Chrome apps are popular among Chrome users. And why not, they provide a method of creating a portable ‘application’ within the familiar environment of the Chrome browser.
Imagine the power of Chrome apps available on your smartphone. We can now run our favorite chrome apps on Android and iOS using a tool set based on Apache Cordova.
Cordova is an open source framework for packaging mobile apps using HTML, CSS and JavaScript. Cordova wraps the HTML, CSS and JavaScript web app using the native shell and allows for distribution on Google Play, The Apple App Store and other stores.
Overview
In this tutorial, we’ll create our own Chrome app using HTML, CSS and JavaScript and port it to the Android emulator. The application we’ll be creating will be a simple app to fetch the latest tweets based on a particular twitter hashtag.
Creating Chrome App
We’ll start by creating our Chrome app. Once we have create the Chrome app and tested it on the Chrome browser, we’ll port it to Android.
Source code from this tutorial is available on GitHub.
Create a project folder called TwitterChromeApp
. Inside the project folder create a manifest file called manifest.json
which will be the config file for our app. Inside manifest.json
we’ll define a few settings required by the Chrome app. Chrome apps require manifest_version
to be 2
. We’ll also define the name of the app, its version and the path to a background script to execute on launching the app. We’ll be fetching the tweets from an external service url, so we’ll also specify that inside this file. Here is how the manifest.json
should look:
{
"manifest_version": 2,
"name": "Tweet Chrome App",
"version": "1.0",
"app": {
"background": {
"scripts": ["scripts/main.js"]
}
},
"permissions": [
"http://twittersearchapi.herokuapp.com/search"
]
}
When the Chrome app launches we will show a window where the tweets from twitter will be displayed. Chrome apps have an event called onLaunched
which we use to create the window for our app on app launch.
Inside the project folder TwitterChromeApp
, create another folder called scripts
and inside it create the background script called main.js
. Add the following code to main.js
:
chrome.app.runtime.onLaunched.addListener(function() {
// creating window for app code will be here
});
Inside the onLaunched
event we will create a widow for our Chrome app. Add the following code to main.js
:
chrome.app.runtime.onLaunched.addListener(function() {
var screenWidth = screen.availWidth;
var screenHeight = screen.availHeight;
var width = 500;
var height = 300;
chrome.app.window.create('index.html', {
id: "tweetAppID",
outerBounds: {
width: width,
height: height,
left: Math.round((screenWidth - width) / 2),
top: Math.round((screenHeight - height) / 2)
}
});
});
In the above code, we have used the screen
object to get the available screen width and height. Based on the actual width of the screen, we set the outer bounds of the new Chrome window to display correctly.
chrome.app.window.create
creates a new window using the html inside the file index.html
.
Inside the project folder TwitterChromeApp
create a new file called index.html
as shown below:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Chrome Tweet App</title>
</head>
<body>
<h1>Tweets !!</h1>
</body>
</html>
Now try to install the Chrome app in your Chrome browser. Open to Tools -> Extensions (possibly More Tools). Enable developer mode from the checkbox in the top right and then click on Load unpacked extension and select the project folder.
Run the extension either through the Apps or Extensions window and you should see the below:
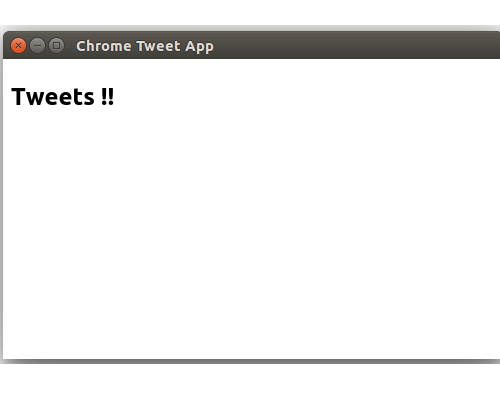
Next, we’ll make create an ajax call that is triggered when the window launches that retrieves tweets from a service url. We’ll be using a service hosted on Heroku. The service has a few limitations. It only fetches tweets with the hashtag perkytweets, this is enough for our example.
We’ll use jQuery to make our AJAX call, so download it to the scripts
folder and include it in index.html
, for example:
<script type="text/javascript" src="scripts/jquery-1.11.1.min.js"></script>
Now create a new file called script.js
in the scripts
folder and create the ajax call as shown:
$(function() {
$.ajax({
type: 'GET',
url: 'http://twittersearchapi.herokuapp.com/search',
success: function(response) {
var resObj = JSON.parse(response);
console.log(resObj);
},
error: function(error) {
console.log(error);
}
});
});
Include script.js
in index.html
:
<script type="text/javascript" src="scripts/script.js"></script>
Now open Tools -> extensions (Or More tools) and click reload to reflect the changes. Next click on the app to relaunch it. If you check the Chrome console (just the normal console you access in Chrome), it should show the response from the service URL.
Next, we’ll display the responses in index.html
. We’ll be using Bootstrap for styling the page.
Create a styles
folder inside your project folder and download the Bootstrap css files into the folder. Include the Bootstrap CSS file in index.html
.
<link href="styles/bootstrap.min.css" rel="stylesheet">
Include a ul
element in index.html
to display the tweets. Here is how index.html
should look now:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Chrome Tweet App</title>
<link href="styles/bootstrap.min.css" rel="stylesheet">
<script type="text/javascript" src="scripts/jquery-1.11.1.min.js"></script> <!-- Check this matches your jQuery version and file name -->
<script src="scripts/script.js"></script>
</head>
<body>
<h1>Tweets !!</h1>
<ul id="ulTweets" class="list-group">
</ul>
</body>
</html>
Inside the AJAX success call back in scripts.js
include the following code to append the items fetched from the service call into the ul
in index.html
.
$.each(resObj, function(index, value) {
$('#ulTweets').append('<li class="list-group-item"><span class="badge">' + resObj[index].retweet_count + '</span>' + resObj[index].text + '</li>');
});
Reload and relaunch the app and you should be able to see a screen full of tweets.
Creating a Chrome App for Android
Since we’ll be running our app on Android, make sure you have Java JDK 7 or higher, Android SDK 4.4.2 or higher and Apache Ant installed on your system.
We’ll also need the cca
command line tool. You can install it using
npm install -g cca
Detailed information on setting up your environment for porting Chrome apps to Android and iOS can be found in the official docs.
Once setting up our environment is complete, we’ll create a new project from our existing TwitterChromeApp
to port to Android.
Run the following command to create the project:
cca create TwitterAppForAndroid --copy-from=path/to/TwitterChromeApp/manifest.json
Navigate to TwitterAppForAndroid
and run the following command to run the project in the android emulator:
cd TwitterAppForAndroid
cca emulate android
Once the emulator successfully launches, you should see the app running inside the emulator.
Conclusion
In this tutorial, we saw how to create a simple chrome app and port it to the Android platform. Further information on running Chrome Apps on mobile devices using Apache Cordova can be found in the officials docs.
What do you think about this Chrome App option for creating a mobile app? Does it offer any advantages over just using a standard HTML, CSS and JavaScript web app inside Cordova?
Frequently Asked Questions (FAQs) about Running Chrome Apps on Mobile Device Using Cordova
How can I install Cordova on my system?
To install Cordova, you need to have Node.js installed on your system. Once Node.js is installed, you can install Cordova using npm (Node Package Manager) by running the command npm install -g cordova
in your terminal or command prompt. The -g
flag is used to install Cordova globally on your system.
What are the prerequisites for running Chrome apps on a mobile device using Cordova?
Before you can run Chrome apps on a mobile device using Cordova, you need to have the following installed on your system: Node.js, Cordova, Chrome Apps for Mobile toolchain, and Android SDK or iOS SDK depending on the platform you are targeting.
How can I convert my Chrome app into a Cordova project?
To convert your Chrome app into a Cordova project, you need to use the cca
command followed by the create
command and the name of your project. For example, cca create MyProject
. This will create a new Cordova project in a directory named MyProject.
How can I add platforms to my Cordova project?
To add platforms to your Cordova project, you need to use the cordova platform add
command followed by the name of the platform. For example, cordova platform add android
will add the Android platform to your project.
How can I build my Cordova project?
To build your Cordova project, you need to use the cordova build
command followed by the name of the platform. For example, cordova build android
will build your project for the Android platform.
How can I run my Cordova project on a device?
To run your Cordova project on a device, you need to use the cordova run
command followed by the name of the platform. For example, cordova run android
will run your project on an Android device.
How can I debug my Cordova project?
To debug your Cordova project, you can use Chrome DevTools. To do this, you need to navigate to chrome://inspect
in your Chrome browser and click on the inspect link under your device.
What are the limitations of running Chrome apps on a mobile device using Cordova?
While Cordova allows you to run Chrome apps on a mobile device, there are some limitations. For example, not all Chrome APIs are supported, and there may be differences in behavior between the Chrome app and the Cordova app due to differences in the underlying platforms.
Can I use Cordova plugins in my Chrome app?
Yes, you can use Cordova plugins in your Chrome app. To do this, you need to add the plugin to your project using the cordova plugin add
command followed by the name of the plugin.
How can I update my Cordova project?
To update your Cordova project, you can use the cordova platform update
command followed by the name of the platform. For example, cordova platform update android
will update the Android platform in your project.

Jay is a Software Engineer and Writer. He blogs occasionally at Code Handbook and Tech Illumination.