Quick point. It might be beneficial to mention that we are using vanilla for loops instead of jQuery.each for speed (up to 84% faster). Using a for loop with variable caching produces even faster results jsperf – each vs for.
jQuery.each
$.each(a, function() {
e = this;
});
For Loop with Caching
for ( var i = 0, len = a.length; i < len; i++) {
e = a[ i] ;
};
For Loop without Caching
for (var i = 0; i < a.length; i++) {
e = a[i];
};
for (var i = 0; i < a.length; i++) {
e = a[i];
};
Pre-calculated Length attempt
var len = a.length, i = 0;
for (i; i < len; i++) {
e = a[i];
};
var len = a.length, i = 0;
for (i; i < len; i++) {
e = a[i];
};
Frequently Asked Questions (FAQs) about jQuery Each vs Loop
What is the main difference between jQuery each and a loop?
The main difference between jQuery each and a loop lies in their functionality and usage. jQuery each is a method that is used to iterate over an array or an object. It is a high-level, abstracted way of looping through elements, providing a simpler syntax and avoiding the need to manually increment counters. On the other hand, a loop, such as a for loop, is a fundamental programming construct that allows for repeated execution of a block of code. It provides more control over the iteration process, but requires manual management of the loop counter and condition.
Is jQuery each slower than a for loop?
Yes, jQuery each is generally slower than a for loop. This is because jQuery each involves function calls and overheads that a simple for loop does not have. However, the difference in speed is usually negligible in everyday coding and is only noticeable when dealing with a large number of iterations.
When should I use jQuery each instead of a for loop?
jQuery each is best used when you are working with arrays or objects and you want a simpler, more readable syntax. It is also useful when you want to avoid manually managing loop counters and conditions. However, if performance is a critical factor and you are dealing with a large number of iterations, a for loop would be a better choice.
Can I break out of a jQuery each loop?
Yes, you can break out of a jQuery each loop by returning false in the callback function. This is equivalent to the ‘break’ statement in a for loop. However, note that returning true in the callback function will not continue to the next iteration as it would in a for loop, but will instead just move on to the next item in the array or object.
How can I access the current index or element in a jQuery each loop?
In a jQuery each loop, the current index and element can be accessed through the parameters of the callback function. The first parameter represents the index and the second parameter represents the current element. For example, in the loop $.each(array, function(index, element) {...})
, ‘index’ is the current index and ‘element’ is the current element.
Can I use jQuery each with a NodeList or HTMLCollection?
Yes, jQuery each can be used with a NodeList or HTMLCollection. However, note that the ‘this’ keyword within the callback function will refer to a native DOM element, not a jQuery object. To use jQuery methods on the element, you will need to wrap it in the jQuery function, like so: $(this)
.
Is there a performance difference between jQuery each and JavaScript’s native forEach method?
Yes, there is a performance difference between jQuery each and JavaScript’s native forEach method. Generally, native JavaScript methods are faster than jQuery methods because they do not have the overhead of jQuery’s abstraction. However, the difference in speed is usually negligible in everyday coding.
Can I use jQuery each with an object?
Yes, jQuery each can be used with an object. The callback function will be called for each property on the object, with the property name and value as its parameters.
How does jQuery each handle sparse arrays?
jQuery each handles sparse arrays by skipping over the undefined indices. This is different from a for loop, which will include the undefined indices in the iteration.
Can I use jQuery each in a chain of jQuery methods?
Yes, jQuery each can be used in a chain of jQuery methods. However, note that it does not return the original jQuery object, but instead returns the result of the iteration. This means that you cannot continue the chain after using each.
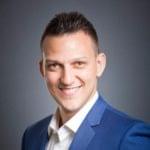
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.