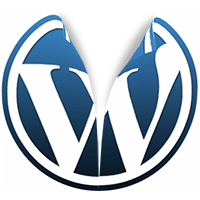
the_content(args);
But what if you need to split the content into two or more blocks? That might be necessary if your theme requires multiple columns or sections on the page. WordPress provides a get_the_content()
function to return content as a PHP variable, but how do you determine where the divisions occur? There are a few solutions on the web, but most involve either:
- Splitting the content at HTML tags such as
h2
headings. Unfortunately, that requires the content author to know a little HTML and it’s not very versatile — you couldn’t allow two headings in one column. - Using a WordPress shortcode. That’s more flexible, but it still puts the onus on the content editor to remember and use the right code.
<!--more-->
tag may offer a better solution. It’s normally used to split a long article into two or more pages, but not all themes use that facility and it only works for WordPress posts by default (not pages). Using the <!--more-->
tag offers several advantages:
- A “more” toolbar button is available in both the visual and HTML editing pane.
- Divisions can be placed anywhere in the content.
- It’s easy for non-technical users to understand how the content will be split.
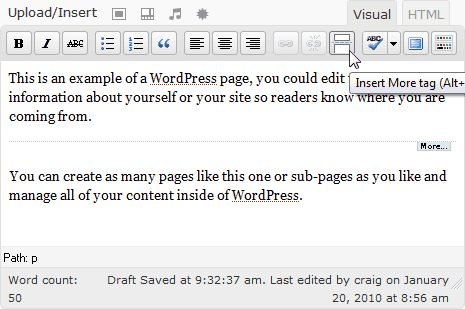
// split content at the more tag and return an array
function split_content() {
global $more;
$more = true;
$content = preg_split('/<span id="more-d+"></span>/i', get_the_content('more'));
for($c = 0, $csize = count($content); $c < $csize; $c++) {
$content[$c] = apply_filters('the_content', $content[$c]);
}
return $content;
}
You now need to locate the theme files which call the_content()
within the WordPress loop. You should find it in single.php and page.php since these are used to display single posts and pages respectively. It may also be found in index.php, archive.php and search.php, however, these normally show more than one article so be careful how multiple content blocks are handled.
Once you’ve found the relevant code, comment out the_content()
and call the split_content()
function. It returns the content as an array; each element contains a single content block split at the <!--more-->
tag, e.g. $content[0], $content[1], $content[2] … etc. The HTML can then be output as required, e.g.
< ?php
// original content display
// the_content('<p>Read the rest of this page »</p>');
// split content into array
$content = split_content();
// output first content section in column1
echo '<div id="column1">', array_shift($content), '</div>';
// output remaining content sections in column2
echo '<div id="column2">', implode($content), '</div>';
?>
I hope you find it useful.
Frequently Asked Questions (FAQs) about Splitting WordPress Content into Multiple Sections
How can I split my WordPress content into multiple sections without using any plugins?
You can split your WordPress content into multiple sections without using any plugins by using the built-in WordPress editor. Simply click on the “+” button to add a new block and select the “Columns” block. You can then add as many columns as you need and fill them with your content. This method is straightforward and doesn’t require any additional software.
Can I use a plugin to split my WordPress content into multiple sections?
Yes, there are several plugins available that can help you split your WordPress content into multiple sections. One popular option is the Advanced Gutenberg plugin, which offers a multi-column block that you can customize to fit your needs.
How can I convert a container to a column in WordPress?
To convert a container to a column in WordPress, you need to first select the container block. Then, click on the “Change block type” button (which looks like a rectangle with arrows) in the toolbar at the top of the editor. From the dropdown menu, select “Columns.” Your container block will then be converted into a column block.
Can I split my WordPress content into more than two columns?
Yes, you can split your WordPress content into more than two columns. When you add a new “Columns” block, you can choose the number of columns you want to add. You can add up to six columns in a single block.
How can I add multi-column content in WordPress posts without using HTML?
You can add multi-column content in WordPress posts without using HTML by using the built-in WordPress editor. Simply add a new “Columns” block and fill it with your content. You can customize the number of columns and their layout to fit your needs.
Is there a way to split WordPress content into multiple sections on the mobile version of my site?
Yes, the “Columns” block in WordPress is responsive, which means it will automatically adjust to fit the screen size of the device your site is being viewed on. This includes mobile devices.
Can I customize the layout of my columns in WordPress?
Yes, you can customize the layout of your columns in WordPress. After adding a “Columns” block, you can adjust the width of each column by dragging the divider between them. You can also choose to have your columns of equal width or varying widths.
How can I add images to my columns in WordPress?
To add images to your columns in WordPress, first add a “Columns” block. Then, in each column, add an “Image” block. You can then upload or select an image to add to each column.
Can I add different types of content to each column in WordPress?
Yes, you can add different types of content to each column in WordPress. Each column acts as a separate block, so you can add any type of block to each column. This includes text, images, videos, and more.
How can I change the order of my columns in WordPress?
To change the order of your columns in WordPress, you need to first select the “Columns” block. Then, in the block settings panel on the right, you can use the “Move Up” and “Move Down” buttons to change the order of your columns.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.