasingly the way in which companies exchange data, services and complex resources, both internally, externally with partners, and openly with the public. Credit: API Evangelist
Within PHP, there are many possible ways for sending HTTP requests. Examples include file_get_contents, fsockopen, and cURL.
Prior to WordPress 2.7, plugin developers all had their own implementation for sending HTTP requests and receiving the response which was an added burden to them because they had to support it afterwards to keep them working.
The WordPress HTTP API was created to standardize a single API that handled everything with regards to HTTP as simply as possible. The HTTP API supports various PHP HTTP transports or implementations to cater for different hosting environments and configurations.
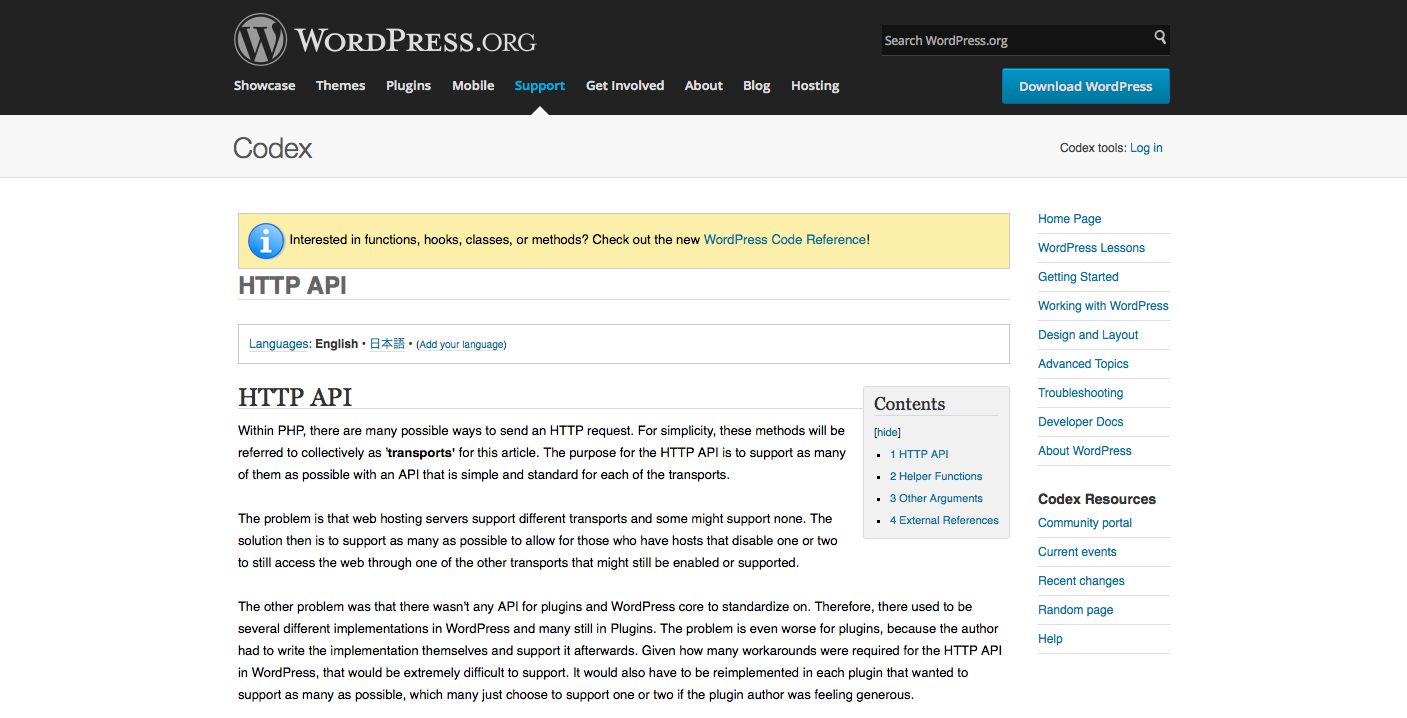
Sending Requests with the HTTP API
To send or make a request to a web service or API using the WordPress HTTP API, the following helper functions come in handy.
- wp_remote_get() – send HTTP GET method requests.
- wp_remote_post() – send HTTP POST requests.
- wp_remote_head() – send HTTP HEAD requests.
- wp_remote_request() – send requests using any custom HTTP method, be it GET, POST, HEAD, PUT or DELETE.
Soon, we’ll go over the above functions and see how they work.
For the purpose of this tutorial, all requests will be made to httpbin.org – an HTTP Request & Response Service.
Basic Examples
wp_remote_get( $url, $args )
The function above is used for sending a GET request. It has two parameters: the URL to be acted upon or endpoint ( $url
) and an array of argument details ( $args
).
Let’s see some examples to have an understanding of these functions.
$url = 'http://httpbin.org/get?a=b&c=d';
$response = wp_remote_get( $url );
All the code does is send a GET request to the endpoint http://httpbin.org/get
where the query string are the GET parameters ?a=b&c=d
Using PHP print_r function to print the HTTP data ($response
) in a human-readable format reveals the following:
Array
(
[headers] => Array
(
[access-control-allow-credentials] => true
[access-control-allow-origin] => *
[content-type] => application/json
[date] => Mon, 22 Sep 2014 15:46:40 GMT
[server] => gunicorn/18.0
[content-length] => 407
[connection] => Close
)
[body] => {
"args": {
"a": "b",
"c": "d"
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "deflate;q=1.0, compress;q=0.5, gzip;q=0.5",
"Connection": "close",
"Host": "httpbin.org",
"User-Agent": "WordPress/4.0; http://localhost/blog",
"X-Request-Id": "bb98282e-f428-48c8-a7d4-07660a70cf37"
},
"origin": "41.203.67.131",
"url": "http://httpbin.org/get?a=b&c=d"
}
[response] => Array
(
[[code]] => 200
[message] => OK
)
[cookies] => Array
(
)
[filename] =>
)
Taking a closer look at the human readable information, you will see the response is a multi-dimensional array broken into five parts: headers, body, response, cookies and filename.
- The
headers
contain the HTTP header fields of the request and response. body
is the response message sent by the API server or web service.response
contains the request HTTP status code.cookies
contains the cookies set by the web service or endpoint server if present.filename
contains the location or path of a file being sent to an API endpoint. This can be done for example by a POST request.
Let’s assume you want to inform the API you are sending a request so that the content body being sent is a JSON object, including the Content-type
header in the array of argument function parameter as follows does the trick.
$url = 'http://httpbin.org/get?a=b&c=d';
$args = array(
'headers' => array( "Content-type" => "application/json" )
);
$response = wp_remote_get( $url, $args );
To conveniently retrieve the different parts of the response and also test for any resultant error, the WordPress HTTP API provides the following helper functions:
- wp_remote_retrieve_body() – Retrieves just the body from the response.
- wp_remote_retrieve_headers() – Returns an array of all the response HTTP headers.
- wp_remote_retrieve_header() – Returns the value of an HTTP header based on the supplied name.
- wp_remote_retrieve_response_code() – Returns the response status codes of the HTTP request.
http://httpbin.org/get
, the wp_remote_retrieve_body()
(which accepts the response as an argument) comes in handy.
$url = 'http://httpbin.org/get?a=b&c=d';
$args = array(
'headers' => array( "Content-type" => "application/json" )
);
$response = wp_remote_get( $url, $args );
$body = wp_remote_retrieve_body( $response );
To retrieve only the response header, use the wp_remote_retrieve_headers()
as follows.
$response_headers = wp_remote_retrieve_headers( $response );
print_r( $response_headers );
Result:
Array
(
[access-control-allow-credentials] => true
[access-control-allow-origin] => *
[content-type] => application/json
[date] => Mon, 22 Sep 2014 19:25:57 GMT
[server] => gunicorn/18.0
[content-length] => 448
[connection] => Close
)
To return the value of a single header say content-type
, the function wp_remote_retrieve_header()
is used.
$response_header = wp_remote_retrieve_header( $response, 'content-type' );
print_r( $response_header );
Result:
application/json
To retrieve only the response status code, use the wp_remote_retrieve_response_code()
function.
$response_code = wp_remote_retrieve_response_code( $response );
print_r( $response_code );
While wp_remote_retrieve_response_code()
retrieves the response status code, wp_remote_retrieve_response_message
retrieves the status message.
Below is a header response returned by an HTTP request.
[response] => Array
(
[[code]] => 200
[message] => OK
)
The former will returns 200
(the status code) and the latter OK
(the status message).
So far, we’ve overviewed the various helper functions provided by the WordPress HTTP API, how to make GET request via wp_remote_get()
and retrieving the response headers and body using the response helper functions.
POST Requests
The functionwp_remote_post()
is use for sending POST requests.
$url = 'http://httpbin.org/post';
$args = array(
'body' => array(
"name" => "collins",
"url" => 'http://w3guy.com'
)
);
$response = wp_remote_post( $url, $args );
The code above is a basic POST request to the endpoint http://httpbin.org/post
with an array of parameter or body sent alongside.
To retrieve the response body, headers, status code and message, use the appropriate response helper function provided by the HTTP API.
Head Requests
To send an HTTP request using the HEAD method, the functionwp_remote_head()
is used.
$url = 'http://httpbin.org';
$args = array(
'body' => array(
"name" => "collins",
"url" => 'http://w3guy.com'
)
);
$response = wp_remote_head( $url, $args );
$response_code = wp_remote_retrieve_response_message( $response );
Requests Using Other HTTP Methods
To send a PUT, DELETE or any other custom HTTP method request, use thewp_remote_request()
function.
An example of a PUT request:
$url = 'http://httpbin.org';
$args = array(
'method' => 'PUT'
);
$response = wp_remote_request( $url, $args );
An example of a DELETE request:
$url = 'http://httpbin.org';
$args = array(
'method' => 'DELETE'
);
$response = wp_remote_request( $url, $args );
The function wp_remote_request()
can be use also to make GET and POST requests.
GET request
$url = 'http://httpbin.org/get';
$args = array(
'method' => 'GET'
);
$response = wp_remote_request( $url, $args );
POST request
$url = 'http://httpbin.org/post';
$args = array(
'method' => 'POST'
);
$response = wp_remote_request( $url, $args );
Advanced Configuration
- The argument
timeout
allows for setting the time in seconds before the connection is dropped and an error returned. Note: the default for this value is5 seconds
. - The timeout in the GET request below has been incremented to
45 seconds
.
$url = 'http://httpbin.org/get';
$args = array(
'method' => 'GET',
'timeout' => 45,
);
$response = wp_remote_request( $url, $args );
- The
user-agent
argument is used to set the user-agent. - The default is,
WordPress/4.0; http://w3guy.com
where 4.0 is the WordPress version number and w3guy.com, the blog URL.
$args = array(
'user-agent' => 'Crawler by w3guy',
);
- The
sslverify
argument checks to see if the SSL certificate is valid (not self-signed) and will deny the response if it isn’t. - If you know the site is self-signed but can be trusted, then set to
false
. The default value is alwaystrue
$args = array(
'sslverify' => true
);
Wrap Up
Whew! We’ve come to the end of this tutorial. By now, you should know what APIs are, have a basic understanding of the WordPress HTTP API and how it works.
In subsequent tutorials, we’ll see more of the HTTP API in action. Be sure to keep an eye on the WordPress channel.
Frequently Asked Questions (FAQs) about WordPress HTTP API
What is the WordPress HTTP API and why is it important?
The WordPress HTTP API is a set of PHP functions provided by WordPress to make HTTP requests. It is important because it allows developers to send HTTP requests to remote servers and receive responses. This functionality is crucial for integrating third-party services, retrieving data from other websites, and performing other tasks that require communication with external servers.
How does the WordPress HTTP API work?
The WordPress HTTP API works by providing a set of functions that developers can use to send HTTP requests. These functions handle the complexities of HTTP communication, such as managing headers, cookies, and redirects, allowing developers to focus on the specifics of their application.
What are some common uses of the WordPress HTTP API?
The WordPress HTTP API is commonly used for tasks such as retrieving data from external APIs, sending data to remote servers, integrating third-party services, and more. For example, a developer might use the HTTP API to retrieve the latest posts from a blog, send user data to a CRM system, or integrate a payment gateway.
How can I use the WordPress HTTP API in my own projects?
To use the WordPress HTTP API in your own projects, you can use the provided functions such as wp_remote_get(), wp_remote_post(), and wp_remote_request(). These functions allow you to send GET, POST, and general requests, respectively. You can pass in the URL of the remote server and any necessary parameters, and the function will return the server’s response.
What are the differences between wp_remote_get(), wp_remote_post(), and wp_remote_request()?
The main difference between these functions is the type of HTTP request they send. wp_remote_get() sends a GET request, which is typically used to retrieve data. wp_remote_post() sends a POST request, which is typically used to send data. wp_remote_request() can send any type of request, and is used when the other two functions are not suitable.
How can I handle errors with the WordPress HTTP API?
The WordPress HTTP API functions return a WP_Error object if an error occurs. You can check for errors using the is_wp_error() function. If an error occurs, you can use the get_error_message() method of the WP_Error object to retrieve the error message.
Can I use the WordPress HTTP API to send requests to any server?
Yes, you can use the WordPress HTTP API to send requests to any server that accepts HTTP requests. However, keep in mind that the server’s response may be affected by factors such as its configuration, the presence of a firewall, and the specifics of the HTTP request.
What is the performance impact of using the WordPress HTTP API?
The performance impact of using the WordPress HTTP API depends on several factors, including the complexity of the request, the speed of the remote server, and the network latency. However, WordPress has built-in mechanisms to handle HTTP communication efficiently, so the impact is typically minimal.
Can I use the WordPress HTTP API with non-WordPress projects?
The WordPress HTTP API is a part of WordPress, so it can’t be used directly in non-WordPress projects. However, the principles of HTTP communication are the same, so you can use similar functions provided by other frameworks or write your own HTTP communication code.
How can I learn more about the WordPress HTTP API?
The official WordPress developer documentation is a great resource for learning more about the WordPress HTTP API. It provides detailed information about the API’s functions, how to use them, and examples of their use. Additionally, there are many tutorials and articles available online that provide practical examples and explanations.
Collins is a web developer and freelance writer. Creator of the popular ProfilePress and MailOptin WordPress plugins. When not wrangling with code, you can find him writing at his personal blog or on Twitter.