Spammers are everywhere, they use automated software that crawls the web in search of websites (such WordPress sites) with the aim of submitting and registering hundreds and thousands of accounts and spam comments.
On one of my WordPress powered sites, I discovered over 50 newly registered spam accounts, all created with disposable email addresses. To prevent a re-occurrence, I had to create a plugin that prevented the registration of accounts with disposable email addresses.
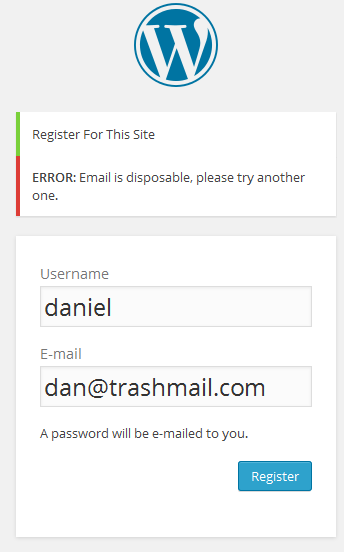
In this tutorial, we’ll learn the meaning of disposable email addresses, how they work and finally, how they can be stopped in a PHP application – albeit with focus on WordPress.
Introduction to Disposable Email Addresses
DEA, an acronym for Disposable Email Address (sometimes referred to as throw-away, temporary email or self-destructive email), is a service that allows a registered user to receive email at a temporary address that expires after a certain time period lapses. Simply put, they are email accounts created to accomplish a short-term goal.
Examples of disposable email providers include: mailinator.com, YOPmail.com, trashmail.com, and many more.
The Good
The original intent behind disposable email addresses is to protect oneself from untrusted websites, typically to avoid spam.
The Bad and the Ugly
Wikipedia has a great explanation why disposable emails are – should I say bad or ugly?
Many forum and wiki administrators dislike DEAs because they obfuscate the identity of the members and make maintaining member control difficult. As an example, trolls, vandals and other users that may have been banned may use throwaway email addresses to get around the ban. Using a DEA provider only makes this easier; the same convenience with which a person may create a DEA to filter spam also applies to trolls. As a result, forum, wiki administrators, blog owners, and indeed any public site requiring user names may have a compelling reason to ban DEAs.
Because spammers can use disposable emails to perpetrate their evil activities, we really need to give serious thought to how we can stop DEAs.
Detecting Disposable Email Addresses
There is no algorithm (to the best of my knowledge) for detecting if an email is disposable or not.
To detect a disposable email address: – Firstly, you will have to create and maintain a list/database of disposable email domains. – Check if the domain part of the email (e.g. in “hi@trashmail.com”, “trashmail.com” is the domain part) is in the database.
Below is a PHP function that accepts an email address as an argument and return true if it is disposable or false otherwise.
<?php
/**
* Check if an email is disposable or not.
*
* @param $email string email to check
*
* @return bool
*/
function detect_disposable_email( $email ) {
$disposable_list = array(
'drdrb.net',
'upliftnow.com',
'uplipht.com',
'venompen.com',
'veryrealemail.com',
'viditag.com',
'viewcastmedia.com',
'viewcastmedia.net',
'viewcastmedia.org',
'gustr.com',
'webm4il.in',
'wegwerfadresse.de',
'wegwerfemail.de',
'wetrainbayarea.com',
'wetrainbayarea.org',
'wh4f.org',
'whyspam.me',
'willselfdestruct.com',
'winemaven.in',
'wronghead.com',
'wuzup.net',
'wuzupmail.net',
'www.e4ward.com',
'www.gishpuppy.com',
'www.mailinator.com',
'wwwnew.eu',
'xagloo.com',
'xemaps.com',
'xents.com',
'xmaily.com',
'xoxy.net',
'yep.it',
'yogamaven.com',
'yopmail.fr',
'yopmail.net',
'ypmail.webarnak.fr.eu.org',
'yuurok.com',
'zehnminutenmail.de',
'zippymail.in',
'zoaxe.com',
'zoemail.org',
'inboxalias.com',
'koszmail.pl',
'tagyourself.com',
'whatpaas.com',
'emeil.in',
'azmeil.tk',
'mailfa.tk',
'inbax.tk',
'emeil.ir',
'crazymailing.com',
'mailimate.com'
);
//extract domain name from email
$domain = array_pop( explode( '@', $email ) );
if ( in_array( $domain, $disposable_list ) ) {
return true;
}
else {
return false;
}
}
//extract domain name from email
$domain = array_pop( explode( '@', $email ) );
if ( in_array( $domain, $disposable_list ) ) {
return true;
}
else {
return false;
}
}
The numbers of disposable email providers are increasing by the day, thus making it impossible to easily keep our list of DEAs updated.
There exist a number of services that keep an updated list of disposable emails and also exposes an API for detecting them, such as NameAPI and block-disposable-email.com. We’ll be using the latter in coding a plugin that will block users trying to create an account with a disposable email in WordPress.
Stopping Disposable Email Registration in WordPress
As previously mentioned, we will use block-disposable-email.com. Before we delve into the plugin development, register an account at the site with a non-disposable email (of course) to grab a free API key.
Note: the free account comes with a limitation of up to 200 requests per month. To increase the quota, see the pricing page.
With that said, let’s begin the plugin development.
First off, include the plugin header.
<?php
/*
Plugin Name: Stop Disposable Email Sign-ups
Plugin URI: https://www.sitepoint.com
Description: Stop users from registering a WordPress account with disposable emails.
Version: 1.0
Author: Agbonghama Collins
Author URI: http://w3guy.com
License: GPL2
*/
Create a PHP class with a properties that will store the API key.
class Stop_Disposable_Email {
/** @type string API key */
static private $api_key = 'd619f9ad24052ad785d1edf65bbd33b4';
The class constructor method will consist of a filter that hooks a method (stop_disposable_email_signup
) to registration_errors to validate the email address and ensure it isn’t disposable.
public function __construct() {
add_filter( 'registration_errors', array( $this, 'stop_disposable_email_signups' ), 10, 3 );
}
Next we use a helper is_email_disposable()
method that will send a GET request to the block-disposable-email.com API via wp_remote_get
using the WordPress HTTP API to check the status of the email – that is, if it is disposable or not.
/**
* Check if an email is disposable or not.
*
* @param $email string email to check
*
* @return bool true if disposable or false otherwise.
*/
public static function is_email_disposable( $email ) {
// get the domain part of the email address
// e.g in hi@trashmail.com, "trashmail.com" is the domain part
$domain = array_pop( explode( '@', $email ) );
$endpoint = 'http://check.block-disposable-email.com/easyapi/json/' . self::$api_key . '/' . $domain;
$request = wp_remote_get( $endpoint );
$reponse_body = $body = wp_remote_retrieve_body( $request );
$response_in_object = json_decode( $reponse_body );
$domain_status = $response_in_object->domain_status;
if ( $response_in_object->request_status == 'success' ) {
if ( $domain_status == 'block' ) {
return true;
} elseif ( $domain_status == 'ok' ) {
return false;
}
}
}
Here is the code for stop_disposable_email_signups()
that will stop users of disposable email addresses from creating an account.
/**
* Stop disposable email from creating an account
*
* @param $errors WP_Error Registration generated error object
* @param $sanitized_user_login string sign-up username
* @param $user_email string sign-up email
*
* @return mixed
*/
public function stop_disposable_email_signups( $errors, $sanitized_user_login, $user_email ) {
if ( self::is_email_disposable( $user_email ) ) {
$errors->add( 'disposable_email', '<strong>ERROR</strong>: Email is disposable, please try another one.' );
}
return $errors;
}
Finally, we close the plugin class.
} // Stop_Disposable_Email
Suggestions for Plugin Improvement
I created a class property and manually added my block-disposable-email.com API key to it. Ideally, a settings page for the plugin should have been created with a form field that will save the key to the database for reuse by the plugin.
Let’s make this an assignment for you. This is one way on how you might do this.
- Create a settings page for the plugin with an input field that will save the key to the database, here is a great guide.
- Retrieve the API key from the database with get_option function and use that instead.
Summary
In this article, we learned the meaning of DEAs, modus-operandi and the good, the bad and the ugly of disposable email address systems. We learned how DEAs can be stopped, and finally created a plugin for stopping users from registering an account with a disposable email address in a WordPress powered site.
The plugin is available on GitHub in case you want to use it on your site or further extend it.
If you have any questions or contributions, please let us know in the comments.
Frequently Asked Questions (FAQs) about Disposable Email Addresses in WordPress
What are disposable email addresses and why are they used?
Disposable email addresses are temporary, throw-away addresses that are used for a short period of time. They are often used to sign up for online services where the user doesn’t want to provide their real email, to avoid spam or maintain privacy. However, they can also be used for malicious purposes, such as spamming, trolling, or other forms of online abuse.
Why should I stop the use of disposable email addresses in WordPress?
Allowing disposable email addresses can lead to a number of issues for your WordPress site. It can lead to an increase in spam comments, fake user registrations, and potential security threats. By blocking disposable email addresses, you can maintain the integrity of your user base and protect your site from potential harm.
How can I block disposable email addresses in WordPress?
There are several plugins available that can help you block disposable email addresses in WordPress. These plugins work by checking the domain of the email address against a list of known disposable email providers. If a match is found, the registration or comment is blocked.
Are there any downsides to blocking disposable email addresses?
While blocking disposable email addresses can help protect your site, it can also potentially turn away legitimate users who prefer to use disposable email addresses for privacy reasons. It’s important to weigh the pros and cons and consider your specific needs and audience.
Can I manually block specific email domains in WordPress?
Yes, WordPress allows you to manually block specific email domains. This can be done through the settings in your WordPress dashboard. However, this method can be time-consuming and may not be as effective as using a plugin.
What are some popular disposable email address providers?
Some popular disposable email address providers include Temp-Mail, DisposableMail, Tempail, and Internxt. These services provide temporary email addresses that can be used to sign up for online services without revealing your real email address.
How can I identify a disposable email address?
Disposable email addresses often come from specific domains associated with disposable email services. They may also look unusual or random, as they are often generated automatically.
Can disposable email addresses be traced?
Disposable email addresses are designed to be anonymous and untraceable. However, like any online activity, they can potentially be traced by law enforcement or other authorities with the right resources and legal justification.
Are disposable email addresses illegal?
No, using a disposable email address is not illegal. However, they can be used for illegal activities, such as spamming, fraud, or harassment. It’s also against the terms of service of many websites to use disposable email addresses.
What are some alternatives to using disposable email addresses?
If you’re concerned about privacy, there are other ways to protect your email address without using a disposable one. You can use a secondary email address for sign-ups, use an email alias, or use a service that provides a permanent but private email address.
Collins is a web developer and freelance writer. Creator of the popular ProfilePress and MailOptin WordPress plugins. When not wrangling with code, you can find him writing at his personal blog or on Twitter.