Opera Software recently released version 12 of its flagship web browser. With its latest release, Opera became the first of the major browsers to begin supporting the W3C’s Multimedia Stream API. The Stream API, also referred to as the getUserMedia API, allows the user’s camera and microphone inputs to be streamed to a browser window. Once passed to the browser, the stream is typically used as the “src” attribute of a <video> element. Because the Stream API is still a widely unsupported draft, it is likely to change over time. This post covers the basics of the Stream API. As the draft becomes more stable and widely supported, this post will be expanded upon.
Detecting Support
Currently, Opera is the only browser to support the Stream API. Therefore, it is absolutely necessary to detect whether or not the API is supported before trying to use it. The following function detects stream support by checking for the existence of the navigator object’s getUserMedia() method.
function isStreamSupported() { if (navigator.getUserMedia) return true; else return false; }
The getUserMedia() Method
The Stream API is accessed via the navigator.getUserMedia() method. However, before any multimedia streams can be accessed, the user must grant explicit permission to the browser. When getUserMedia() is called, Opera uses the following dialog box to receive the user’s consent.
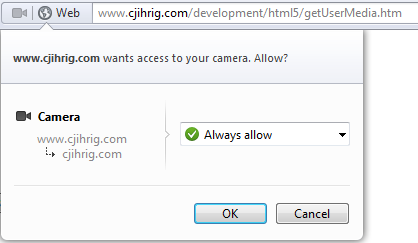
The syntax for getUserMedia() is shown below. The method takes two mandatory arguments, and an optional third argument. The first argument, “constraints”, is an object that specifies which media streams (ie video and/or audio) are requested by the browser. The second argument, “successCallback”, is a callback function which is executed if getUserMedia() is successful. The resulting media stream object is passed to “successCallback” as its only argument. The optional third argument, “errorCallback”, is a callback function that executes if getUserMedia() fails. For example, a failure occurs if the user does not agree to let the browser access the multimedia streams.
navigator.getUserMedia( constraints, successCallback[, errorCallback] )
Streaming to a <video> Element
The following example shows how getUserMedia() can be used to send a camera stream directly to an HTML <video> element. The example provides play, pause, and stop buttons for controlling the multimedia streams. Note that the “constraints” variable causes the browser to request both audio and video streams. If you are using Opera, the example is also live online here.
<!DOCTYPE html> <html lang="en"> <head> <title>getUserMedia Example</title> <meta charset="UTF-8"/> <script> window.addEventListener("load", function() { var camera = document.getElementById("camera"); var play = document.getElementById("play"); var pause = document.getElementById("pause"); var stop = document.getElementById("stop"); var constraints = {audio:true, video:true}; function success(stream) { camera.src = stream; camera.play(); disableButtons(true, false, false); } function failure(error) { alert(JSON.stringify(error)); } function disableButtons(disPlay, disPause, disStop) { play.disabled = disPlay; pause.disabled = disPause; stop.disabled = disStop; } disableButtons(true, true, true); if (navigator.getUserMedia) navigator.getUserMedia(constraints, success, failure); else alert("Your browser does not support getUserMedia()"); play.addEventListener("click", function() { disableButtons(true, false, false); camera.play(); }, false); pause.addEventListener("click", function() { disableButtons(false, true, false); camera.pause(); }, false); stop.addEventListener("click", function() { disableButtons(true, true, true); camera.pause(); camera.src = ""; }, false); }, false); </script> </head> <body> <button id="play">Play</button> <button id="pause">Pause</button> <button id="stop">Stop</button> <br /> <video id="camera"></video> </body> </html>
Frequently Asked Questions (FAQs) about Streaming Your Webcam to a Browser in JavaScript
How can I ensure my webcam stream is secure when using JavaScript?
Security is a crucial aspect when dealing with webcam streams. To ensure your stream is secure, always use the HTTPS protocol instead of HTTP. This is because getUserMedia(), the API used for accessing media devices like webcams, only works on secure origins. Additionally, always request user permission before accessing their webcam to maintain privacy and trust.
Why is my webcam not working with getUserMedia() API?
There could be several reasons why your webcam is not working with getUserMedia() API. First, ensure that your browser supports this API. Most modern browsers do, but it’s always good to check. Secondly, make sure you’re using a secure context (HTTPS). getUserMedia() only works on secure origins. Lastly, check if the webcam is already in use by another application, as this can prevent access.
Can I use JavaScript to stream my webcam to multiple browsers simultaneously?
Yes, it is possible to stream your webcam to multiple browsers simultaneously using JavaScript. This can be achieved by setting up a WebRTC (Web Real-Time Communication) connection, which allows for real-time communication between browsers. However, this requires a more complex setup and may involve using a signaling server to coordinate communication between different browsers.
How can I adjust the resolution of my webcam stream in JavaScript?
You can adjust the resolution of your webcam stream by passing constraints to the getUserMedia() method. These constraints can specify the exact height and width you want for your video. However, keep in mind that not all webcams will support all resolutions, and the actual resolution may be lower than what you requested.
Why is there a delay in my webcam stream?
Delays in webcam streams can be due to several factors including network latency, processing power of your device, and the performance of the browser. To reduce delay, you can try lowering the resolution of the stream, using a faster internet connection, or upgrading your device or browser.
Can I record my webcam stream using JavaScript?
Yes, you can record your webcam stream using JavaScript. This can be done using the MediaRecorder API, which allows you to record media streams. Once the stream is recorded, you can then save it as a video file.
How can I handle errors when streaming my webcam with JavaScript?
When using the getUserMedia() API, you can handle errors by using a catch block to catch any Promise rejection. The error object that is returned will give you more information about what went wrong, allowing you to handle the error appropriately.
Can I stream audio along with video from my webcam using JavaScript?
Yes, you can stream both audio and video from your webcam using JavaScript. When calling the getUserMedia() method, you can specify that you want to access both audio and video by passing in the appropriate constraints.
How can I improve the performance of my webcam stream in JavaScript?
To improve the performance of your webcam stream, you can try several things. First, consider lowering the resolution of the stream. High-resolution streams require more processing power and bandwidth. Second, ensure that your code is efficient and doesn’t have any unnecessary operations. Lastly, consider using a faster internet connection if possible.
Can I apply filters or effects to my webcam stream using JavaScript?
Yes, you can apply filters or effects to your webcam stream using JavaScript. This can be done using the Canvas API, which allows you to manipulate and apply effects to images and video. However, keep in mind that applying filters or effects can increase the processing power required and may affect the performance of the stream.
Colin Ihrig is a software engineer working primarily with Node.js. Colin is the author of Pro Node.js for Developers, and co-author of Full Stack JavaScript Development with MEAN. Colin is a member of the Node.js Technical Steering Committee, and a hapi core team member. Colin received his Bachelor of Science in Engineering, and Master of Science in Computer Engineering from the University of Pittsburgh in 2005 and 2008, respectively.