Keep up to date on current trends and technologies
Ruby - Switching to Ruby
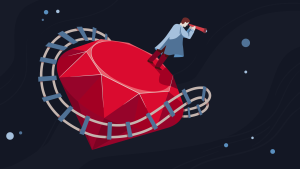
Ruby, Rails, and Imposter Syndrome, with Glenn Goodrich
M. David GreenTim Evko
PHP to Sinatra
Andy Hawthorne
Albacore: Building .NET Applications with Rake
Ian Oxley
Properties and Methods in Ruby from a .NET POV
Claudio Lassala
Class Variables – A Ruby Gotcha
Dave Kennedy
Putting Ruby, Rails, C#, and ASP.NET in context
Claudio Lassala
Showing 6 of 6