In this tutorial, I’ll demonstrate how to build a fully functioning CRUD app using Node for the backend and htmx for the frontend. This will demonstrate how htmx integrates into a full-stack application, allowing you to assess its effectiveness and decide if it’s a good choice for your future projects.
htmx is a modern JavaScript library designed to enhance web applications by enabling partial HTML updates without the need for full page reloads. It does this by sending HTML over the wire, as opposed to the JSON payload associated with traditional frontend frameworks.
What We’ll Be Building
We’ll develop a simple contact manager capable of all CRUD actions: creating, reading, updating, and deleting contacts. By leveraging htmx, the application will offer a single-page application (SPA) feel, enhancing interactivity and user experience.
If users have JavaScript disabled, the app will work with full-page refreshes, maintaining usability and discoverability. This approach showcases htmx’s ability to create modern web apps while keeping them accessible and SEO-friendly.
Here’s what we’ll end up with.
The code for this article can be found on the accompanying GitHub repo.
Prerequisites
To follow along with this tutorial, you’ll need Node.js installed on your PC. If you don’t have Node installed, please head to the official Node download page and grab the correct binaries for your system. Alternatively, you might like to install Node using a version manager. This approach allows you to install multiple Node versions and switch between them at will.
Apart from that, some familiarity with Node, Pug (which we’ll be using as the template engine) and htmx would be helpful, but not essential. If you’d like a refresher on any of the above, check out our tutorials: Build a Simple Beginner App with Node, A Guide to the Pug HTML Template Preprocessor and An Introduction to htmx.
Before we begin, run the following commands:
node -v
npm -v
You should see output like this:
v20.11.1
10.4.0
This confirms that Node and npm are installed on your machine and are accessible from your command line environment.
Setting Up the Project
Let’s start by scaffolding a new Node project:
mkdir contact-manager
cd contact-manager
npm init -y
This should create a package.json
file in the project root.
Next, let’s install the dependencies we’re going to need:
npm i express method-override pug
Of these packages, Express is the backbone of our app. It’s a fast and minimalist web framework which offers a straightforward way to handle requests and responses, and to route URLs to specific handler functions. Pug will serve as our template engine, whereas we’ll use method-override to employ HTTP verbs like PUT and DELETE in places where the client doesn’t support them.
Next, create an app.js
file in the root directory:
touch app.js
And add the following content:
const express = require('express');
const path = require('path');
const routes = require('./routes/index');
const app = express();
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
app.use(express.static('public'));
app.use('/', routes);
const server = app.listen(3000, () => {
console.log(`Express is running on port ${server.address().port}`);
});
Here, we’re setting up the structure of our Express app. This includes configuring Pug as our view engine for rendering views, defining the directory for our static assets, and hooking up our router.
The application listens on port 3000, with a console log to confirm that Express is running and ready to handle requests on the specified port. This setup forms the base of our application and is ready to be extended with further functionality and routes.
Next, let’s create our routes file:
mkdir routes
touch routes/index.js
Open that file and add the following:
const express = require('express');
const router = express.Router();
// GET /contacts
router.get('/contacts', async (req, res) => {
res.send('It works!');
});
Here, we’re setting up a basic route within our newly created routes directory. This route listens for GET requests at the /contacts
endpoint and responds with a simple confirmation message, indicating that everything is functioning properly.
Next, update the “scripts” section of the package.json
file with the following:
"scripts": {
"dev": "node --watch app.js"
},
This makes use of the new watch mode in Node.js, which will restart our app whenever any changes are detected.
Finally, boot everything up with npm run dev
and head to http://localhost:3000/contacts/ in your browser. You should see a message saying “It works!”.
Exciting times!
Displaying All Contacts
Now let’s add some contacts to display. As we’re focusing on htmx, we’ll use a hard-coded array for simplicity. This will keep things streamlined, allowing us to focus on htmx’s dynamic features without the complexity of database integration.
For those interested in adding a database later on, SQLite and Sequelize are great choices, offering a file-based system that doesn’t require a separate database server.
With that said, please add the following to index.js
before the first route:
const contacts = [
{ id: 1, name: 'John Doe', email: 'john.doe@example.com' },
{ id: 2, name: 'Jane Smith', email: 'jane.smith@example.com' },
{ id: 3, name: 'Emily Johnson', email: 'emily.johnson@example.com' },
{ id: 4, name: 'Aarav Patel', email: 'aarav.patel@example.com' },
{ id: 5, name: 'Liu Wei', email: 'liu.wei@example.com' },
{ id: 6, name: 'Fatima Zahra', email: 'fatima.zahra@example.com' },
{ id: 7, name: 'Carlos Hernández', email: 'carlos.hernandez@example.com' },
{ id: 8, name: 'Olivia Kim', email: 'olivia.kim@example.com' },
{ id: 9, name: 'Kwame Nkrumah', email: 'kwame.nkrumah@example.com' },
{ id: 10, name: 'Chen Yu', email: 'chen.yu@example.com' },
];
Now we need to create a template for our route to display. Create a views
folder containing an index.pug
file:
mkdir views
touch views/index.pug
And add the following:
doctype html
html
head
meta(charset='UTF-8')
title Contact Manager
link(rel='preconnect', href='https://fonts.googleapis.com')
link(rel='preconnect', href='https://fonts.gstatic.com', crossorigin)
link(href='https://fonts.googleapis.com/css2?family=Roboto:wght@300;400&display=swap', rel='stylesheet')
link(rel='stylesheet', href='/styles.css')
body
header
a(href='/contacts')
h1 Contact Manager
section#sidebar
ul.contact-list
each contact in contacts
li #{contact.name}
div.actions
a(href='/contacts/new') New Contact
main#content
p Select a contact
script(src='https://unpkg.com/htmx.org@1.9.10')
In this template, we’re laying out the HTML structure for our application. In the head section, we’re including the Roboto font from Google Fonts and a stylesheet for custom styles.
The body is divided into a header, a sidebar for listing contacts, and a main content area where all of our contact information will go. The content area currently contains a placeholder. At the end of the body we’re also including the latest version of the htmx library from a CDN.
The template expects to receive an array of contacts (in a contacts
variable), which we iterate over in the sidebar and output each contact name in an unordered list using Pug’s interpolation syntax.
Next, let’s create the custom stylesheet:
mkdir public
touch public/styles.css
I don’t intend to list the styles here. Please copy them from the CSS file in the accompanying GitHub repo, or feel free to add some of your own. 🙂
Back in index.js
, let’s update our route to use the template:
// GET /contacts
router.get('/contacts', (req, res) => {
res.render('index', { contacts });
});
Now when you refresh the page you should see something like this.
Displaying a Single Contact
So far, all we’ve done is set up a basic Express app. Let’s change that and finally add htmx to the mix. The next step is to make it so that when a user clicks on a contact in the sidebar, that contact’s information is displayed in the main content area — naturally without a full page reload.
To start with, let’s move the sidebar into its own template:
touch views/sidebar.pug
Add the following to this new file:
ul.contact-list
each contact in contacts
li
a(
href=`/contacts/${contact.id}`,
hx-get=`/contacts/${contact.id}`,
hx-target='#content',
hx-push-url='true'
)= contact.name
div.actions
a(href='/contacts/new') New Contact
Here we have made each contact a link pointing to /contacts/${contact.id}
and added three htmx attributes:
hx-get
. When the user clicks a link, htmx will intercept the click and make a GET request via Ajax to the/contacts/${contact.id}
endpoint.hx-target
. When the request completes, the response will be inserted into the div with an ID ofcontent
. We haven’t specified any kind of swap strategy here, so the contents of the div will be replaced with whatever is returned from the Ajax request. This is the default behavior.hx-push-url
. This will ensure that the value specified inhtx-get
is pushed onto the browser’s history stack, changing the URL.
Update index.pug
to use our template:
section#sidebar
include sidebar.pug
Remember: Pug is white space sensitive, so be sure to use the correct indentation.
Now let’s create a new endpoint in index.js
to return the HTML response that htmx is expecting:
// GET /contacts/1
router.get('/contacts/:id', (req, res) => {
const { id } = req.params;
const contact = contacts.find((c) => c.id === Number(id));
res.send(`
<h2>${contact.name}</h2>
<p><strong>Name:</strong> ${contact.name}</p>
<p><strong>Email:</strong> ${contact.email}</p>
`);
});
If you save this and refresh your browser, you should now be able to view the details of each contact.
HTML over the wire
Let’s take a second to understand what’s going on here. As mentioned at the beginning of the article, htmx delivers HTML over the wire, as opposed to the JSON payload associated with traditional frontend frameworks.
We can see this if we open our browser’s developer tools, switch to the Network tab and click on one of the contacts. Upon receiving a request from the frontend, our Express app generates the HTML needed to display that contact and sends it to the browser, where htmx swaps it into the correct place in the UI.
Dealing with a full page refresh
So things are going pretty well, huh? Thanks to htmx, we just made our page dynamic by specifying a couple of attributes on an anchor tag. Unfortunately, there’s a problem…
If you display a contact, then refresh the page, our lovely UI is gone and all you see is the bare contact details. The same will happen if you load the URL directly in your browser.
The reason for this is obvious if you think about it. When you access a URL such as http://localhost:3000/contacts/1, the Express route for '/contacts/:id'
kicks in and returns the HTML for the contact, as we’ve told it to do. It knows nothing about the rest of our UI.
To combat this, we need to make a couple of changes. On the server, we need to check for an HX-Request header, which indicates that the request came from htmx. If this header exists, then we can send our partial. Otherwise, we need to send the full page.
Change the route handler like so:
// GET /contacts/1
router.get('/contacts/:id', (req, res) => {
const { id } = req.params;
const contact = contacts.find((c) => c.id === Number(id));
if (req.headers['hx-request']) {
res.send(`
<h2>${contact.name}</h2>
<p><strong>Name:</strong> ${contact.name}</p>
<p><strong>Email:</strong> ${contact.email}</p>
`);
} else {
res.render('index', { contacts });
}
});
Now, when you reload the page, the UI doesn’t disappear. It does, however, revert from whichever contact you were viewing to the message “Select a contact”, which isn’t ideal.
To fix this, we can introduce a case
statement to our index.pug
template:
main#content
case action
when 'show'
h2 #{contact.name}
p #[strong Name:] #{contact.name}
p #[strong Email:] #{contact.email}
when 'new'
// Coming soon
when 'edit'
// Coming soon
default
p Select a contact
And finally update the route handler:
if (req.headers['hx-request']) {
// As before
} else {
res.render('index', { action: 'show', contacts, contact });
}
Note that we’re now passing in a contact
variable, which will be used in the event of a full page reload.
And with this, our app should withstand being refreshed or having a contact loaded directly.
A quick refactor
Although this works, you might notice that we have some duplicate content in both our route handler and our main pug template. This isn’t ideal, and things will start to get unwieldy as soon as a contact has anything more than a handful of attributes, or we need to use some logic to decide which attributes to display.
To counteract this, let’s move contact into its own template:
touch views/contact.pug
In the newly created template, add this:
h2 #{contact.name}
p #[strong Name:] #{contact.name}
p #[strong Email:] #{contact.email}
In the main template (index.pug
):
main#content
case action
when 'show'
include contact.pug
And our route handler:
if (req.headers['hx-request']) {
res.render('contact', { contact });
} else {
res.render('index', { action: 'show', contacts, contact });
}
Things should still work as before, but now we’ve removed the duplicated code.
The New Contact Form
The next task to turn our attention to is creating a new contact. This part of the tutorial will guide you through setting up the form and backend logic, using htmx to handle submissions dynamically.
Let’s start by updating our sidebar template. Change:
div.actions
a(href='/contacts/new') New Contact
… to:
div.actions
a(
href='/contacts/new',
hx-get='/contacts/new',
hx-target='#content',
hx-push-url='true'
) New Contact
This uses the same htmx attributes as our links to display a contact: hx-get
will make a GET request via Ajax to the /contacts/new
endpoint, hx-target
specifies where to insert the response, and hx-push-url
will ensure that the URL is changed.
Now let’s create a new template for the form:
touch views/form.pug
And add the following code:
h2 New Contact
form(
action='/contacts',
method='POST',
hx-post='/contacts',
hx-target='#sidebar',
hx-on::after-request='if(event.detail.successful) this.reset()'
)
label(for='name') Name:
input#name(type='text', name='name', required)
label(for='email') Email:
input#email(type='email', name='email', required)
div.actions
button(type='submit') Submit
Here, we’re using the hx-post
attribute to tell htmx to intercept the form submission and make a POST request with the form data to the /contacts
endpoint. The result (an updated list of contacts) will be inserted into the sidebar. We don’t want to change the URL in this case, as the user might want to enter multiple new contacts. We do, however, want to empty the form after a successful submission, which is what the hx-on::after-request
does. The hx-on*
attributes allow you to embed scripts inline to respond to events directly on an element. You can read more about it here.
Next, let’s add a route for the form in index.js
:
// GET /contacts
...
// GET /contacts/new
router.get('/contacts/new', (req, res) => {
if (req.headers['hx-request']) {
res.render('form');
} else {
res.render('index', { action: 'new', contacts, contact: {} });
}
});
// GET /contacts/1
...
Route order is important here. If you have the '/contacts/:id'
route first, then Express will try and find a contact with the ID of new
.
Finally, update our index.pug
template to use the form:
when 'new'
include form.pug
Refresh the page, and at this point you should be able to render the new contact form by clicking on the New Contact link in the sidebar.
Creating a Contact
Now we need to create a route to handle form submission.
First update app.js
to give us access to the form’s data within our route handler.
const express = require('express');
const path = require('path');
const routes = require('./routes/index');
const app = express();
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
+ app.use(express.urlencoded({ extended: true }));
app.use(express.static('public'));
app.use('/', routes);
const server = app.listen(3000, () => {
console.log(`Express is running on port ${server.address().port}`);
});
Previously, we would have used the body-parser package, but I recently learned this is no longer necessary.
Then add the following to index.js
:
// POST /contacts
router.post('/contacts', (req, res) => {
const newContact = {
id: contacts.length + 1,
name: req.body.name,
email: req.body.email,
};
contacts.push(newContact);
if (req.headers['hx-request']) {
res.render('sidebar', { contacts });
} else {
res.render('index', { action: 'new', contacts, contact: {} });
}
});
Here, we’re creating a new contact with the data we received from the client and adding it to the contacts
array. We’re then re-rendering the sidebar, passing it the updated list of contacts.
Note that, if you’re making any kind of application that has users, it’s up to you to validate the data you’re receiving from the client. In our example, I’ve added some basic client-side validation, but this can easily be bypassed.
There’s an example of how to validate input on the server using the express-validator package package in the Node tutorial I linked to above.
Now, if you refresh your browser and try adding a contact, it should work as expected: the new contact should be added to the sidebar and the form should be reset.
Adding flash messages
This is well and good, but now we need a way to inform the user that a contact has been added. In a typical application, we would use a flash message — a temporary notification that alerts the user about the outcome of an action.
The problem we encounter with htmx is that we’re updating the sidebar after successfully creating a new contact, but this isn’t where we want our flash message to be displayed. A better location would be above the new contact form.
To get around this, we can use the hx-swap-oob
attribute. This allows you to specify that some content in a response should be swapped into the DOM somewhere other than the target, that is “Out of Band”.
Update the route handler as follows:
if (req.headers['hx-request']) {
res.render('sidebar', { contacts }, (err, sidebarHtml) => {
const html = `
<main id="content" hx-swap-oob="afterbegin">
<p class="flash">Contact was successfully added!</p>
</main>
${sidebarHtml}
`;
res.send(html);
});
} else {
res.render('index', { action: 'new', contacts, contact: {} });
}
Here, we’re rendering the sidebar as before, but passing the render
method an anonymous function as the third parameter. This function receives the HTML generated by calling res.render('sidebar', { contacts })
, which we can then use to assemble our final response.
By specifying a swap strategy of "afterbegin"
, the flash message is inserted at the top of the container.
Now, when we add a contact, we should get a nice message informing us what happened.
Editing a Contact
For updating a contact, we’re going to reuse the form we created in the previous section.
Let’s start by updating our contact.pug
template to add the following:
div.actions
a(
href=`/contacts/${contact.id}/edit`,
hx-get=`/contacts/${contact.id}/edit`,
hx-target='#content',
hx-push-url='true'
) Edit Contact
This will add an Edit Contact button beneath a contacts details. As we’ve seen before, when the link is clicked, hx-get
will make a GET request via Ajax to the /${contact.id}/edit
endpoint, hx-target
will specify where to insert the response, and hx-push-url
will ensure that the URL is changed.
Now let’s alter our index.pug
template to use the form:
when 'edit'
include form.pug
Also add a route handler to display the form:
// GET /contacts/1/edit
router.get('/contacts/:id/edit', (req, res) => {
const { id } = req.params;
const contact = contacts.find((c) => c.id === Number(id));
if (req.headers['hx-request']) {
res.render('form', { contact });
} else {
res.render('index', { action: 'edit', contacts, contact });
}
});
Note that we’re retrieving the contact using the ID from the request, then passing that contact to the form.
We’ll also need to update our new contact handler to do the same, but here passing an empty object:
// GET /contacts/new
router.get('/contacts/new', (req, res) => {
if (req.headers['hx-request']) {
- res.render('form');
+ res.render('form', { contact: {} });
} else {
res.render('index', { action: 'new', contacts, contact: {} });
}
});
Then we need to update the form itself:
- isEditing = () => !(Object.keys(contact).length === 0);
h2=isEditing() ? "Edit Contact" : "New Contact"
form(
action=isEditing() ? `/update/${contact.id}?_method=PUT` : '/contacts',
method='POST',
hx-post=isEditing() ? false : '/contacts',
hx-put=isEditing() ? `/update/${contact.id}` : false,
hx-target='#sidebar',
hx-push-url=isEditing() ? `/contacts/${contact.id}` : false
hx-on::after-request='if(event.detail.successful) this.reset()',
)
label(for='name') Name:
input#name(type='text', name='name', required, value=contact.name)
label(for='email') Email:
input#email(type='email', name='email', required, value=contact.email)
div.actions
button(type='submit') Submit
As we’re passing in either a contact or an empty object to this form, we now have an easy way to determine if we’re in “edit” or “create” mode. We can do this by checking Object.keys(contact).length
. We can also extract this check into a little helper function at the top of the file using Pug’s unbuffered code syntax.
Once we know which mode we find ourselves in, we can conditionally change the page title, then decide which attributes we add to the form tag. For the edit form, we need to add a hx-put
attribute and set it to /update/${contact.id}
. We also need to update the URL once the contact’s details have been saved.
To do all of this, we can utilize the fact that, if a conditional returns false
, Pug will omit the attribute from the tag.
Meaning that this:
form(
action=isEditing() ? `/update/${contact.id}?_method=PUT` : '/contacts',
method='POST',
hx-post=isEditing() ? false : '/contacts',
hx-put=isEditing() ? `/update/${contact.id}` : false,
hx-target='#sidebar',
hx-on::after-request='if(event.detail.successful) this.reset()',
hx-push-url=isEditing() ? `/contacts/${contact.id}` : false
)
… will compile to the following when isEditing()
returns false
:
<form
action="/contacts"
method="POST"
hx-post="/contacts"
hx-target="#sidebar"
hx-on::after-request="if(event.detail.successful) this.reset()"
>
...
</form>
But when isEditing()
returns true
, it will compile to:
<form
action="/update/1?_method=PUT"
method="POST"
hx-put="/update/1"
hx-target="#sidebar"
hx-on::after-request="if(event.detail.successful) this.reset()"
hx-push-url="/contacts/1"
>
...
</form>
In its update state, notice that the form action is "/update/1?_method=PUT"
. This query string parameter has been added because we’re using the method-override package, and it will make our router respond to a PUT request.
Out of the box, htmx can send PUT and DELETE requests, but the browser can’t. This means that, if we want to deal with a scenario where JavaScript is disabled, we would need to duplicate our route handler, having it respond to both PUT (htmx) and POST (the browser). Using this middleware will keep our code DRY.
Let’s go ahead and add it to app.js
:
const express = require('express');
const path = require('path');
+ const methodOverride = require('method-override');
const routes = require('./routes/index');
const app = express();
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
+ app.use(methodOverride('_method'));
app.use(express.urlencoded({ extended: true }));
app.use(express.static('public'));
app.use('/', routes);
const server = app.listen(3000, () => {
console.log(`Express is running on port ${server.address().port}`);
});
Finally, let’s update index.js
with a new route handler:
// PUT /contacts/1
router.put('/update/:id', (req, res) => {
const { id } = req.params;
const newContact = {
id: Number(id),
name: req.body.name,
email: req.body.email,
};
const index = contacts.findIndex((c) => c.id === Number(id));
if (index !== -1) contacts[index] = newContact;
if (req.headers['hx-request']) {
res.render('sidebar', { contacts }, (err, sidebarHtml) => {
res.render('contact', { contact: contacts[index] }, (err, contactHTML) => {
const html = `
${sidebarHtml}
<main id="content" hx-swap-oob="true">
<p class="flash">Contact was successfully updated!</p>
${contactHTML}
</main>
`;
res.send(html);
});
});
} else {
res.redirect(`/contacts/${index + 1}`);
}
});
Hopefully there’s nothing too mysterious here by now. At the beginning of the handler we grab the contact ID from the request params. We then find the contact we wish to update and swap it out with a new contact created from the form data we received.
When dealing with an htmx request, we first render the sidebar template with our updated contacts list. We then render the contact template with the updated contact and use the result of both of these calls to assemble our response. As before, we use an “Out of Band” update to create a flash message informing the user that the contact was updated.
At this point, you should be able to update contacts.
Deleting a Contact
The final piece of the puzzle is the ability to delete contacts. Let’s add a button to do this to our contact template:
div.actions
form(method='POST', action=`/delete/${contact.id}?_method=DELETE`)
button(
type='submit',
hx-delete=`/delete/${contact.id}`,
hx-target='#sidebar',
hx-push-url='/contacts'
class='link'
) Delete Contact
a(
// as before
)
Note that it’s good practice to use a form and a button to issue the DELETE request. Forms are designed for actions that cause changes, like deletions, and this ensures semantic correctness. Additionally, using a link for a delete action could be risky because search engines can inadvertently follow links, potentially leading to unwanted deletions.
That being said, I’ve added some CSS to style the button like a link, as buttons are ugly. If you copied the styles from the repo before, you already have this in your code.
And finally, our route handler in index.js
:
// DELETE /contacts/1
router.delete('/delete/:id', (req, res) => {
const { id } = req.params;
const index = contacts.findIndex((c) => c.id === Number(id));
if (index !== -1) contacts.splice(index, 1);
if (req.headers['hx-request']) {
res.render('sidebar', { contacts }, (err, sidebarHtml) => {
const html = `
<main id="content" hx-swap-oob="true">
<p class="flash">Contact was successfully deleted!</p>
</main>
${sidebarHtml}
`;
res.send(html);
});
} else {
res.redirect('/contacts');
}
});
Once the contact has been removed, we’re updating the sidebar and showing the user a flash message.
Taking It Further
And that’s a wrap.
In this article, we’ve crafted a full-stack CRUD application using Node and Express for the backend and htmx for the frontend. Along the way, I’ve demonstrated how htmx can simplify adding dynamic behavior to your web apps, reducing the need for complex JavaScript and full-page reloads, and thus making the user experience smoother and more interactive.
And as an added bonus, the app also functions well without JavaScript.
Yet while our app is fully functional, it’s admittedly a little bare-bones. If you wish to continue exploring htmx, you might like to look at implementing view transitions between app states, or adding some further validation to the form — for example, to verify that the email address comes from a specific domain.
I have examples of both of these things (and more besides) in my Introduction to htmx.
Apart from that, if you have any questions or comments, please reach out on X.
Happy coding!
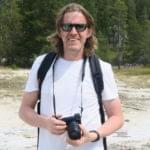
Network admin, freelance web developer and editor at SitePoint.